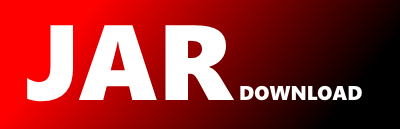
com.icesoft.faces.component.ext.HtmlInputSecret Maven / Gradle / Ivy
Show all versions of icefaces-compat Show documentation
/*
* Copyright 2004-2012 ICEsoft Technologies Canada Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the
* License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an "AS
* IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package com.icesoft.faces.component.ext;
import com.icesoft.faces.component.CSS_DEFAULT;
import com.icesoft.faces.component.PORTLET_CSS_DEFAULT;
import com.icesoft.faces.component.ext.taglib.Util;
import com.icesoft.faces.context.effects.CurrentStyle;
import com.icesoft.faces.context.effects.Effect;
import com.icesoft.faces.context.effects.JavascriptContext;
import com.icesoft.faces.util.CoreUtils;
import com.icesoft.util.CoreComponentUtils;
import javax.faces.context.FacesContext;
import javax.faces.el.ValueBinding;
/**
* This is an extension of com.icesoft.faces.component.ext.HtmlInputText, which
* provides some additional behavior to this component such as: - default
* classes for enabled and disabled state
- provides full and partial
* submit mechanism
- changes the component's enabled and rendered state
* based on the authentication
- adds effects to the component
* - allows to set the action and actionListener for this component
*/
public class HtmlInputSecret extends HtmlInputText {
public static final String COMPONENT_TYPE =
"com.icesoft.faces.HtmlInputSecret";
public static final String RENDERER_TYPE = "com.icesoft.faces.Secret";
private static final boolean DEFAULT_VISIBLE = true;
private String styleClass = null;
private boolean redisplay = false;
private boolean redisplaySet = false;
private boolean focus = false;
private Effect onclickeffect;
private Effect ondblclickeffect;
private Effect onmousedowneffect;
private Effect onmouseupeffect;
private Effect onmousemoveeffect;
private Effect onmouseovereffect;
private Effect onmouseouteffect;
private Effect onchangeeffect;
private Effect onkeypresseffect;
private Effect onkeydowneffect;
private Effect onkeyupeffect;
private CurrentStyle currentStyle;
private Effect effect;
private Boolean visible = null;
public HtmlInputSecret() {
super();
setRendererType(RENDERER_TYPE);
}
/**
* This method is used to communicate a focus request from the application
* to the client browser.
*/
public void requestFocus() {
CoreComponentUtils.setFocusId( "null" );
JavascriptContext.focus(FacesContext.getCurrentInstance(),
this.getClientId(
FacesContext.getCurrentInstance()));
}
public void setValueBinding(String s, ValueBinding vb) {
if (s != null && s.indexOf("effect") != -1) {
// If this is an effect attribute make sure Ice Extras is included
JavascriptContext.includeLib(JavascriptContext.ICE_EXTRAS,
getFacesContext());
}
super.setValueBinding(s, vb);
}
/**
* Set the value of the effect
property.
*/
public void setEffect(Effect effect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.effect = effect;
}
/**
* Return the value of the effect
property.
*/
public Effect getEffect() {
if (effect != null) {
return effect;
}
ValueBinding vb = getValueBinding("effect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the visible
property.
*/
public void setVisible(boolean visible) {
this.visible = Boolean.valueOf(visible);
}
/**
* Return the value of the visible
property.
*/
public boolean getVisible() {
if (visible != null) {
return visible.booleanValue();
}
ValueBinding vb = getValueBinding("visible");
Boolean boolVal =
vb != null ? (Boolean) vb.getValue(getFacesContext()) : null;
return boolVal != null ? boolVal.booleanValue() : DEFAULT_VISIBLE;
}
/**
* Set the value of the styleClass
property.
*/
public void setStyleClass(String styleClass) {
this.styleClass = styleClass;
}
/**
* Return the value of the styleClass
property.
*/
public String getStyleClass() {
return Util.getQualifiedStyleClass(this,
styleClass,
CSS_DEFAULT.INPUT_SECRET_DEFAULT_STYLE_CLASS,
"styleClass",
isDisabled(),
PORTLET_CSS_DEFAULT.PORTLET_FORM_INPUT_FIELD);
}
/**
* Return the value of the rendered
property.
*/
public boolean isRendered() {
if (!Util.isRenderedOnUserRole(this)) {
return false;
}
return super.isRendered();
}
/**
* Set the value of the redisplay
property.
*/
public void setRedisplay(boolean redisplay) {
this.redisplay = redisplay;
}
/**
* Return the value of the redisplay
property.
*/
public boolean isRedisplay() {
if (this.redisplaySet) {
return this.redisplay;
}
ValueBinding _vb = getValueBinding("redisplay");
if (_vb != null) {
Object _result = _vb.getValue(getFacesContext());
if (_result == null) {
return false;
} else {
return ((Boolean) _result).booleanValue();
}
} else {
return this.redisplay;
}
}
/**
* Set the value of the focus
property.
*/
public void setFocus(boolean focus) {
this.focus = focus;
}
/**
* Return the value of the focus
property.
*/
public boolean hasFocus() {
return focus;
}
/**
* Return the value of the onclickeffect
property.
*/
public Effect getOnclickeffect() {
if (onclickeffect != null) {
return onclickeffect;
}
ValueBinding vb = getValueBinding("onclickeffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onclickeffect
property.
*/
public void setOnclickeffect(Effect onclickeffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onclickeffect = onclickeffect;
}
/**
* Return the value of the ondblclickeffect
property.
*/
public Effect getOndblclickeffect() {
if (ondblclickeffect != null) {
return ondblclickeffect;
}
ValueBinding vb = getValueBinding("ondblclickeffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the ondblclickeffect
property.
*/
public void setOndblclickeffect(Effect ondblclickeffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.ondblclickeffect = ondblclickeffect;
}
/**
* Return the value of the onmousedowneffect
property.
*/
public Effect getOnmousedowneffect() {
if (onmousedowneffect != null) {
return onmousedowneffect;
}
ValueBinding vb = getValueBinding("onmousedowneffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onmousedowneffect
property.
*/
public void setOnmousedowneffect(Effect onmousedowneffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onmousedowneffect = onmousedowneffect;
}
/**
* Return the value of the onmouseupeffect
property.
*/
public Effect getOnmouseupeffect() {
if (onmouseupeffect != null) {
return onmouseupeffect;
}
ValueBinding vb = getValueBinding("onmouseupeffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onmouseupeffect
property.
*/
public void setOnmouseupeffect(Effect onmouseupeffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onmouseupeffect = onmouseupeffect;
}
/**
* Return the value of the onmousemoveeffect
property.
*/
public Effect getOnmousemoveeffect() {
if (onmousemoveeffect != null) {
return onmousemoveeffect;
}
ValueBinding vb = getValueBinding("onmousemoveeffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onmousemoveeffect
property.
*/
public void setOnmousemoveeffect(Effect onmousemoveeffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onmousemoveeffect = onmousemoveeffect;
}
/**
* Return the value of the onmouseovereffect
property.
*/
public Effect getOnmouseovereffect() {
if (onmouseovereffect != null) {
return onmouseovereffect;
}
ValueBinding vb = getValueBinding("onmouseovereffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onmouseovereffect
property.
*/
public void setOnmouseovereffect(Effect onmouseovereffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onmouseovereffect = onmouseovereffect;
}
/**
* Return the value of the onmouseouteffect
property.
*/
public Effect getOnmouseouteffect() {
if (onmouseouteffect != null) {
return onmouseouteffect;
}
ValueBinding vb = getValueBinding("onmouseouteffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onmouseouteffect
property.
*/
public void setOnmouseouteffect(Effect onmouseouteffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onmouseouteffect = onmouseouteffect;
}
/**
* Return the value of the onchangeeffect
property.
*/
public Effect getOnchangeeffect() {
if (onchangeeffect != null) {
return onchangeeffect;
}
ValueBinding vb = getValueBinding("onchangeeffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onchangeeffect
property.
*/
public void setOnchangeeffect(Effect onchangeeffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onchangeeffect = onchangeeffect;
}
/**
* Return the value of the onkeypresseffect
property.
*/
public Effect getOnkeypresseffect() {
if (onkeypresseffect != null) {
return onkeypresseffect;
}
ValueBinding vb = getValueBinding("onkeypresseffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onkeypresseffect
property.
*/
public void setOnkeypresseffect(Effect onkeypresseffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onkeypresseffect = onkeypresseffect;
}
/**
* Return the value of the onkeydowneffect
property.
*/
public Effect getOnkeydowneffect() {
if (onkeydowneffect != null) {
return onkeydowneffect;
}
ValueBinding vb = getValueBinding("onkeydowneffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onkeydowneffect
property.
*/
public void setOnkeydowneffect(Effect onkeydowneffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onkeydowneffect = onkeydowneffect;
}
/**
* Return the value of the onkeyupeffect
property.
*/
public Effect getOnkeyupeffect() {
if (onkeyupeffect != null) {
return onkeyupeffect;
}
ValueBinding vb = getValueBinding("onkeyupeffect");
return vb != null ? (Effect) vb.getValue(getFacesContext()) : null;
}
/**
* Set the value of the onkeyupeffect
property.
*/
public void setOnkeyupeffect(Effect onkeyupeffect) {
JavascriptContext
.includeLib(JavascriptContext.ICE_EXTRAS, getFacesContext());
this.onkeyupeffect = onkeyupeffect;
}
/**
* Return the value of the currentStyle
property.
*/
public CurrentStyle getCurrentStyle() {
return currentStyle;
}
/**
* Set the value of the currentStyle
property.
*/
public void setCurrentStyle(CurrentStyle currentStyle) {
this.currentStyle = currentStyle;
}
private String autocomplete;
/**
* Set the value of the autocomplete
property.
*/
public void setAutocomplete(String autocomplete) {
this.autocomplete = autocomplete;
}
/**
* Return the value of the autocomplete
property.
*/
public String getAutocomplete() {
if (autocomplete != null) {
return autocomplete;
}
ValueBinding vb = getValueBinding("autocomplete");
return vb != null ? (String) vb.getValue(getFacesContext()) : null;
}
/**
* Gets the state of the instance as a Serializable
* Object.
*/
public Object saveState(FacesContext context) {
Object values[] = new Object[23];
values[0] = super.saveState(context);
values[1] = onkeyupeffect;
values[2] = styleClass;
values[3] = redisplay ? Boolean.TRUE : Boolean.FALSE;
values[4] = redisplaySet ? Boolean.TRUE : Boolean.FALSE;
values[5] = onclickeffect;
values[6] = ondblclickeffect;
values[7] = onmousedowneffect;
values[8] = onmouseupeffect;
values[9] = onmousemoveeffect;
values[10] = onmouseovereffect;
values[11] = onmouseouteffect;
values[12] = onchangeeffect;
values[15] = onkeypresseffect;
values[16] = onkeydowneffect;
values[17] = currentStyle;
values[18] = effect;
values[19] = visible;
values[20] = autocomplete;
values[21] = Boolean.valueOf(focus);
values[22] = getSubmittedValue();
return ((Object) (values));
}
/**
* Perform any processing required to restore the state from the entries
* in the state Object.
*/
public void restoreState(FacesContext context, Object state) {
Object values[] = (Object[]) state;
super.restoreState(context, values[0]);
onkeyupeffect = (Effect) values[1];
styleClass = (String) values[2];
redisplay = ((Boolean) values[3]).booleanValue();
redisplaySet = ((Boolean) values[3]).booleanValue();
onclickeffect = (Effect) values[5];
ondblclickeffect = (Effect) values[6];
onmousedowneffect = (Effect) values[7];
onmouseupeffect = (Effect) values[8];
onmousemoveeffect = (Effect) values[9];
onmouseovereffect = (Effect) values[10];
onmouseouteffect = (Effect) values[11];
onchangeeffect = (Effect) values[12];
onkeypresseffect = (Effect) values[15];
onkeydowneffect = (Effect) values[16];
currentStyle = (CurrentStyle) values[17];
effect = (Effect) values[18];
visible = (Boolean) values[19];
autocomplete = (String) values[20];
focus = ((Boolean) values[21]).booleanValue();
setSubmittedValue(values[22]);
}
}