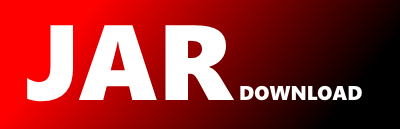
org.id4me.Id4meDnsResponseParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of relying-party-api Show documentation
Show all versions of relying-party-api Show documentation
ID4me RelyingParty API prototype
The newest version!
/*
*
* Copyright (C) 2018 Fonpit AG
*
*/
package org.id4me;
import java.net.IDN;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
*
* @author Sven Woltmann
*/
class Id4meDnsResponseParser {
private static final Logger log = LoggerFactory.getLogger(Id4meDnsResponseParser.class);
private Id4meDnsResponseParser() {
// hide implicit public constructor of utility class
}
static Id4meDnsData parseDnsResponse(String data) throws Exception {
String v = null;
String iau = null;
String iag = null;
String[] values = data.split(";");
for (String value : values) {
String[] e = value.trim().split("=");
if (e.length == 2) {
log.debug("DNS response: {} = \"{}\"", e[0], e[1]);
if ("v".equals(e[0])) {
if (v != null) {
log.warn("More than one v field found in TXT RR: {}", data);
throw new Exception("More than one v field found in TXT RR: " + data);
} else {
v = e[1].trim();
}
}
if ("iss".equals(e[0])) {
if (iau != null) {
log.warn("More than one iss field found in TXT RR: {}", data);
throw new Exception("More than one iss field found in TXT RR: " + data);
} else {
iau = e[1].trim();
iau = IDN.toASCII(iau);
}
}
/* CLP discovery removed
if ("clp".equals(e[0])) {
if (iag != null) {
log.warn("More than one clp field found in TXT RR: {}", data);
throw new Exception("More than one clp field found in TXT RR: " + data);
} else {
iag = e[1].trim();
iag = IDN.toASCII(iag);
}
}
*/
if ("iau".equals(e[0])) {
if (iau != null) {
log.warn("More than one iss field found in TXT RR: {}", data);
throw new Exception("More than one iss field found in TXT RR: " + data);
} else {
iau = e[1].trim();
iau = IDN.toASCII(iau);
}
}
if ("iag".equals(e[0])) {
if (iag != null) {
log.warn("More than one clp field found in TXT RR: {}", data);
throw new Exception("More than one clp field found in TXT RR: " + data);
} else {
iag = e[1].trim();
iag = IDN.toASCII(iag);
}
}
}
}
validateParameters(v, iau, iag);
return buildDataObject(v, iau, iag);
}
private static void validateParameters(String v, String iau, String iag) throws Exception {
if (!"OID1".equals(v)) {
log.warn("Error getting domain-id data from DNS: version != \"OID1\"");
throw new Exception("Error getting domain-id data from DNS: version != \"OID1\"");
} else if (iau == null) {
log.warn("Error getting domain-id data from DNS: iss missing");
throw new Exception("Error getting domain-id data from DNS: iss missing");
}
/*
* else if (iag == null) {
* log.warn("Error getting domain-id data from DNS: clp missing"); throw new
* Exception("Error getting domain-id data from DNS: clp missing"); }
*/
}
private static Id4meDnsData buildDataObject(String v, String iau, String iag) {
iau = removeTrailingSlash(iau);
iag = removeTrailingSlash(iag);
return new Id4meDnsData(v, iau, iag);
}
private static String removeTrailingSlash(String s) {
if (s == null) {
return s;
}
return s.endsWith("/") ? s.substring(0, s.length() - 1) : s;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy