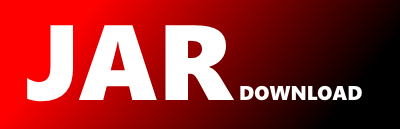
org.id4me.Id4meClaimsConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of relying-party-api Show documentation
Show all versions of relying-party-api Show documentation
ID4me RelyingParty API prototype
The newest version!
/*
*
* Copyright (C) 2018 Fonpit AG
*
*/
package org.id4me;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.id4me.config.Id4meClaimsParameters;
import org.id4me.config.Id4meClaimsParameters.Entry;
import org.json.JSONObject;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Unmodifiable ID4me claims configuration with serialization and validation
* methods.
*
* The functionality of this class has not been built into
* {@link Id4meClaimsParameters} for two reasons:
*
*
* SRP (single responsibility principle)
* so that the configuration cannot be changed after being passed to the
* {@link Id4meLogon} constructor
*
*
* @author Sven Woltmann
*/
class Id4meClaimsConfig {
private static final Logger log = LoggerFactory.getLogger(Id4meClaimsConfig.class);
private final transient List entries;
private final String claimsParam;
private final Set essentialClaims;
private final String[] profile = new String[] { "name", "family_name", "given_name", "middle_name", "nickname",
"preferred_username", "profile", "picture", "website", "gender", "birthdate", "zoneinfo", "locale",
"updated_at" };
private final String[] email = new String[] { "email", "email_verified" };
private final String[] address = new String[] { "address" };
private final String[] phone = new String[] { "phone_number", "phone_number_verified" };
private String scopes = "openid";
/**
* Builds the ID4me claims configuration based on the specified parameters.
*
* @param parameters the claims parameters
*/
Id4meClaimsConfig(Id4meClaimsParameters parameters) {
this.entries = Collections.unmodifiableList(new ArrayList<>(parameters.getEntries()));
this.claimsParam = buildClaimsParam();
log.info("Configured claims param: {}", claimsParam);
this.essentialClaims = buildEssentialClaims();
log.info("Configured essential claims: {}", essentialClaims);
}
private String buildClaimsParam() {
JSONObject userinfo = new JSONObject();
for (Entry entry : entries) {
JSONObject p = null;
if (entry.isEssential()) {
p = new JSONObject();
p.put("essential", true);
}
String reason = entry.getReason();
if (reason != null && !reason.equals("")) {
p = p == null ? new JSONObject() : p;
p.put("reason", reason);
}
if (p != null)
userinfo.put(entry.getName(), p);
else
userinfo.put(entry.getName(), JSONObject.NULL);
}
String info = "{\"userinfo\":" + userinfo.toString() + "}";
//TODO identity assurance
// info = "{\"userinfo\":{\"verified_claims\":{\"verification\": {\"trust_framework\": \"denicid_famework\"},\"claims\":{\"family_name\":{\"essential\": false}}}}}";
// info = "{\"userinfo\": {\"verified_claims\": {\"verification\": {\"trust_framework\": \"denicid_famework\",\"time\": null,\"evidence\": [{\"type\": {\"value\": \"id_document\"},\"method\": null,\"document\": {\"type\": null}}]},\"claims\": {\"given_name\": null,\"family_name\": null}}}}";
return info;
}
/**
* Returns the claims request parameter to be used in the authorize URI.
*
* @return the claims request parameter
*/
String getClaimsParam() {
return claimsParam;
}
/**
* Add scopes which contain requested claims. Is used when
* claims_parameter_supported is false AND fallbackToScopes is true at
* Id4meLogon.authorize(Id4meSessionData sessionData)
*/
String getScopesForClaims() {
JSONObject claims = new JSONObject(claimsParam);
JSONObject userinfo = claims.getJSONObject("userinfo");
String s = scopes;
for (String c : userinfo.keySet()) {
if (isInList(c, profile))
if (s.indexOf("profile") < 0)
s += " profile";
if (isInList(c, email))
if (s.indexOf("email") < 0)
s += " email";
if (isInList(c, address))
if (s.indexOf("address") < 0)
s += " address";
if (isInList(c, phone))
if (s.indexOf("phone") < 0)
s += " phone";
}
return s.trim();
}
boolean isInList(String v, String[] list) {
for (String e : list)
if (e.toLowerCase().equals(v.toLowerCase()))
return true;
return false;
}
/**
* Adds a scope permanently to the scopes for this {@link Id4meClaimsConfig}
*
* @param scope
*/
public void addScope(String scope) {
if (scopes.indexOf(scope) < 0)
scopes += " " + scope;
}
public String getScopesParam() {
return scopes;
}
private Set buildEssentialClaims() {
Set claims = new HashSet<>();
for (Entry entry : entries) {
if (entry.isEssential()) {
claims.add(entry.getName());
}
}
return Collections.unmodifiableSet(claims);
}
/**
* Returns a set of essential claim names.
*
* @return the set of essential claim names
*/
Set getEssentialClaims() {
return essentialClaims;
}
/**
* Checks if the specified claim is essential.
*
* @param claimName the claim name
*
* @return whether the specified claim is essential
*/
boolean isEssential(String claimName) {
return essentialClaims.contains(claimName);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy