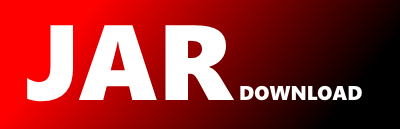
org.id4me.Id4meSessionData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of relying-party-api Show documentation
Show all versions of relying-party-api Show documentation
ID4me RelyingParty API prototype
The newest version!
/*
* Copyright (C) 2016-2020 OX Software GmbH
* Developed by Peter Höbel [email protected]
* See the LICENSE file for licensing conditions
* SPDX-License-Identifier: MIT
*/
package org.id4me;
import java.util.UUID;
import org.json.JSONObject;
/**
* Id4meSessionData holds any data used by {@link Id4meLogon} to logon an user
* on an Id4ME identity authority and to requests the userinfo from an
* Id4ME identity agent.
*
* @author Peter Hoebel
*
*/
public class Id4meSessionData {
private String identityHandle;
private String id4me = null;
private String redirectUri = "";
private String[] redirectUris = null;
private String logoUri = "";
private String loginHint = "";
private String state = "authorize";
private String nonce;
private String scope = "openid";
private boolean standardClaimsValidated = false;
private JSONObject bearerToken = null;
private String accessToken = null;
private String idToken = null;
private JSONObject userinfo = null;
private JSONObject idTokenUserinfo = null;
private JSONObject accessTokenUserinfo = null;
private String iau = null;
private String iag = null;
private Long tokenExpires = 0L;
private Id4meIdentityAuthorityData iauData;
Id4meSessionData() {
this.nonce = UUID.randomUUID().toString().replace("-", "");
}
public JSONObject getBearerToken() {
return bearerToken;
}
public JSONObject getIdTokenUserinfo() {
return idTokenUserinfo;
}
public void setIdTokenUserinfo(JSONObject idTokenUserinfo) {
this.idTokenUserinfo = idTokenUserinfo;
}
public JSONObject getAccessTokenUserinfo() {
return accessTokenUserinfo;
}
public void setAccessTokenUserinfo(JSONObject accessTokenUserinfo) {
this.accessTokenUserinfo = accessTokenUserinfo;
}
public void setBearerToken(JSONObject bearerToken) {
this.bearerToken = bearerToken;
}
public String getIdToken() {
return idToken;
}
public void setIdToken(String idToken) {
this.idToken = idToken;
}
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
/**
* Returns the unique identity handle consists of the value of the iss claim +
* "#"
+ the value of the sub claim of the access-token
*
* @return String the unique identity handle
*/
public String getIdentityHandle() {
return identityHandle;
}
void setIdentityHandle(String identityHandle) {
this.identityHandle = identityHandle;
}
public String getId4me() {
return id4me;
}
void setId4me(String id4me) {
this.id4me = id4me;
}
public String getLoginHint() {
return loginHint;
}
public String getLogoUri() {
return logoUri;
}
void setLogoUri(String logoUri) {
this.logoUri = logoUri;
}
void setLoginHint(String loginHint) {
this.loginHint = loginHint;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public String getNonce() {
return nonce;
}
void setNonce(String nonce) {
this.nonce = nonce;
}
public String getScope() {
return scope;
}
void setScope(String scope) {
this.scope = scope;
}
public String getRedirectUri() {
return redirectUri;
}
void setRedirectUri(String redirectUri) {
this.redirectUri = redirectUri;
}
public JSONObject getUserinfo() {
return userinfo;
}
void setUserinfo(JSONObject userinfo) {
this.userinfo = userinfo;
}
public String getIag() {
return iag;
}
void setIag(String iag) {
this.iag = iag;
}
public long getTokenExpires() {
if (tokenExpires == null)
return 0;
return tokenExpires;
}
void setTokenExpires(Long expires) {
this.tokenExpires = expires;
}
public String getIau() {
return iau;
}
void setIau(String iau) {
this.iau = iau;
}
Id4meIdentityAuthorityData getIauData() {
return iauData;
}
void setIauData(Id4meIdentityAuthorityData iauData) {
this.iauData = iauData;
}
public String[] getRedirectUris() {
return redirectUris;
}
public void setRedirectUris(String[] redirectUris) {
this.redirectUris = redirectUris;
}
public boolean isStandardClaimsValidated() {
return standardClaimsValidated;
}
public void setStandardClaimsValidated(boolean standardClaimsValidated) {
this.standardClaimsValidated = standardClaimsValidated;
}
public String getAccessToken() {
return accessToken;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy