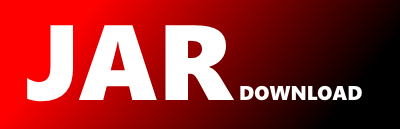
org.icefaces.ace.component.datatable.DataTableMeta Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of icefaces-ace Show documentation
Show all versions of icefaces-ace Show documentation
The advanced component library for ICEfaces.
/*
* Copyright 2004-2013 ICEsoft Technologies Canada Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the
* License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an "AS
* IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.icefaces.ace.component.datatable;
import org.icefaces.ace.meta.annotation.*;
import org.icefaces.ace.meta.baseMeta.UIDataMeta;
import org.icefaces.ace.model.table.CellSelections;
import org.icefaces.ace.model.table.RowStateMap;
import org.icefaces.ace.resources.ACEResourceNames;
import org.icefaces.resources.ICEResourceDependencies;
import org.icefaces.resources.ICEResourceDependency;
import org.icefaces.resources.ICEResourceLibrary;
import javax.el.MethodExpression;
import java.util.List;
import java.util.Map;
@Component(
tagName = "dataTable",
componentClass = "org.icefaces.ace.component.datatable.DataTable",
generatedClass = "org.icefaces.ace.component.datatable.DataTableBase",
rendererClass = "org.icefaces.ace.component.datatable.DataTableRenderer",
extendsClass = "javax.faces.component.UIData",
componentType = "org.icefaces.ace.component.DataTable",
rendererType = "org.icefaces.ace.component.DataTableRenderer",
componentFamily = "org.icefaces.ace.DataTable",
tlddoc = "Renders an HTML table element. This table and its associated " +
"components offers support for features like: scrolling, " +
"sorting, filtering, row selection, row editing, lazy loading " +
"and expandable subrows and subpanels." +
"For more information, see the DataTable Wiki Documentation.
"
)
@ICEResourceLibrary(ACEResourceNames.ACE_LIBRARY)
@ICEResourceDependencies({
@ICEResourceDependency(name=ACEResourceNames.COMBINED_CSS),
@ICEResourceDependency(name=ACEResourceNames.JQUERY_JS),
@ICEResourceDependency(name="util/ace-datatable.js")
})
@ClientBehaviorHolder(events = {
@ClientEvent(name="page", javadoc="Fired when the page is changed on the DataTable.",
tlddoc="Fired when the page is changed on the DataTable.", defaultRender="@all", defaultExecute="@this"),
@ClientEvent(name="select", javadoc="Fired when a row or cell is selected on the DataTable.",
tlddoc="Fired when a row or cell is selected on the DataTable.", defaultRender="@all", defaultExecute="@this"),
@ClientEvent(name="deselect", javadoc="Fired when a row or cell is deselected on the DataTable.",
tlddoc="Fired when a row or cell is deselected on the DataTable.", defaultRender="@all", defaultExecute="@this"),
@ClientEvent(name="sort", javadoc="Fired when a change to the current sort occurs on the DataTable.",
tlddoc="Fired when a change to the current sort occurs on the DataTable.", defaultRender="@all", defaultExecute="@this"),
@ClientEvent(name="filter", javadoc="Fired when a change to the current filters occurs on the DataTable.",
tlddoc="Fired when a change to the current filters occurs on the DataTable.", defaultRender="@all", defaultExecute="@this"),
@ClientEvent(name="reorder", javadoc="Fired when a column is dragged and dropped into a new ordering.",
tlddoc="Fired when a column is dragged and dropped into a new ordering.", defaultRender="@all", defaultExecute="@this"),
// Edit has custom render and execute, @none is just a null placeholder for additional update/execute fields
@ClientEvent(name="editStart", javadoc="Fired when a row is enabled for editing.",
tlddoc="Fired when a row is enabled for editing.", defaultRender="@this", defaultExecute="@this"),
@ClientEvent(name="editSubmit", javadoc="Fired when a row is submits its edits.",
tlddoc="Fired when a row is submits its edits.", defaultRender="@all", defaultExecute="@this"),
@ClientEvent(name="editCancel", javadoc="Fired when a row cancels an in-progress edit.",
tlddoc="Fired when a row cancels an in-progress edit.", defaultRender="@this", defaultExecute="@this"),
@ClientEvent(name="expand", javadoc="Fired when a child ExpansionToggler component is clicked to expand.",
tlddoc="Fired when a child ExpansionToggler component is clicked to expand.", defaultRender="@all", defaultExecute="@this"),
@ClientEvent(name="contract", javadoc="Fired when a child ExpansionToggler component is clicked to contract.",
tlddoc="Fired when a child ExpansionToggler component is clicked to contract.", defaultRender="@all", defaultExecute="@this"),
@ClientEvent(name="pin", javadoc="Fired when a column is added to the pinning region of the table.",
tlddoc="Fired when a column is added to the pinning region of the table.", defaultRender="@this", defaultExecute="@this"),
@ClientEvent(name="unpin", javadoc="Fired when a column is removed to the pinning region of the table.",
tlddoc="Fired when a column is removed to the pinning region of the table.", defaultRender="@this", defaultExecute="@this")} ,
defaultEvent = "select"
)
public class DataTableMeta extends UIDataMeta {
/* ##################################################################### */
/* ############################ Misc. Prop. ############################ */
/* ##################################################################### */
@Property(tlddoc = "The JavaScript global component instance name. " +
"Must be unique among components on a page. ")
private String widgetVar;
@Property(tlddoc = "Disable all features of the data table.", defaultValue = "false",
defaultValueType= DefaultValueType.EXPRESSION)
private boolean disabled;
@Property(tlddoc = "The request-scope attribute (if any) under which the data " +
"object index for the current row will be exposed when iterating.")
private String rowIndexVar;
@Property(tlddoc = "The request-scope attribute (if any) under which the table" +
" state object for the current row will be exposed when iterating.", defaultValue = "rowState")
private String rowStateVar;
@Property(tlddoc = "Enables lazy loading. Lazy loading expects the 'value' property to reference " +
"an instance of LazyDataModel, an interface to support incremental fetching of " +
"table entities.")
private boolean lazy;
@Property(tlddoc = "Defines a tabindex to be shared by all keyboard navigable elements of the table. " +
"This includes sort controls, filter fields and individual rows themselves.",
defaultValue = "0",
defaultValueType = DefaultValueType.EXPRESSION)
private Integer tabIndex;
@Property(defaultValue="0",
defaultValueType= DefaultValueType.EXPRESSION,
implementation=Implementation.GENERATE,
tlddoc="The number of rows to be displayed, or zero to display the entire " +
"set of available rows.")
private int rows;
@Property(tlddoc = "Define a string to render when there are no records to display.")
private String emptyMessage;
@Property(tlddoc = "Disables sorting for multiple columns at once. Sorting " +
"is enabled by the use of sortBy on ace:column components.",
defaultValue = "false", defaultValueType = DefaultValueType.EXPRESSION)
private boolean singleSort;
@Property(tlddoc = "Defines a map of your row data objects to UI states. Row-level " +
"features (selection, expansion, etc.) are manipulable through this repository.")
private RowStateMap stateMap;
@Property(tlddoc = "Enable the decoding of child components during table feature " +
"requests. The table attempts to decode children whenever it is executed, " +
"meaning whenever a parent region is submitted, or the table submits itself " +
"to paginate, make a selection, reorder columns, or any other feature. " +
"Decoding children during feature requests can result in unwanted input " +
"submission (during pagination for example), so by default this component " +
"suppresses child decoding whenever submitting itself. To cause decoding in the " +
"children of the table, use the row editing feature for row-scoped input " +
"decoding, ajax submit the form (or other table parent) for broad decoding" +
"or enable this option to submit during all table operations.",
defaultValue = "false", defaultValueType = DefaultValueType.EXPRESSION)
private Boolean alwaysExecuteContents;
@Property(tlddoc = "Enable the the client to revert the edited row with the default state following a failed edit." +
" By default when validation fails during a row editing request the row remains in editing mode.",
defaultValue = "false", defaultValueType = DefaultValueType.EXPRESSION)
private Boolean toggleOnInvalidEdit;
@Property(tlddoc = "Enable the default handling of the scrollable table when " +
"rendered into a hidden page region. The table attempts to poll its hidden " +
"status, looking for when it is shown and then call the scrollable table sizing " +
"JavaScript. This can be expensive in environments of reduced JavaScript performance with " +
"many tables and a complex DOM. When this is disabled, upon revealing a hidden " +
"scrollable table, to ensure it is sized correctly the JS " +
"'tableWidgetVar.resizeScrolling()' function must be called.",
defaultValue = "true", defaultValueType = DefaultValueType.EXPRESSION)
private Boolean hiddenScrollableSizing;
@Property(tlddoc = "Enable the default scrollable table behaviour of using JavaScript to size a " +
"table header and footer that are in a fixed position regardless of table body scrolling.",
defaultValue = "true", defaultValueType = DefaultValueType.EXPRESSION)
private Boolean staticHeaders;
// Map from row data to field names (cells) that are selected
@Field(defaultValue = "null", defaultValueIsStringLiteral = false)
protected Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy