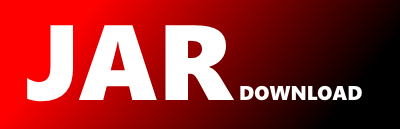
org.iata.ndc.schema.SeatAvailabilityRQ Maven / Gradle / Ivy
Show all versions of ndc-jaxb Show documentation
package org.iata.ndc.schema;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.iata.org/IATA/EDIST}PointOfSale" minOccurs="0"/>
* <element ref="{http://www.iata.org/IATA/EDIST}Document"/>
* <element ref="{http://www.iata.org/IATA/EDIST}Party"/>
* <element name="Parameters" type="{http://www.iata.org/IATA/EDIST}SeatAvailReqParamsType" minOccurs="0"/>
* <element ref="{http://www.iata.org/IATA/EDIST}Travelers" minOccurs="0"/>
* <element name="Qualifiers" type="{http://www.iata.org/IATA/EDIST}QualifiersType" minOccurs="0"/>
* <choice>
* <element ref="{http://www.iata.org/IATA/EDIST}ShoppingResponseIDs"/>
* <element name="Query">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="OriginDestination" type="{http://www.iata.org/IATA/EDIST}FlightInfoAssocType" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://www.iata.org/IATA/EDIST}OrderID" minOccurs="0"/>
* <element ref="{http://www.iata.org/IATA/EDIST}BookingReference" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </choice>
* <element name="JourneyData" type="{http://www.iata.org/IATA/EDIST}ItineraryType" minOccurs="0"/>
* <element name="DataList" type="{http://www.iata.org/IATA/EDIST}DataListType" minOccurs="0"/>
* <element name="Metadata" type="{http://www.iata.org/IATA/EDIST}SeatAvailReqMetadataType" minOccurs="0"/>
* <element ref="{http://www.iata.org/IATA/EDIST}Policies" minOccurs="0"/>
* </sequence>
* <attGroup ref="{http://www.iata.org/IATA/EDIST}IATA_PayloadStdAttributes"/>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"pointOfSale",
"document",
"party",
"parameters",
"travelers",
"qualifiers",
"shoppingResponseIDs",
"query",
"journeyData",
"dataList",
"metadata",
"policies"
})
@XmlRootElement(name = "SeatAvailabilityRQ")
public class SeatAvailabilityRQ {
@XmlElement(name = "PointOfSale")
protected PointOfSaleType pointOfSale;
@XmlElement(name = "Document", required = true)
protected MsgDocumentType document;
@XmlElement(name = "Party", required = true)
protected MsgPartiesType party;
@XmlElement(name = "Parameters")
protected SeatAvailReqParamsType parameters;
@XmlElementWrapper(name = "Travelers")
@XmlElement(name = "Traveler", namespace = "http://www.iata.org/IATA/EDIST")
protected List travelers;
@XmlElementWrapper(name = "Qualifiers")
@XmlElement(name = "Qualifier", namespace = "http://www.iata.org/IATA/EDIST")
protected List qualifiers;
@XmlElement(name = "ShoppingResponseIDs")
protected ShoppingResponseIDType shoppingResponseIDs;
@XmlElement(name = "Query")
protected SeatAvailabilityRQ.Query query;
@XmlElement(name = "JourneyData")
protected ItineraryType journeyData;
@XmlElement(name = "DataList")
protected DataListType dataList;
@XmlElement(name = "Metadata")
protected SeatAvailReqMetadataType metadata;
@XmlElementWrapper(name = "Policies")
@XmlElement(name = "Policy", namespace = "http://www.iata.org/IATA/EDIST")
protected List policies;
@XmlAttribute(name = "EchoToken")
protected String echoToken;
@XmlAttribute(name = "TimeStamp")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar timeStamp;
@XmlAttribute(name = "Target")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String target;
@XmlAttribute(name = "Version", required = true)
protected String version;
@XmlAttribute(name = "TransactionIdentifier")
protected String transactionIdentifier;
@XmlAttribute(name = "SequenceNmbr")
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger sequenceNmbr;
@XmlAttribute(name = "TransactionStatusCode")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String transactionStatusCode;
@XmlAttribute(name = "RetransmissionIndicator")
protected Boolean retransmissionIndicator;
@XmlAttribute(name = "CorrelationID")
protected String correlationID;
@XmlAttribute(name = "AsynchronousAllowedInd")
protected Boolean asynchronousAllowedInd;
@XmlAttribute(name = "PrimaryLangID")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "language")
protected String primaryLangID;
@XmlAttribute(name = "AltLangID")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "language")
protected String altLangID;
/**
* Gets the value of the pointOfSale property.
*
* @return
* possible object is
* {@link PointOfSaleType }
*
*/
public PointOfSaleType getPointOfSale() {
return pointOfSale;
}
/**
* Sets the value of the pointOfSale property.
*
* @param value
* allowed object is
* {@link PointOfSaleType }
*
*/
public void setPointOfSale(PointOfSaleType value) {
this.pointOfSale = value;
}
/**
* Gets the value of the document property.
*
* @return
* possible object is
* {@link MsgDocumentType }
*
*/
public MsgDocumentType getDocument() {
return document;
}
/**
* Sets the value of the document property.
*
* @param value
* allowed object is
* {@link MsgDocumentType }
*
*/
public void setDocument(MsgDocumentType value) {
this.document = value;
}
/**
* Gets the value of the party property.
*
* @return
* possible object is
* {@link MsgPartiesType }
*
*/
public MsgPartiesType getParty() {
return party;
}
/**
* Sets the value of the party property.
*
* @param value
* allowed object is
* {@link MsgPartiesType }
*
*/
public void setParty(MsgPartiesType value) {
this.party = value;
}
/**
* Gets the value of the parameters property.
*
* @return
* possible object is
* {@link SeatAvailReqParamsType }
*
*/
public SeatAvailReqParamsType getParameters() {
return parameters;
}
/**
* Sets the value of the parameters property.
*
* @param value
* allowed object is
* {@link SeatAvailReqParamsType }
*
*/
public void setParameters(SeatAvailReqParamsType value) {
this.parameters = value;
}
/**
* Gets the value of the shoppingResponseIDs property.
*
* @return
* possible object is
* {@link ShoppingResponseIDType }
*
*/
public ShoppingResponseIDType getShoppingResponseIDs() {
return shoppingResponseIDs;
}
/**
* Sets the value of the shoppingResponseIDs property.
*
* @param value
* allowed object is
* {@link ShoppingResponseIDType }
*
*/
public void setShoppingResponseIDs(ShoppingResponseIDType value) {
this.shoppingResponseIDs = value;
}
/**
* Gets the value of the query property.
*
* @return
* possible object is
* {@link SeatAvailabilityRQ.Query }
*
*/
public SeatAvailabilityRQ.Query getQuery() {
return query;
}
/**
* Sets the value of the query property.
*
* @param value
* allowed object is
* {@link SeatAvailabilityRQ.Query }
*
*/
public void setQuery(SeatAvailabilityRQ.Query value) {
this.query = value;
}
/**
* Gets the value of the journeyData property.
*
* @return
* possible object is
* {@link ItineraryType }
*
*/
public ItineraryType getJourneyData() {
return journeyData;
}
/**
* Sets the value of the journeyData property.
*
* @param value
* allowed object is
* {@link ItineraryType }
*
*/
public void setJourneyData(ItineraryType value) {
this.journeyData = value;
}
/**
* Gets the value of the dataList property.
*
* @return
* possible object is
* {@link DataListType }
*
*/
public DataListType getDataList() {
return dataList;
}
/**
* Sets the value of the dataList property.
*
* @param value
* allowed object is
* {@link DataListType }
*
*/
public void setDataList(DataListType value) {
this.dataList = value;
}
/**
* Gets the value of the metadata property.
*
* @return
* possible object is
* {@link SeatAvailReqMetadataType }
*
*/
public SeatAvailReqMetadataType getMetadata() {
return metadata;
}
/**
* Sets the value of the metadata property.
*
* @param value
* allowed object is
* {@link SeatAvailReqMetadataType }
*
*/
public void setMetadata(SeatAvailReqMetadataType value) {
this.metadata = value;
}
/**
* Gets the value of the echoToken property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEchoToken() {
return echoToken;
}
/**
* Sets the value of the echoToken property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEchoToken(String value) {
this.echoToken = value;
}
/**
* Gets the value of the timeStamp property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTimeStamp() {
return timeStamp;
}
/**
* Sets the value of the timeStamp property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTimeStamp(XMLGregorianCalendar value) {
this.timeStamp = value;
}
/**
* Gets the value of the target property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTarget() {
if (target == null) {
return "Production";
} else {
return target;
}
}
/**
* Sets the value of the target property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTarget(String value) {
this.target = value;
}
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
/**
* Gets the value of the transactionIdentifier property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionIdentifier() {
return transactionIdentifier;
}
/**
* Sets the value of the transactionIdentifier property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionIdentifier(String value) {
this.transactionIdentifier = value;
}
/**
* Gets the value of the sequenceNmbr property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getSequenceNmbr() {
return sequenceNmbr;
}
/**
* Sets the value of the sequenceNmbr property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setSequenceNmbr(BigInteger value) {
this.sequenceNmbr = value;
}
/**
* Gets the value of the transactionStatusCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionStatusCode() {
return transactionStatusCode;
}
/**
* Sets the value of the transactionStatusCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionStatusCode(String value) {
this.transactionStatusCode = value;
}
/**
* Gets the value of the retransmissionIndicator property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRetransmissionIndicator() {
return retransmissionIndicator;
}
/**
* Sets the value of the retransmissionIndicator property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRetransmissionIndicator(Boolean value) {
this.retransmissionIndicator = value;
}
/**
* Gets the value of the correlationID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCorrelationID() {
return correlationID;
}
/**
* Sets the value of the correlationID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCorrelationID(String value) {
this.correlationID = value;
}
/**
* Gets the value of the asynchronousAllowedInd property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAsynchronousAllowedInd() {
return asynchronousAllowedInd;
}
/**
* Sets the value of the asynchronousAllowedInd property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setAsynchronousAllowedInd(Boolean value) {
this.asynchronousAllowedInd = value;
}
/**
* Gets the value of the primaryLangID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrimaryLangID() {
return primaryLangID;
}
/**
* Sets the value of the primaryLangID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrimaryLangID(String value) {
this.primaryLangID = value;
}
/**
* Gets the value of the altLangID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAltLangID() {
return altLangID;
}
/**
* Sets the value of the altLangID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAltLangID(String value) {
this.altLangID = value;
}
public List getTravelers() {
if (travelers == null) {
travelers = new ArrayList();
}
return travelers;
}
public void setTravelers(List travelers) {
this.travelers = travelers;
}
public List getQualifiers() {
if (qualifiers == null) {
qualifiers = new ArrayList();
}
return qualifiers;
}
public void setQualifiers(List qualifiers) {
this.qualifiers = qualifiers;
}
public List getPolicies() {
if (policies == null) {
policies = new ArrayList();
}
return policies;
}
public void setPolicies(List policies) {
this.policies = policies;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="OriginDestination" type="{http://www.iata.org/IATA/EDIST}FlightInfoAssocType" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://www.iata.org/IATA/EDIST}OrderID" minOccurs="0"/>
* <element ref="{http://www.iata.org/IATA/EDIST}BookingReference" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"originDestination",
"orderID",
"bookingReference"
})
public static class Query {
@XmlElement(name = "OriginDestination")
protected List originDestination;
@XmlElement(name = "OrderID")
protected OrderIDType orderID;
@XmlElement(name = "BookingReference")
protected BookingReference bookingReference;
/**
* Gets the value of the originDestination property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the originDestination property.
*
*
* For example, to add a new item, do as follows:
*
* getOriginDestination().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FlightInfoAssocType }
*
*
*/
public List getOriginDestination() {
if (originDestination == null) {
originDestination = new ArrayList();
}
return this.originDestination;
}
/**
* Gets the value of the orderID property.
*
* @return
* possible object is
* {@link OrderIDType }
*
*/
public OrderIDType getOrderID() {
return orderID;
}
/**
* Sets the value of the orderID property.
*
* @param value
* allowed object is
* {@link OrderIDType }
*
*/
public void setOrderID(OrderIDType value) {
this.orderID = value;
}
/**
* Gets the value of the bookingReference property.
*
* @return
* possible object is
* {@link BookingReference }
*
*/
public BookingReference getBookingReference() {
return bookingReference;
}
/**
* Sets the value of the bookingReference property.
*
* @param value
* allowed object is
* {@link BookingReference }
*
*/
public void setBookingReference(BookingReference value) {
this.bookingReference = value;
}
}
}