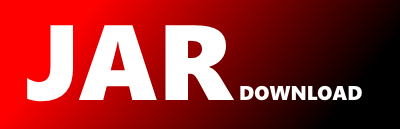
nextapp.echo2.webcontainer.propertyrender.AlignmentRender Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ibis-echo2 Show documentation
Show all versions of ibis-echo2 Show documentation
Echo2 bundled with Echo2_Extras, Echo2_FileTransfer and echopointing and various improvements/bugfixes
/*
* This file is part of the Echo Web Application Framework (hereinafter "Echo").
* Copyright (C) 2002-2009 NextApp, Inc.
*
* Version: MPL 1.1/GPL 2.0/LGPL 2.1
*
* The contents of this file are subject to the Mozilla Public License Version
* 1.1 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the
* License.
*
* Alternatively, the contents of this file may be used under the terms of
* either the GNU General Public License Version 2 or later (the "GPL"), or
* the GNU Lesser General Public License Version 2.1 or later (the "LGPL"),
* in which case the provisions of the GPL or the LGPL are applicable instead
* of those above. If you wish to allow use of your version of this file only
* under the terms of either the GPL or the LGPL, and not to allow others to
* use your version of this file under the terms of the MPL, indicate your
* decision by deleting the provisions above and replace them with the notice
* and other provisions required by the GPL or the LGPL. If you do not delete
* the provisions above, a recipient may use your version of this file under
* the terms of any one of the MPL, the GPL or the LGPL.
*/
package nextapp.echo2.webcontainer.propertyrender;
import org.w3c.dom.Element;
import nextapp.echo2.app.Alignment;
import nextapp.echo2.app.Component;
import nextapp.echo2.app.LayoutDirection;
import nextapp.echo2.webrender.output.CssStyle;
/**
* Utility class for rendering nextapp.echo2.app.Alignment
* properties to CSS.
*/
public class AlignmentRender {
/**
* Returns the horizontal property of an Alignment
object,
* with Alignment.LEADING
and Alignment.TRAILING
* automatically translated based on the layout direction of the provided
* Component
. If the provided component is null, a
* left-to-right layout direction will be assumed.
*
* @param alignment the Alignment
to analyze
* @param component the Component
to analyze
* @return the horizontal alignment constant
*/
public static int getRenderedHorizontal(Alignment alignment, Component component) {
LayoutDirection layoutDirection;
if (component == null) {
layoutDirection = LayoutDirection.LTR;
} else {
layoutDirection = component.getRenderLayoutDirection();
}
switch (alignment.getHorizontal()) {
case Alignment.LEADING:
return layoutDirection.isLeftToRight() ? Alignment.LEFT : Alignment.RIGHT;
case Alignment.TRAILING:
return layoutDirection.isLeftToRight() ? Alignment.RIGHT : Alignment.LEFT;
default:
return alignment.getHorizontal();
}
}
/**
* Renders an Alignment
property to the given element.
* The 'align' and 'valign' attributes will be set if they
* can be derived from the provided Alignment
.
* Null property values are ignored.
*
* @param element the target Element
* @param alignment the property value
*/
public static void renderToElement(Element element, Alignment alignment) {
renderToElement(element, alignment, null);
}
/**
* Renders an Alignment
property to the given element.
* The 'align' and 'valign' attributes will be set if they
* can be derived from the provided Alignment
.
* Null property values are ignored.
*
* @param element the target Element
* @param alignment the property value
* @param component The Component
for which the style is being
* rendered (necessary for property translation of leading/trailing
* alignment settings).
*/
public static void renderToElement(Element element, Alignment alignment, Component component) {
if (alignment == null) {
return;
}
String horizontal = getHorizontalCssAttributeValue(alignment, component);
if (horizontal != null) {
element.setAttribute("align", horizontal);
}
String vertical = getVerticalCssAttributeValue(alignment);
if (vertical != null) {
element.setAttribute("valign", vertical);
}
}
/**
* Renders an Alignment
property to the given CSS style.
* The 'text-align' and 'vertical-align' properties will be set if they
* can be derived from the provided Alignment
.
* Null property values are ignored.
*
* @param cssStyle the target CssStyle
* @param alignment the property value
*/
public static void renderToStyle(CssStyle cssStyle, Alignment alignment) {
renderToStyle(cssStyle, alignment, null);
}
/**
* Renders an Alignment
property to the given CSS style.
* The 'text-align' and 'vertical-align' properties will be set if they
* can be derived from the provided Alignment
.
* Null property values are ignored.
*
* @param cssStyle the target CssStyle
* @param component The Component
for which the style is being
* rendered (necessary for property translation of leading/trailing
* alignment settings).
* @param alignment the property value
*/
public static void renderToStyle(CssStyle cssStyle, Alignment alignment, Component component) {
if (alignment == null) {
return;
}
String horizontal = getHorizontalCssAttributeValue(alignment, component);
if (horizontal != null) {
cssStyle.setAttribute("text-align", horizontal);
}
String vertical = getVerticalCssAttributeValue(alignment);
if (vertical != null) {
cssStyle.setAttribute("vertical-align", vertical);
}
}
/**
* Determines the CSS attribute value of the horizontal property of the
* specified Alignment
. The provided Component
* is used to determine the rendered alignment setting for
* Alignment.LEADING
and alignment.TRAILING
* values.
*
* @param alignment the Alignment
property value
* @param component the Component
to use in order to determine
* appropriate rendered values for Alignment.LEADING
/
* Alignment.TRAILING
(this value may be null, in
* which case an LTR layout direction will be assumed)
* @return the horizontal CSS attribute value, or null if it is not
* specified by the Alignment
*/
private static String getHorizontalCssAttributeValue(Alignment alignment, Component component) {
switch (getRenderedHorizontal(alignment, component)) {
case Alignment.LEFT:
return "left";
case Alignment.CENTER:
return "center";
case Alignment.RIGHT:
return "right";
default:
return null;
}
}
/**
* Determines the CSS attribute value of the vertical property of the
* specified Alignment
.
*
* @param alignment the Alignment
property value
* @return the CSS attribute value, or null if it is not specified by the
* Alignment
*/
private static String getVerticalCssAttributeValue(Alignment alignment) {
switch (alignment.getVertical()) {
case Alignment.TOP:
return "top";
case Alignment.CENTER:
return "middle";
case Alignment.BOTTOM:
return "bottom";
default:
return null;
}
}
/** Non-instantiable class. */
private AlignmentRender() { }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy