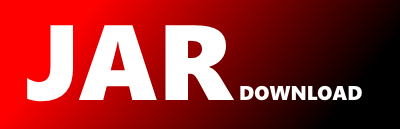
org.iata.ndc.schema.OrderListRS Maven / Gradle / Ivy
Show all versions of ndc-jaxb Show documentation
package org.iata.ndc.schema;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlIDREF;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlValue;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.iata.org/IATA/EDIST}Document"/>
* <choice>
* <sequence>
* <element name="Success" type="{http://www.iata.org/IATA/EDIST}SuccessType"/>
* <element name="Warnings" type="{http://www.iata.org/IATA/EDIST}WarningsType" minOccurs="0"/>
* <element name="Response">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.iata.org/IATA/EDIST}OrderListProcessing"/>
* <element name="Orders" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Order" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Agency" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.iata.org/IATA/EDIST}AgencyID" minOccurs="0"/>
* <element name="Name" type="{http://www.iata.org/IATA/EDIST}ProperNameSimpleType" minOccurs="0"/>
* <element name="IATA_Number" type="{http://www.iata.org/IATA/EDIST}IATA_NbrSimpleType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="CreationDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* <element name="Departure" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AirportCode">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>AirportCitySimpleType">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}RadiusAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="Date" type="{http://www.w3.org/2001/XMLSchema}date"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="NbrInParty" minOccurs="0">
* <complexType>
* <simpleContent>
* <extension base="<http://www.w3.org/2001/XMLSchema>positiveInteger">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}ObjAssociationAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="Passengers" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice>
* <element name="Group" type="{http://www.iata.org/IATA/EDIST}GroupType"/>
* <element name="FullName" maxOccurs="unbounded">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>ProperNameSimpleType">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}ObjAssociationAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* </choice>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="TicketingStatus" minOccurs="0">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>TicketDesigAppListType">
* <attribute name="Context" type="{http://www.iata.org/IATA/EDIST}ContextSimpleType" />
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="OrderID" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* <element ref="{http://www.iata.org/IATA/EDIST}Errors"/>
* </choice>
* </sequence>
* <attGroup ref="{http://www.iata.org/IATA/EDIST}IATA_PayloadStdAttributes"/>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"document",
"success",
"warnings",
"response",
"errors"
})
@XmlRootElement(name = "OrderListRS")
public class OrderListRS {
@XmlElement(name = "Document", required = true)
protected MsgDocumentType document;
@XmlElement(name = "Success")
protected SuccessType success;
@XmlElementWrapper(name = "Warnings")
@XmlElement(name = "Warning", namespace = "http://www.iata.org/IATA/EDIST")
protected List warnings;
@XmlElement(name = "Response")
protected OrderListRS.Response response;
@XmlElementWrapper(name = "Errors")
@XmlElement(name = "Error", namespace = "http://www.iata.org/IATA/EDIST")
protected List errors;
@XmlAttribute(name = "EchoToken")
protected String echoToken;
@XmlAttribute(name = "TimeStamp")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar timeStamp;
@XmlAttribute(name = "Target")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String target;
@XmlAttribute(name = "Version", required = true)
protected String version;
@XmlAttribute(name = "TransactionIdentifier")
protected String transactionIdentifier;
@XmlAttribute(name = "SequenceNmbr")
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger sequenceNmbr;
@XmlAttribute(name = "TransactionStatusCode")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String transactionStatusCode;
@XmlAttribute(name = "RetransmissionIndicator")
protected Boolean retransmissionIndicator;
@XmlAttribute(name = "CorrelationID")
protected String correlationID;
@XmlAttribute(name = "AsynchronousAllowedInd")
protected Boolean asynchronousAllowedInd;
@XmlAttribute(name = "PrimaryLangID")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "language")
protected String primaryLangID;
@XmlAttribute(name = "AltLangID")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "language")
protected String altLangID;
/**
* Gets the value of the document property.
*
* @return
* possible object is
* {@link MsgDocumentType }
*
*/
public MsgDocumentType getDocument() {
return document;
}
/**
* Sets the value of the document property.
*
* @param value
* allowed object is
* {@link MsgDocumentType }
*
*/
public void setDocument(MsgDocumentType value) {
this.document = value;
}
/**
* Gets the value of the success property.
*
* @return
* possible object is
* {@link SuccessType }
*
*/
public SuccessType getSuccess() {
return success;
}
/**
* Sets the value of the success property.
*
* @param value
* allowed object is
* {@link SuccessType }
*
*/
public void setSuccess(SuccessType value) {
this.success = value;
}
/**
* Gets the value of the response property.
*
* @return
* possible object is
* {@link OrderListRS.Response }
*
*/
public OrderListRS.Response getResponse() {
return response;
}
/**
* Sets the value of the response property.
*
* @param value
* allowed object is
* {@link OrderListRS.Response }
*
*/
public void setResponse(OrderListRS.Response value) {
this.response = value;
}
/**
* Gets the value of the echoToken property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEchoToken() {
return echoToken;
}
/**
* Sets the value of the echoToken property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEchoToken(String value) {
this.echoToken = value;
}
/**
* Gets the value of the timeStamp property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTimeStamp() {
return timeStamp;
}
/**
* Sets the value of the timeStamp property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTimeStamp(XMLGregorianCalendar value) {
this.timeStamp = value;
}
/**
* Gets the value of the target property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTarget() {
if (target == null) {
return "Production";
} else {
return target;
}
}
/**
* Sets the value of the target property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTarget(String value) {
this.target = value;
}
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
/**
* Gets the value of the transactionIdentifier property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionIdentifier() {
return transactionIdentifier;
}
/**
* Sets the value of the transactionIdentifier property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionIdentifier(String value) {
this.transactionIdentifier = value;
}
/**
* Gets the value of the sequenceNmbr property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getSequenceNmbr() {
return sequenceNmbr;
}
/**
* Sets the value of the sequenceNmbr property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setSequenceNmbr(BigInteger value) {
this.sequenceNmbr = value;
}
/**
* Gets the value of the transactionStatusCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionStatusCode() {
return transactionStatusCode;
}
/**
* Sets the value of the transactionStatusCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionStatusCode(String value) {
this.transactionStatusCode = value;
}
/**
* Gets the value of the retransmissionIndicator property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRetransmissionIndicator() {
return retransmissionIndicator;
}
/**
* Sets the value of the retransmissionIndicator property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRetransmissionIndicator(Boolean value) {
this.retransmissionIndicator = value;
}
/**
* Gets the value of the correlationID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCorrelationID() {
return correlationID;
}
/**
* Sets the value of the correlationID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCorrelationID(String value) {
this.correlationID = value;
}
/**
* Gets the value of the asynchronousAllowedInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAsynchronousAllowedInd() {
return asynchronousAllowedInd;
}
/**
* Sets the value of the asynchronousAllowedInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setAsynchronousAllowedInd(Boolean value) {
this.asynchronousAllowedInd = value;
}
/**
* Gets the value of the primaryLangID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrimaryLangID() {
return primaryLangID;
}
/**
* Sets the value of the primaryLangID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrimaryLangID(String value) {
this.primaryLangID = value;
}
/**
* Gets the value of the altLangID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAltLangID() {
return altLangID;
}
/**
* Sets the value of the altLangID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAltLangID(String value) {
this.altLangID = value;
}
public List getWarnings() {
if (warnings == null) {
warnings = new ArrayList();
}
return warnings;
}
public void setWarnings(List warnings) {
this.warnings = warnings;
}
public List getErrors() {
if (errors == null) {
errors = new ArrayList();
}
return errors;
}
public void setErrors(List errors) {
this.errors = errors;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.iata.org/IATA/EDIST}OrderListProcessing"/>
* <element name="Orders" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Order" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Agency" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.iata.org/IATA/EDIST}AgencyID" minOccurs="0"/>
* <element name="Name" type="{http://www.iata.org/IATA/EDIST}ProperNameSimpleType" minOccurs="0"/>
* <element name="IATA_Number" type="{http://www.iata.org/IATA/EDIST}IATA_NbrSimpleType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="CreationDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* <element name="Departure" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AirportCode">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>AirportCitySimpleType">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}RadiusAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="Date" type="{http://www.w3.org/2001/XMLSchema}date"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="NbrInParty" minOccurs="0">
* <complexType>
* <simpleContent>
* <extension base="<http://www.w3.org/2001/XMLSchema>positiveInteger">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}ObjAssociationAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="Passengers" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice>
* <element name="Group" type="{http://www.iata.org/IATA/EDIST}GroupType"/>
* <element name="FullName" maxOccurs="unbounded">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>ProperNameSimpleType">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}ObjAssociationAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* </choice>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="TicketingStatus" minOccurs="0">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>TicketDesigAppListType">
* <attribute name="Context" type="{http://www.iata.org/IATA/EDIST}ContextSimpleType" />
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="OrderID" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"orderListProcessing",
"orders"
})
public static class Response {
@XmlElement(name = "OrderListProcessing", required = true)
protected OrderListProcessType orderListProcessing;
@XmlElementWrapper(name = "Orders")
@XmlElement(name = "Order", namespace = "http://www.iata.org/IATA/EDIST")
protected List orders;
/**
* Transaction Processing Results, including processing status, alerts, notices, business warnings, supplemental marketing messages and policy.
*
* @return
* possible object is
* {@link OrderListProcessType }
*
*/
public OrderListProcessType getOrderListProcessing() {
return orderListProcessing;
}
/**
* Sets the value of the orderListProcessing property.
*
* @param value
* allowed object is
* {@link OrderListProcessType }
*
*/
public void setOrderListProcessing(OrderListProcessType value) {
this.orderListProcessing = value;
}
public List getOrders() {
if (orders == null) {
orders = new ArrayList();
}
return orders;
}
public void setOrders(List orders) {
this.orders = orders;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="Agency" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.iata.org/IATA/EDIST}AgencyID" minOccurs="0"/>
* <element name="Name" type="{http://www.iata.org/IATA/EDIST}ProperNameSimpleType" minOccurs="0"/>
* <element name="IATA_Number" type="{http://www.iata.org/IATA/EDIST}IATA_NbrSimpleType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="CreationDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* <element name="Departure" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AirportCode">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>AirportCitySimpleType">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}RadiusAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="Date" type="{http://www.w3.org/2001/XMLSchema}date"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="NbrInParty" minOccurs="0">
* <complexType>
* <simpleContent>
* <extension base="<http://www.w3.org/2001/XMLSchema>positiveInteger">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}ObjAssociationAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="Passengers" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice>
* <element name="Group" type="{http://www.iata.org/IATA/EDIST}GroupType"/>
* <element name="FullName" maxOccurs="unbounded">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>ProperNameSimpleType">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}ObjAssociationAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* </choice>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="TicketingStatus" minOccurs="0">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>TicketDesigAppListType">
* <attribute name="Context" type="{http://www.iata.org/IATA/EDIST}ContextSimpleType" />
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="OrderID" type="{http://www.w3.org/2001/XMLSchema}anyType"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"agency",
"creationDate",
"departure",
"nbrInParty",
"passengers",
"ticketingStatus",
"orderID"
})
public static class Order {
@XmlElement(name = "Agency")
protected OrderListRS.Response.Order.Agency agency;
@XmlElement(name = "CreationDate")
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar creationDate;
@XmlElement(name = "Departure")
protected OrderListRS.Response.Order.Departure departure;
@XmlElement(name = "NbrInParty")
protected OrderListRS.Response.Order.NbrInParty nbrInParty;
@XmlElement(name = "Passengers")
protected OrderListRS.Response.Order.Passengers passengers;
@XmlElement(name = "TicketingStatus")
protected OrderListRS.Response.Order.TicketingStatus ticketingStatus;
@XmlElement(name = "OrderID", required = true)
protected Object orderID;
/**
* Gets the value of the agency property.
*
* @return
* possible object is
* {@link OrderListRS.Response.Order.Agency }
*
*/
public OrderListRS.Response.Order.Agency getAgency() {
return agency;
}
/**
* Sets the value of the agency property.
*
* @param value
* allowed object is
* {@link OrderListRS.Response.Order.Agency }
*
*/
public void setAgency(OrderListRS.Response.Order.Agency value) {
this.agency = value;
}
/**
* Gets the value of the creationDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getCreationDate() {
return creationDate;
}
/**
* Sets the value of the creationDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setCreationDate(XMLGregorianCalendar value) {
this.creationDate = value;
}
/**
* Gets the value of the departure property.
*
* @return
* possible object is
* {@link OrderListRS.Response.Order.Departure }
*
*/
public OrderListRS.Response.Order.Departure getDeparture() {
return departure;
}
/**
* Sets the value of the departure property.
*
* @param value
* allowed object is
* {@link OrderListRS.Response.Order.Departure }
*
*/
public void setDeparture(OrderListRS.Response.Order.Departure value) {
this.departure = value;
}
/**
* Gets the value of the nbrInParty property.
*
* @return
* possible object is
* {@link OrderListRS.Response.Order.NbrInParty }
*
*/
public OrderListRS.Response.Order.NbrInParty getNbrInParty() {
return nbrInParty;
}
/**
* Sets the value of the nbrInParty property.
*
* @param value
* allowed object is
* {@link OrderListRS.Response.Order.NbrInParty }
*
*/
public void setNbrInParty(OrderListRS.Response.Order.NbrInParty value) {
this.nbrInParty = value;
}
/**
* Gets the value of the passengers property.
*
* @return
* possible object is
* {@link OrderListRS.Response.Order.Passengers }
*
*/
public OrderListRS.Response.Order.Passengers getPassengers() {
return passengers;
}
/**
* Sets the value of the passengers property.
*
* @param value
* allowed object is
* {@link OrderListRS.Response.Order.Passengers }
*
*/
public void setPassengers(OrderListRS.Response.Order.Passengers value) {
this.passengers = value;
}
/**
* Gets the value of the ticketingStatus property.
*
* @return
* possible object is
* {@link OrderListRS.Response.Order.TicketingStatus }
*
*/
public OrderListRS.Response.Order.TicketingStatus getTicketingStatus() {
return ticketingStatus;
}
/**
* Sets the value of the ticketingStatus property.
*
* @param value
* allowed object is
* {@link OrderListRS.Response.Order.TicketingStatus }
*
*/
public void setTicketingStatus(OrderListRS.Response.Order.TicketingStatus value) {
this.ticketingStatus = value;
}
/**
* Gets the value of the orderID property.
*
* @return
* possible object is
* {@link Object }
*
*/
public Object getOrderID() {
return orderID;
}
/**
* Sets the value of the orderID property.
*
* @param value
* allowed object is
* {@link Object }
*
*/
public void setOrderID(Object value) {
this.orderID = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.iata.org/IATA/EDIST}AgencyID" minOccurs="0"/>
* <element name="Name" type="{http://www.iata.org/IATA/EDIST}ProperNameSimpleType" minOccurs="0"/>
* <element name="IATA_Number" type="{http://www.iata.org/IATA/EDIST}IATA_NbrSimpleType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"agencyID",
"name",
"iataNumber"
})
public static class Agency {
@XmlElement(name = "AgencyID")
protected AgencyIDType agencyID;
@XmlElement(name = "Name")
protected String name;
@XmlElement(name = "IATA_Number")
protected String iataNumber;
/**
* Gets the value of the agencyID property.
*
* @return
* possible object is
* {@link AgencyIDType }
*
*/
public AgencyIDType getAgencyID() {
return agencyID;
}
/**
* Sets the value of the agencyID property.
*
* @param value
* allowed object is
* {@link AgencyIDType }
*
*/
public void setAgencyID(AgencyIDType value) {
this.agencyID = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the iataNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIATANumber() {
return iataNumber;
}
/**
* Sets the value of the iataNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIATANumber(String value) {
this.iataNumber = value;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AirportCode">
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>AirportCitySimpleType">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}RadiusAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
* </element>
* <element name="Date" type="{http://www.w3.org/2001/XMLSchema}date"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"airportCode",
"date"
})
public static class Departure {
@XmlElement(name = "AirportCode", required = true)
protected OrderListRS.Response.Order.Departure.AirportCode airportCode;
@XmlElement(name = "Date", required = true)
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar date;
/**
* Gets the value of the airportCode property.
*
* @return
* possible object is
* {@link OrderListRS.Response.Order.Departure.AirportCode }
*
*/
public OrderListRS.Response.Order.Departure.AirportCode getAirportCode() {
return airportCode;
}
/**
* Sets the value of the airportCode property.
*
* @param value
* allowed object is
* {@link OrderListRS.Response.Order.Departure.AirportCode }
*
*/
public void setAirportCode(OrderListRS.Response.Order.Departure.AirportCode value) {
this.airportCode = value;
}
/**
* Gets the value of the date property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getDate() {
return date;
}
/**
* Sets the value of the date property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setDate(XMLGregorianCalendar value) {
this.date = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <simpleContent>
* <extension base="<http://www.iata.org/IATA/EDIST>AirportCitySimpleType">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}RadiusAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"value"
})
public static class AirportCode {
@XmlValue
protected String value;
@XmlAttribute(name = "Application")
protected String application;
@XmlAttribute(name = "Area")
@XmlSchemaType(name = "positiveInteger")
protected BigInteger area;
@XmlAttribute(name = "UOM")
protected DistanceUnitListType uom;
/**
* Examples: JFK, NYC
*
* @return
* possible object is
* {@link String }
*
*/
public String getValue() {
return value;
}
/**
* Sets the value of the value property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setValue(String value) {
this.value = value;
}
/**
* Gets the value of the application property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getApplication() {
return application;
}
/**
* Sets the value of the application property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setApplication(String value) {
this.application = value;
}
/**
* Gets the value of the area property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getArea() {
return area;
}
/**
* Sets the value of the area property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setArea(BigInteger value) {
this.area = value;
}
/**
* Gets the value of the uom property.
*
* @return
* possible object is
* {@link DistanceUnitListType }
*
*/
public DistanceUnitListType getUOM() {
return uom;
}
/**
* Sets the value of the uom property.
*
* @param value
* allowed object is
* {@link DistanceUnitListType }
*
*/
public void setUOM(DistanceUnitListType value) {
this.uom = value;
}
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <simpleContent>
* <extension base="<http://www.w3.org/2001/XMLSchema>positiveInteger">
* <attGroup ref="{http://www.iata.org/IATA/EDIST}ObjAssociationAttrGroup"/>
* </extension>
* </simpleContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"value"
})
public static class NbrInParty {
@XmlValue
@XmlSchemaType(name = "positiveInteger")
protected BigInteger value;
@XmlAttribute(name = "refs")
@XmlIDREF
protected List