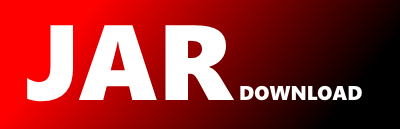
org.iherus.shiro.cache.redis.AbstractRedisOperations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shiro-redis Show documentation
Show all versions of shiro-redis Show documentation
Shiro cache Integration Adapter.
/**
* Copyright (c) 2016-2019, Bosco.Liao ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.iherus.shiro.cache.redis;
import java.util.concurrent.atomic.AtomicReference;
import java.util.function.Function;
import java.util.function.Supplier;
import org.apache.shiro.util.ClassUtils;
import org.iherus.shiro.cache.redis.connection.RedisConnection;
import org.iherus.shiro.cache.redis.connection.RedisConnectionFactory;
import org.iherus.shiro.cache.redis.serializer.DefaultSerializer;
import org.iherus.shiro.cache.redis.serializer.FstSerializer;
import org.iherus.shiro.cache.redis.serializer.ValueSerializer;
import org.iherus.shiro.exception.ExecutionException;
/**
* 根据FST依赖包的引入与否,将FstSerializer设定为默认的ValueSerializer
*
* @author Bosco.Liao
* @since 2.0.0
*/
public abstract class AbstractRedisOperations implements RedisOperations {
private static final Supplier VS_SUPPLIER = new Supplier() {
final AtomicReference vsRef = new AtomicReference<>();
@Override
public ValueSerializer get() {
ValueSerializer serializer = vsRef.get();
if (serializer != null)
return serializer;
serializer = ClassUtils.isAvailable("org.nustaq.serialization.FSTConfiguration") ? new FstSerializer()
: DefaultSerializer.INSTANCE;
return vsRef.compareAndSet(null, serializer) ? serializer : vsRef.get();
}
};
/**
* If the {@literal FST.jar} is added, the {@literal FstSerializer} will be the default Serializer.
*/
@Override
public ValueSerializer getValueSerializer() {
return VS_SUPPLIER.get();
}
/**
* Implementation of {@link RedisOperations}.
*/
static class DefaultRedisOperations extends AbstractRedisOperations {
private final RedisConnectionFactory connectionFactory;
DefaultRedisOperations(RedisConnectionFactory connectionFactory) {
this.connectionFactory = connectionFactory;
}
@Override
public RedisConnectionFactory getConnectionFactory() {
return connectionFactory;
}
public RedisConnectionFactory getRequiredConnectionFactory() {
RedisConnectionFactory connectionFactory = getConnectionFactory();
if (connectionFactory == null) {
throw new IllegalStateException("RedisConnectionFactory is required");
}
return connectionFactory;
}
@Override
public T execute(Function action) throws ExecutionException {
RedisConnectionFactory factory = getRequiredConnectionFactory();
RedisConnection connection = null;
try {
connection = factory.getConnection();
return action.apply(connection);
} catch (Exception e) {
throw new ExecutionException("Unable to execute redis command normally.", e);
} finally {
connection.close();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy