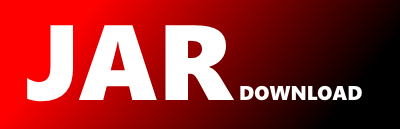
echopointng.util.QuoterKit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ibis-echo2 Show documentation
Show all versions of ibis-echo2 Show documentation
Echo2 bundled with Echo2_Extras, Echo2_FileTransfer and echopointing and various improvements/bugfixes
package echopointng.util;
/*
* This file is part of the Echo Point Project. This project is a collection
* of Components that have extended the Echo Web Application Framework.
*
* Version: MPL 1.1/GPL 2.0/LGPL 2.1
*
* The contents of this file are subject to the Mozilla Public License Version
* 1.1 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* Alternatively, the contents of this file may be used under the terms of
* either the GNU General Public License Version 2 or later (the "GPL"), or
* the GNU Lesser General Public License Version 2.1 or later (the "LGPL"),
* in which case the provisions of the GPL or the LGPL are applicable instead
* of those above. If you wish to allow use of your version of this file only
* under the terms of either the GPL or the LGPL, and not to allow others to
* use your version of this file under the terms of the MPL, indicate your
* decision by deleting the provisions above and replace them with the notice
* and other provisions required by the GPL or the LGPL. If you do not delete
* the provisions above, a recipient may use your version of this file under
* the terms of any one of the MPL, the GPL or the LGPL.
*/
/**
* This class will quote string data
*/
public class QuoterKit {
/** not instantiable */
private QuoterKit() {
}
/**
* This method will apply a "Java quote" (ie double slashes)
* to a string with the given quote char
*/
public static String quoteJ(String s, char quoteChar) {
StringBuffer sb = new StringBuffer(s.length());
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
if (c == quoteChar) {
sb.append("\\");
sb.append(quoteChar);
} else {
sb.append(c);
}
}
return sb.toString();
}
/**
* Quortes a string into HTML characters.
* @param s - the string to quote into safe HTML
* @return - safe HTML or null if s is null
*/
public static String quoteHTML(String s) {
if (s == null)
return s;
int slen = s.length();
StringBuffer sb = new StringBuffer((int) (slen*1.5));
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
switch (c) {
case '<':
sb.append("<");
break;
case '>':
sb.append(">");
break;
case '"':
sb.append(""");
break;
case '&': {
// if the next N chars are &xxx; then dont replace it
if (checkForEntities(i,slen,s)) {
sb.append(c);
continue;
}
sb.append("&");
break;
}
default :
sb.append(c);
break;
}
}
return sb.toString();
}
/**
* All the valid HTML entities according to W3CSchools :
* http://www.w3schools.com/html/html_entitiesref.asp
*/
private static String[] htmlEntities = {
""", """,
"'", "'",
"&&", "&",
"<", "<",
">", ">",
" ", " ",
"¡", "¡",
"¤", "¤",
"¢", "¢",
"£", "£",
"¥", "¥",
"¦", "¦",
"§", "§",
"¨", "¨",
"©", "©",
"ª", "ª",
"«", "«",
"¬", "¬",
"", "",
"®", "®",
"¯", "¯",
"°", "°",
"±", "±",
"²", "²",
"³", "³",
"´", "´",
"µ", "µ",
"¶", "¶",
"·", "·",
"¸", "¸",
"¹", "¹",
"º", "º",
"»", "»",
"¼", "¼",
"½", "½",
"¾", "¾",
"¿", "¿",
"×", "×",
"÷", "÷",
"À", "À",
"Á", "Á",
"Â", "Â",
"Ã", "Ã",
"Ä", "Ä",
"Å", "Å",
"Æ", "Æ",
"Ç", "Ç",
"È", "È",
"É", "É",
"Ê", "Ê",
"Ë", "Ë",
"Ì", "Ì",
"Í", "Í",
"Î", "Î",
"Ï", "Ï",
"Ð", "Ð",
"Ñ", "Ñ",
"Ò", "Ò",
"Ó", "Ó",
"Ô", "Ô",
"Õ", "Õ",
"Ö", "Ö",
"Ø", "Ø",
"Ù", "Ù",
"Ú", "Ú",
"Û", "Û",
"Ü", "Ü",
"Ý", "Ý",
"Þ", "Þ",
"ß", "ß",
"à", "à",
"á", "á",
"â", "â",
"ã", "ã",
"ä", "ä",
"å", "å",
"æ", "æ",
"ç", "ç",
"è", "è",
"é", "é",
"ê", "ê",
"ë", "ë",
"ì", "ì",
"í", "í",
"î", "î",
"ï", "ï",
"ð", "ð",
"ñ", "ñ",
"ò", "ò",
"ó", "ó",
"ô", "ô",
"õ", "õ",
"ö", "ö",
"ø", "ø",
"ù", "ù",
"ú", "ú",
"û", "û",
"ü", "ü",
"ý", "ý",
"þ", "þ",
"ÿ", "ÿ",
"Œ", "Œ",
"œ", "œ",
"Š", "Š",
"š", "š",
"Ÿ", "Ÿ",
"ˆ", "ˆ",
"˜", "˜",
" ", " ",
" ", " ",
" ", " ",
"", "",
"", "",
"", "",
"", "",
"–", "–",
"—", "—",
"‘", "‘",
"’", "’",
"‚", "‚",
"“", "“",
"”", "”",
"„", "„",
"†", "†",
"‡", "‡",
"…", "…",
"‰", "‰",
"‹", "‹",
"›", "›",
"€", "€",
"™", "™",
};
/*
* Check that the string does have any HTML entities
*/
private static boolean checkForEntities(int i, int slen, String s) {
for (int j = 0; j < htmlEntities.length; j++) {
if (checkFor(i,slen,s,htmlEntities[j]))
return true;
}
return false;
}
/*
* Check that the string does have the HTML entity
*/
private static boolean checkFor(int i, int slen, String s, String checkFor) {
int cflen = checkFor.length();
if (i <= (slen - cflen)) {
if (s.substring(i,i+cflen).equalsIgnoreCase(checkFor)) {
return true;
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy