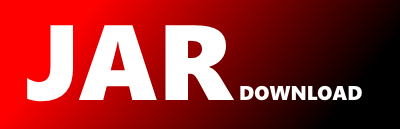
org.icefaces.mobi.component.dataview.DataViewRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of icefaces-mobi Show documentation
Show all versions of icefaces-mobi Show documentation
${icefaces.product.name} MOBI Component Library
/*
* Copyright 2004-2014 ICEsoft Technologies Canada Corp.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the
* License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an "AS
* IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.icefaces.mobi.component.dataview;
import org.icefaces.impl.util.DOMUtils;
import org.icefaces.ace.component.textentry.TextEntry;
import org.icefaces.ace.util.ComponentUtils;
import org.icefaces.ace.util.HTML;
import org.icefaces.mobi.model.dataview.DataViewColumnModel;
import org.icefaces.mobi.model.dataview.DataViewColumnsModel;
import org.icefaces.mobi.model.dataview.DataViewDataModel;
import org.icefaces.mobi.model.dataview.IndexedIterator;
import org.icefaces.mobi.renderkit.CoreRenderer;
import javax.el.ELContext;
import javax.el.ValueExpression;
import javax.faces.component.*;
import javax.faces.component.html.*;
import javax.faces.context.FacesContext;
import javax.faces.context.ResponseWriter;
import java.io.IOException;
import java.io.OptionalDataException;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.*;
import java.util.logging.Logger;
public class DataViewRenderer extends CoreRenderer {
private static final Logger logger = Logger.getLogger(DataViewRenderer.class.getName());
public void decode(FacesContext facesContext, UIComponent uiComponent) {
Map requestMap = facesContext.getExternalContext().getRequestParameterMap();
Object sourceId = requestMap.get("ice.event.captured");
if (sourceId != null && sourceId.toString().equals(uiComponent.getClientId(facesContext))) {
decodeBehaviors(facesContext, (DataView) uiComponent);
}
}
@Override
public void encodeBegin(FacesContext context, UIComponent component) throws IOException {
DataView dataView = (DataView) component;
String dvId = dataView.getClientId();
ResponseWriter writer = context.getResponseWriter();
writer.startElement(HTML.DIV_ELEM, component);
writer.writeAttribute(HTML.ID_ATTR, dvId, null);
String styleClass = DataView.DATAVIEW_CLASS;
String userClass = dataView.getStyleClass();
if (userClass != null) styleClass += " " + userClass;
writer.writeAttribute(HTML.CLASS_ATTR, styleClass, null);
String userStyle = dataView.getStyle();
if (userStyle != null)
writer.writeAttribute(HTML.STYLE_ATTR, userStyle, null);
}
@Override
public void encodeChildren(FacesContext context,
UIComponent component) throws IOException {
DataView dataView = (DataView) component;
ResponseWriter writer = context.getResponseWriter();
encodeDetails(context, writer, dataView);
encodeColumns(context, writer, dataView);
}
@Override
public void encodeEnd(FacesContext context, UIComponent component) throws IOException {
ResponseWriter writer = context.getResponseWriter();
encodeScript(context, writer, (DataView)component);
writer.endElement(HTML.DIV_ELEM);
}
private void encodeScript(FacesContext context, ResponseWriter writer, DataView dataView) throws IOException {
String dvId = dataView.getClientId();
writer.startElement(HTML.SPAN_ELEM, null);
writer.writeAttribute(HTML.ID_ATTR, dvId + "_jswrp", null);
writer.startElement(HTML.SCRIPT_ELEM, null);
writer.writeAttribute(HTML.TYPE_ATTR, HTML.SCRIPT_TYPE_TEXT_JAVASCRIPT, null);
String activationMode = dataView.getClientBehaviors().isEmpty() ? "client" : "server";
String cfg = "{";
cfg += "active:'" + activationMode + "'";
cfg += ",disabled:" + dataView.isDisabled();
cfg += encodeClientBehaviors(context, dataView, "action").toString();
cfg += "}";
String js =
"ice.mobi.dataView.create("
+ '"' + dvId + '"'
+ ", " + cfg
+ ");";
writer.writeText(js, null);
writer.endElement(HTML.SCRIPT_ELEM);
writer.endElement(HTML.SPAN_ELEM);
}
private void encodeColumns(FacesContext context,
ResponseWriter writer,
DataView dataView) throws IOException {
DataViewColumns columns = dataView.getColumns();
String var = dataView.getVar();
if (columns == null) encodeEmptyBodyTable(writer);
else {
writer.startElement(HTML.DIV_ELEM, null);
writer.writeAttribute(HTML.CLASS_ATTR, DataView.DATAVIEW_MASTER_CLASS, null);
DataViewColumnsModel columnModel = columns.getModel();
DataViewDataModel dataModel = dataView.getDataModel();
if (columnModel.hasHeaders())
encodeHeaders(writer, columnModel, dataModel, true);
encodeRows(context, writer, dataView, var, columnModel, dataModel);
if (columnModel.hasFooters())
encodeFooters(writer, columnModel, dataModel, true);
writer.endElement(HTML.DIV_ELEM);
}
}
private void encodeEmptyBodyTable(ResponseWriter writer) throws IOException {
writer.startElement(HTML.DIV_ELEM, null);
writer.writeAttribute(HTML.CLASS_ATTR, DataView.DATAVIEW_MASTER_CLASS, null);
writer.startElement(HTML.DIV_ELEM, null);
writer.startElement(HTML.TABLE_ELEM, null);
writer.writeAttribute(HTML.CLASS_ATTR, DataView.DATAVIEW_BODY_CLASS, null);
writer.endElement(HTML.TABLE_ELEM);
writer.endElement(HTML.DIV_ELEM);
writer.endElement(HTML.DIV_ELEM);
}
private void encodeHeaders(ResponseWriter writer,
DataViewColumnsModel columnModel,
DataViewDataModel dataModel,
boolean writeTable) throws IOException {
/* Skip table when writing duplicate alignment header */
if (writeTable) {
writer.startElement(HTML.TABLE_ELEM, null);
writer.writeAttribute(HTML.CLASS_ATTR, DataView.DATAVIEW_HEAD_CLASS, null);
}
writer.startElement(HTML.THEAD_ELEM, null);
writer.startElement(HTML.TR_ELEM, null);
writer.writeAttribute(HTML.CLASS_ATTR, DataView.DATAVIEW_HEADER_ROW_CLASS, null);
for (IndexedIterator columnIter = columnModel.iterator(); columnIter.hasNext();) {
DataViewColumnModel column = columnIter.next();
int index = columnIter.getIndex();
if (column.isRendered()) {
writer.startElement(HTML.TH_ELEM, null);
String className = getColumnStyleClass(column, index);
writer.writeAttribute(HTML.CLASS_ATTR, className, null);
if (column.getHeaderText() != null)
writer.write(column.getHeaderText());
if (column.isSortable()) {
writer.startElement("i", null);
writer.writeAttribute(HTML.CLASS_ATTR, DataView.DATAVIEW_SORT_INDICATOR_CLASS, null);
writer.endElement("i");
}
writer.endElement(HTML.TH_ELEM);
}
}
writer.endElement(HTML.TR_ELEM);
writer.endElement(HTML.THEAD_ELEM);
if (writeTable) writer.endElement(HTML.TABLE_ELEM);
}
private void encodeFooters(ResponseWriter writer,
DataViewColumnsModel columnModel,
DataViewDataModel dataModel, boolean writeTable) throws IOException {
if (writeTable) {
writer.startElement(HTML.TABLE_ELEM, null);
writer.writeAttribute(HTML.CLASS_ATTR, DataView.DATAVIEW_FOOT_CLASS, null);
}
writer.startElement(HTML.TFOOT_ELEM, null);
writer.startElement(HTML.TR_ELEM, null);
for (IndexedIterator columnIter = columnModel.iterator(); columnIter.hasNext();) {
DataViewColumnModel column = columnIter.next();
int index = columnIter.getIndex();
if (column.isRendered()) {
writer.startElement(HTML.TD_ELEM, null);
String className = getColumnStyleClass(column, index);
writer.writeAttribute(HTML.CLASS_ATTR, className, null);
if (column.getFooterText() != null)
writer.write(column.getFooterText());
writer.endElement(HTML.TD_ELEM);
}
}
writer.endElement(HTML.TR_ELEM);
writer.endElement(HTML.TFOOT_ELEM);
if (writeTable) writer.endElement(HTML.TABLE_ELEM);
}
private String getColumnStyleClass(DataViewColumnModel column, int index) {
String colStyleClass = column.getStyleClass();
String className = DataView.DATAVIEW_COLUMN_CLASS + "-" + index;
if (colStyleClass != null) className += " " + colStyleClass;
return className;
}
private void encodeRows(FacesContext context,
ResponseWriter writer,
DataView dataView,
String var,
DataViewColumnsModel columnModel,
DataViewDataModel dataModel) throws IOException {
String dvId = dataView.getClientId();
ELContext elContext = context.getELContext();
Map requestMap = context.getExternalContext().getRequestMap();
String clientId = dvId;
String bodyClass = DataView.DATAVIEW_BODY_CLASS;
if (dataView.isRowStroke()) bodyClass += " stroke";
if (dataView.isRowStripe()) bodyClass += " stripe";
writer.startElement(HTML.DIV_ELEM, null);
writer.writeAttribute(HTML.CLASS_ATTR, "overthrow", null);
writer.startElement(HTML.TABLE_ELEM, null);
writer.writeAttribute(HTML.CLASS_ATTR, bodyClass, null);
if (columnModel.hasHeaders()) encodeHeaders(writer, columnModel, dataModel, false);
writer.startElement(HTML.TBODY_ELEM, null);
List detailHolders = getDetailHolders(dataView.getDetails());
String rowIndexVar = dataView.getRowIndexVar();
Integer activeRowIndex = dataView.getActiveRowIndex();
int selectedRowIndex = activeRowIndex == null ? -1 : activeRowIndex;
for (IndexedIterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy