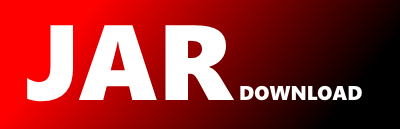
org.id4me.Id4meKeyPairHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of relying-party-api Show documentation
Show all versions of relying-party-api Show documentation
ID4me RelyingParty API prototype
The newest version!
package org.id4me;
import java.io.DataInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.security.KeyFactory;
import java.security.KeyPair;
import java.security.PrivateKey;
import java.security.PublicKey;
import java.security.interfaces.RSAPublicKey;
import java.security.spec.PKCS8EncodedKeySpec;
import java.security.spec.X509EncodedKeySpec;
import java.util.Base64;
import java.util.UUID;
import org.json.JSONArray;
import org.json.JSONObject;
import com.nimbusds.jose.jwk.JWK;
import com.nimbusds.jose.jwk.KeyUse;
import com.nimbusds.jose.jwk.RSAKey;
/**
* Simple class to handle public and private key to request encrypted token at
* the client registration and decrypt the encrypted id token from the identity
* authority . The keys could be created by the following commands: openssl
* genrsa -out id4me.rsa.pem 4096 openssl rsa -pubout -in id4me.rsa.pem -out
* id4me.pub.key.pem openssl pkcs8 -topk8 -inform PEM -outform PEM -in
* id4me.rsa.pem -out id4me.priv.key.pem -nocrypt
*
* The resulting id4me.priv.key.pem and id4me.pub.key.pem files should be used
* to instantiate a Id4meKeyPairHandler
*
* @author Peter Hoebel
*
*/
class Id4meKeyPairHandler {
private KeyPair keyPair = null;
private String pub_key_file;
private String priv_key_file;
public Id4meKeyPairHandler(String pub_key_file, String priv_key_file) throws Exception {
this.priv_key_file = priv_key_file;
this.pub_key_file = pub_key_file;
PublicKey pub_key = getPemPublicKey(pub_key_file);
PrivateKey priv_key = getPemPrivateKey(priv_key_file);
keyPair = new KeyPair(pub_key, priv_key);
}
public String toString() {
return pub_key_file + ", " + priv_key_file;
}
public KeyPair getKeyPair() {
return keyPair;
}
public JSONObject generateJwks() throws Exception {
JWK jwk = generateJwk(keyPair);
JSONObject jwks = new JSONObject();
JSONArray keys = new JSONArray();
JSONObject jwk_json = new JSONObject(jwk.toJSONString());
jwk_json.remove("use");
keys.put(jwk_json);
jwks.put("keys", keys);
return jwks;
}
private JWK generateJwk(KeyPair keyPair) throws Exception {
// Convert to JWK format
JWK jwk = new RSAKey.Builder((RSAPublicKey) keyPair.getPublic()).keyUse(KeyUse.SIGNATURE)
.keyID(UUID.randomUUID().toString()).build();
return jwk;
}
/**
* Create a {@link PrivateKey} from pem file.
*
* @param path to the private key .pem file
* @return {@link java.security.PrivateKey}
* @throws Exception
*/
public PrivateKey getPemPrivateKey(String filename) throws Exception {
String algorithm = "RSA";
File f = new File(filename);
FileInputStream fis = new FileInputStream(f);
DataInputStream dis = new DataInputStream(fis);
byte[] keyBytes = new byte[(int) f.length()];
dis.readFully(keyBytes);
dis.close();
String temp = new String(keyBytes);
String privKeyPEM = temp.replace("-----BEGIN PRIVATE KEY-----\n", "");
privKeyPEM = privKeyPEM.replace("-----END PRIVATE KEY-----", "");
byte[] decoded = Base64.getDecoder().decode(privKeyPEM);
PKCS8EncodedKeySpec spec = new PKCS8EncodedKeySpec(decoded);
KeyFactory kf = KeyFactory.getInstance(algorithm);
return kf.generatePrivate(spec);
}
/**
* Create a {@link PublicKey} from pem file.
*
* @param path to the private key .pem file
* @return {@link java.security.PublicKey}
* @throws Exception
*/
public PublicKey getPemPublicKey(String filename) throws Exception {
String algorithm = "RSA";
File f = new File(filename);
FileInputStream fis = new FileInputStream(f);
DataInputStream dis = new DataInputStream(fis);
byte[] keyBytes = new byte[(int) f.length()];
dis.readFully(keyBytes);
dis.close();
String temp = new String(keyBytes);
String publicKeyPEM = temp.replace("-----BEGIN PUBLIC KEY-----\n", "");
publicKeyPEM = publicKeyPEM.replace("-----END PUBLIC KEY-----", "");
byte[] decoded = Base64.getDecoder().decode(publicKeyPEM);
X509EncodedKeySpec spec = new X509EncodedKeySpec(decoded);
KeyFactory kf = KeyFactory.getInstance(algorithm);
return kf.generatePublic(spec);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy