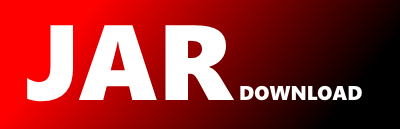
org.hyperledger.fabric.protos.peer.TxValidationCode Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: peer/transaction.proto
// Protobuf Java Version: 4.28.2
package org.hyperledger.fabric.protos.peer;
/**
* Protobuf enum {@code protos.TxValidationCode}
*/
public enum TxValidationCode
implements com.google.protobuf.ProtocolMessageEnum {
/**
* VALID = 0;
*/
VALID(0),
/**
* NIL_ENVELOPE = 1;
*/
NIL_ENVELOPE(1),
/**
* BAD_PAYLOAD = 2;
*/
BAD_PAYLOAD(2),
/**
* BAD_COMMON_HEADER = 3;
*/
BAD_COMMON_HEADER(3),
/**
* BAD_CREATOR_SIGNATURE = 4;
*/
BAD_CREATOR_SIGNATURE(4),
/**
* INVALID_ENDORSER_TRANSACTION = 5;
*/
INVALID_ENDORSER_TRANSACTION(5),
/**
* INVALID_CONFIG_TRANSACTION = 6;
*/
INVALID_CONFIG_TRANSACTION(6),
/**
* UNSUPPORTED_TX_PAYLOAD = 7;
*/
UNSUPPORTED_TX_PAYLOAD(7),
/**
* BAD_PROPOSAL_TXID = 8;
*/
BAD_PROPOSAL_TXID(8),
/**
* DUPLICATE_TXID = 9;
*/
DUPLICATE_TXID(9),
/**
* ENDORSEMENT_POLICY_FAILURE = 10;
*/
ENDORSEMENT_POLICY_FAILURE(10),
/**
* MVCC_READ_CONFLICT = 11;
*/
MVCC_READ_CONFLICT(11),
/**
* PHANTOM_READ_CONFLICT = 12;
*/
PHANTOM_READ_CONFLICT(12),
/**
* UNKNOWN_TX_TYPE = 13;
*/
UNKNOWN_TX_TYPE(13),
/**
* TARGET_CHAIN_NOT_FOUND = 14;
*/
TARGET_CHAIN_NOT_FOUND(14),
/**
* MARSHAL_TX_ERROR = 15;
*/
MARSHAL_TX_ERROR(15),
/**
* NIL_TXACTION = 16;
*/
NIL_TXACTION(16),
/**
* EXPIRED_CHAINCODE = 17;
*/
EXPIRED_CHAINCODE(17),
/**
* CHAINCODE_VERSION_CONFLICT = 18;
*/
CHAINCODE_VERSION_CONFLICT(18),
/**
* BAD_HEADER_EXTENSION = 19;
*/
BAD_HEADER_EXTENSION(19),
/**
* BAD_CHANNEL_HEADER = 20;
*/
BAD_CHANNEL_HEADER(20),
/**
* BAD_RESPONSE_PAYLOAD = 21;
*/
BAD_RESPONSE_PAYLOAD(21),
/**
* BAD_RWSET = 22;
*/
BAD_RWSET(22),
/**
* ILLEGAL_WRITESET = 23;
*/
ILLEGAL_WRITESET(23),
/**
* INVALID_WRITESET = 24;
*/
INVALID_WRITESET(24),
/**
* INVALID_CHAINCODE = 25;
*/
INVALID_CHAINCODE(25),
/**
* NOT_VALIDATED = 254;
*/
NOT_VALIDATED(254),
/**
* INVALID_OTHER_REASON = 255;
*/
INVALID_OTHER_REASON(255),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 2,
/* suffix= */ "",
TxValidationCode.class.getName());
}
/**
* VALID = 0;
*/
public static final int VALID_VALUE = 0;
/**
* NIL_ENVELOPE = 1;
*/
public static final int NIL_ENVELOPE_VALUE = 1;
/**
* BAD_PAYLOAD = 2;
*/
public static final int BAD_PAYLOAD_VALUE = 2;
/**
* BAD_COMMON_HEADER = 3;
*/
public static final int BAD_COMMON_HEADER_VALUE = 3;
/**
* BAD_CREATOR_SIGNATURE = 4;
*/
public static final int BAD_CREATOR_SIGNATURE_VALUE = 4;
/**
* INVALID_ENDORSER_TRANSACTION = 5;
*/
public static final int INVALID_ENDORSER_TRANSACTION_VALUE = 5;
/**
* INVALID_CONFIG_TRANSACTION = 6;
*/
public static final int INVALID_CONFIG_TRANSACTION_VALUE = 6;
/**
* UNSUPPORTED_TX_PAYLOAD = 7;
*/
public static final int UNSUPPORTED_TX_PAYLOAD_VALUE = 7;
/**
* BAD_PROPOSAL_TXID = 8;
*/
public static final int BAD_PROPOSAL_TXID_VALUE = 8;
/**
* DUPLICATE_TXID = 9;
*/
public static final int DUPLICATE_TXID_VALUE = 9;
/**
* ENDORSEMENT_POLICY_FAILURE = 10;
*/
public static final int ENDORSEMENT_POLICY_FAILURE_VALUE = 10;
/**
* MVCC_READ_CONFLICT = 11;
*/
public static final int MVCC_READ_CONFLICT_VALUE = 11;
/**
* PHANTOM_READ_CONFLICT = 12;
*/
public static final int PHANTOM_READ_CONFLICT_VALUE = 12;
/**
* UNKNOWN_TX_TYPE = 13;
*/
public static final int UNKNOWN_TX_TYPE_VALUE = 13;
/**
* TARGET_CHAIN_NOT_FOUND = 14;
*/
public static final int TARGET_CHAIN_NOT_FOUND_VALUE = 14;
/**
* MARSHAL_TX_ERROR = 15;
*/
public static final int MARSHAL_TX_ERROR_VALUE = 15;
/**
* NIL_TXACTION = 16;
*/
public static final int NIL_TXACTION_VALUE = 16;
/**
* EXPIRED_CHAINCODE = 17;
*/
public static final int EXPIRED_CHAINCODE_VALUE = 17;
/**
* CHAINCODE_VERSION_CONFLICT = 18;
*/
public static final int CHAINCODE_VERSION_CONFLICT_VALUE = 18;
/**
* BAD_HEADER_EXTENSION = 19;
*/
public static final int BAD_HEADER_EXTENSION_VALUE = 19;
/**
* BAD_CHANNEL_HEADER = 20;
*/
public static final int BAD_CHANNEL_HEADER_VALUE = 20;
/**
* BAD_RESPONSE_PAYLOAD = 21;
*/
public static final int BAD_RESPONSE_PAYLOAD_VALUE = 21;
/**
* BAD_RWSET = 22;
*/
public static final int BAD_RWSET_VALUE = 22;
/**
* ILLEGAL_WRITESET = 23;
*/
public static final int ILLEGAL_WRITESET_VALUE = 23;
/**
* INVALID_WRITESET = 24;
*/
public static final int INVALID_WRITESET_VALUE = 24;
/**
* INVALID_CHAINCODE = 25;
*/
public static final int INVALID_CHAINCODE_VALUE = 25;
/**
* NOT_VALIDATED = 254;
*/
public static final int NOT_VALIDATED_VALUE = 254;
/**
* INVALID_OTHER_REASON = 255;
*/
public static final int INVALID_OTHER_REASON_VALUE = 255;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TxValidationCode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static TxValidationCode forNumber(int value) {
switch (value) {
case 0: return VALID;
case 1: return NIL_ENVELOPE;
case 2: return BAD_PAYLOAD;
case 3: return BAD_COMMON_HEADER;
case 4: return BAD_CREATOR_SIGNATURE;
case 5: return INVALID_ENDORSER_TRANSACTION;
case 6: return INVALID_CONFIG_TRANSACTION;
case 7: return UNSUPPORTED_TX_PAYLOAD;
case 8: return BAD_PROPOSAL_TXID;
case 9: return DUPLICATE_TXID;
case 10: return ENDORSEMENT_POLICY_FAILURE;
case 11: return MVCC_READ_CONFLICT;
case 12: return PHANTOM_READ_CONFLICT;
case 13: return UNKNOWN_TX_TYPE;
case 14: return TARGET_CHAIN_NOT_FOUND;
case 15: return MARSHAL_TX_ERROR;
case 16: return NIL_TXACTION;
case 17: return EXPIRED_CHAINCODE;
case 18: return CHAINCODE_VERSION_CONFLICT;
case 19: return BAD_HEADER_EXTENSION;
case 20: return BAD_CHANNEL_HEADER;
case 21: return BAD_RESPONSE_PAYLOAD;
case 22: return BAD_RWSET;
case 23: return ILLEGAL_WRITESET;
case 24: return INVALID_WRITESET;
case 25: return INVALID_CHAINCODE;
case 254: return NOT_VALIDATED;
case 255: return INVALID_OTHER_REASON;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TxValidationCode> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TxValidationCode findValueByNumber(int number) {
return TxValidationCode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.hyperledger.fabric.protos.peer.TransactionProto.getDescriptor().getEnumTypes().get(0);
}
private static final TxValidationCode[] VALUES = values();
public static TxValidationCode valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private TxValidationCode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:protos.TxValidationCode)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy