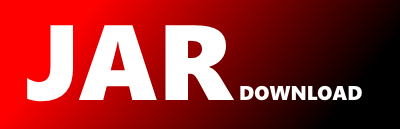
org.icij.extract.mysql.SQLConcurrentMap Maven / Gradle / Ivy
package org.icij.extract.mysql;
import javax.sql.DataSource;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Map;
import java.util.concurrent.ConcurrentMap;
public abstract class SQLConcurrentMap implements ConcurrentMap {
protected final FunctionalDataSource dataSource;
final SQLMapCodec codec;
SQLConcurrentMap(final DataSource dataSource, final SQLMapCodec codec) {
this.dataSource = FunctionalDataSource.cast(dataSource);
this.codec = codec;
}
public abstract boolean fastPut(final K key, final V value);
public class Entry implements Map.Entry {
private final K key;
private final V value;
Entry(final K key, final V value) {
this.key = key;
this.value = value;
}
@Override
public K getKey() {
return key;
}
@Override
public V getValue() {
return value;
}
@Override
public V setValue(final V value) {
final V old = this.value;
fastPut(key, value);
return old;
}
}
protected class EntrySetCodec implements SQLCodec> {
@Override
public Map encodeKey(final Object o) {
return codec.encodeKey(o);
}
@Override
public Entry decodeValue(final ResultSet rs) throws SQLException {
return new Entry(codec.decodeKey(rs), codec.decodeValue(rs));
}
@Override
public Map encodeValue(final Object o) {
return codec.encodeValue(o);
}
}
protected class KeySetCodec implements SQLCodec {
@Override
public Map encodeKey(final Object o) {
return codec.encodeKey(o);
}
@Override
public K decodeValue(final ResultSet rs) throws SQLException {
return codec.decodeKey(rs);
}
@Override
public Map encodeValue(final Object o) {
return codec.encodeValue(o);
}
}
protected class ValuesCodec implements SQLCodec {
@Override
public Map encodeKey(final Object o) {
return codec.encodeKey(o);
}
@Override
public V decodeValue(final ResultSet rs) throws SQLException {
return codec.decodeValue(rs);
}
@Override
public Map encodeValue(final Object o) {
return codec.encodeValue(o);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy