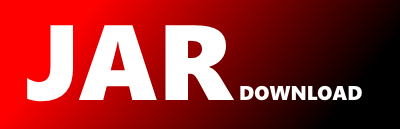
org.id4me.Id4meIdentityAuthorityStorage2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of relying-party-api Show documentation
Show all versions of relying-party-api Show documentation
ID4me RelyingParty API prototype
The newest version!
/*
* Copyright (C) 2016-2020 OX Software GmbH
* Developed by Peter Höbel [email protected]
* See the LICENSE file for licensing conditions
* SPDX-License-Identifier: MIT
*/
package org.id4me;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.Writer;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardCopyOption;
import org.id4me.util.FileReader;
import org.json.JSONObject;
/**
* This class implements a simple file store for the relying party registration
* data. Every identity authority has one file in the file system where the
* registration data as JSON string is saved. For faster access to the data this
* data is mirrored in a hash map which is queried first.
*
* @author phoebel
*
*/
class Id4meIdentityAuthorityStorage2 implements Id4meIdentityAuthorityStorage {
static final Id4meIdentityAuthorityStorage INSTANCE = new Id4meIdentityAuthorityStorage2();
private Id4meIdentityAuthorityStorage2() {
}
boolean hasIauData(String iau) {
return storage.containsKey(iau);
}
/**
* Return the registration data for an identity authority.
*
* @param iau the identity authority domain
* @return Id4meIdentityAuthorityData, or null if not found.
* @throws FileNotFoundException if the file was not found
* @throws IOException if any other I/O error occurred
*/
public Id4meIdentityAuthorityData getIauData(Path authorityPath, String iau) throws IOException {
Path path = buildIauFilePath(authorityPath, iau);
if (storage.containsKey(path.toString()))
return storage.get(path.toString());
if (loadIauDataFromFile(authorityPath, iau)) {
return storage.get(path.toString());
}
return null;
}
/**
* Load the registration data from file.
*
* @param iau the identity authority domain
* @return true, if the file exists and contains data, otherwise false
* @throws FileNotFoundException if the file was not found
* @throws IOException if any other I/O error occurred
*/
public boolean loadIauDataFromFile(Path authorityPath, String iau) throws IOException {
Path path = buildIauFilePath(authorityPath, iau);
// Not using Files.exists(..) because of poor performance in JDK 8
if (path.toFile().exists()) {
String json = FileReader.readFileToString(path);
if (json.length() > 0) {
JSONObject registrationJson = new JSONObject(json);
Id4meIdentityAuthorityData data = storage.computeIfAbsent(path.toString(),
k -> new Id4meIdentityAuthorityData());
data.setIau(iau);
data.setClientId(registrationJson.getString("client_id"));
data.setClientSecret(registrationJson.getString("client_secret"));
data.setRegistrationData(registrationJson);
storage.put(path.toString(), data);
return true;
}
}
return false;
}
/**
* Delete the registration data file if any exists and remove the data from the
* hash map.
*
* @param iau the identity authority domain
*/
public void removeIauData(Path authorityPath, String iau) throws IOException {
Path path = buildIauFilePath(authorityPath, iau);
Files.deleteIfExists(path);
storage.remove(path.toString());
}
/**
* Save the registration data to a file and in a private Hastable.
*
* @param iau the identity authority domain
* @param registrationData the registration data as JSONObject
* @return Id4meIdentityAuthorityData or null if not available
* @throws IOException
*/
public Id4meIdentityAuthorityData saveRegistrationData(Path authorityPath, String iau, JSONObject registrationData)
throws IOException {
Path tmpPath = buildIauTmpFilePath(authorityPath, iau);
Path path = buildIauFilePath(authorityPath, iau);
ensurePathExists(tmpPath);
log.info("Saving registration data in iau data file: {}", path);
try (Writer writer = Files.newBufferedWriter(tmpPath)) {
writer.write(registrationData.toString(2));
writer.flush();
// make the creation of the file an atomic operation
Files.move(tmpPath, path, StandardCopyOption.ATOMIC_MOVE);
}
Id4meIdentityAuthorityData data = storage.get(path.toString());
if (data == null)
data = new Id4meIdentityAuthorityData();
data.setIau(iau);
data.setClientId(registrationData.getString("client_id"));
data.setClientSecret(registrationData.getString("client_secret"));
data.setRegistrationData(registrationData);
storage.put(path.toString(), data);
return data;
}
/**
* Ensures that the directories for the authority-data.json are created if the
* identity authority from the dns field iss contains a folder. For example, if
* the iss definition in dns is "iss=myidentityauthority.org/testdir", the
* directory "myidentityauthority.org" will be created.
*
* @param the complete authority file path
*/
private void ensurePathExists(Path path) {
Path parent = path.getParent();
if (parent != null && !parent.toFile().exists()) {
parent.toFile().mkdirs();
}
}
private Path buildIauFilePath(Path authorityPath, String iau) {
return authorityPath.resolve(iau + ".json");
}
private Path buildIauTmpFilePath(Path authorityPath, String iau) {
return authorityPath.resolve(iau + ".json.tmp");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy