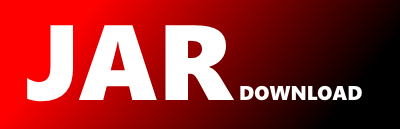
org.iata.ndc.schema.LeadPrice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ndc-jaxb Show documentation
Show all versions of ndc-jaxb Show documentation
JAXB generated classes for NDC Client
The newest version!
package org.iata.ndc.schema;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlElementRefs;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="LeadDate" type="{http://www.w3.org/2001/XMLSchema}date"/>
* <element name="RequestedDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* <choice maxOccurs="2">
* <element ref="{http://www.iata.org/IATA/EDIST}OriginDestinationReference"/>
* <element ref="{http://www.iata.org/IATA/EDIST}SegmentReference"/>
* </choice>
* <choice>
* <element name="TotalAmount">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice>
* <element ref="{http://www.iata.org/IATA/EDIST}AwardPricing"/>
* <element ref="{http://www.iata.org/IATA/EDIST}CombinationPricing"/>
* <element ref="{http://www.iata.org/IATA/EDIST}SimpleCurrencyPrice"/>
* <element ref="{http://www.iata.org/IATA/EDIST}DetailCurrencyPrice"/>
* <element ref="{http://www.iata.org/IATA/EDIST}EncodedCurrencyPrice"/>
* </choice>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <sequence>
* <element name="BaseAmount" type="{http://www.iata.org/IATA/EDIST}CurrencyAmountOptType"/>
* <element ref="{http://www.iata.org/IATA/EDIST}FareFiledIn" minOccurs="0"/>
* <element name="Surcharges" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Surcharge" type="{http://www.iata.org/IATA/EDIST}FeeSurchargeType" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="Taxes" type="{http://www.iata.org/IATA/EDIST}TaxDetailType" minOccurs="0"/>
* <element ref="{http://www.iata.org/IATA/EDIST}TaxExemption" minOccurs="0"/>
* <choice>
* <element name="AwardPricing" type="{http://www.iata.org/IATA/EDIST}AwardPriceUnitType" minOccurs="0"/>
* <element name="CombinationPricing" type="{http://www.iata.org/IATA/EDIST}CombinationPriceType" minOccurs="0"/>
* </choice>
* </sequence>
* </choice>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"leadDate",
"requestedDate",
"originDestinationReferenceOrSegmentReference",
"totalAmount",
"baseAmount",
"fareFiledIn",
"surcharges",
"taxes",
"taxExemption",
"awardPricing",
"combinationPricing"
})
@XmlRootElement(name = "LeadPrice")
public class LeadPrice {
@XmlElement(name = "LeadDate", required = true)
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar leadDate;
@XmlElement(name = "RequestedDate")
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar requestedDate;
@XmlElementRefs({
@XmlElementRef(name = "SegmentReference", namespace = "http://www.iata.org/IATA/EDIST", type = SegmentReference.class),
@XmlElementRef(name = "OriginDestinationReference", namespace = "http://www.iata.org/IATA/EDIST", type = JAXBElement.class)
})
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy