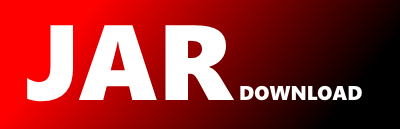
org.iherus.shiro.cache.redis.config.RedisClusterConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shiro-redis Show documentation
Show all versions of shiro-redis Show documentation
Shiro cache Integration Adapter.
/**
* Copyright (c) 2016-2019, Bosco.Liao ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.iherus.shiro.cache.redis.config;
import static org.iherus.shiro.util.Utils.*;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Set;
import org.iherus.shiro.cache.redis.config.RedisConfiguration.ClusterConfiguration;
public class RedisClusterConfiguration implements RedisConfiguration, ClusterConfiguration {
private static final int DEFAULT_MAX_ATTEMPS = 5;
private Set clusterNodes;
private int maxAttempts;
private String password;
public RedisClusterConfiguration() {
this.maxAttempts = DEFAULT_MAX_ATTEMPS;
this.clusterNodes = new LinkedHashSet();
}
@Override
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
@Override
public Set getClusterNodes() {
return Collections.unmodifiableSet(clusterNodes);
}
public void setClusterNodes(Set clusterNodes) {
this.clusterNodes = clusterNodes;
}
public void setClusterNodesFromText(String clusterNodes) {
String nodesToUse = isNotBlank(clusterNodes) ? clusterNodes.replace(" ", "") : clusterNodes;
this.addClusterNodes(commaDelimitedListToSet(nodesToUse));
}
@Override
public int getMaxAttempts() {
return maxAttempts;
}
public void setMaxAttempts(int maxAttempts) {
this.maxAttempts = Math.max(maxAttempts, 0);
}
/**
* Add cluster node.
*
* @param node must not be {@literal null}.
*/
public void addClusterNode(HostPortPair node) {
assertNotNull(node, "Cluster node must not be 'null'.");
this.clusterNodes.add(node);
}
private void addClusterNodes(Set hostAndPorts) {
for (String hostAndPort : hostAndPorts) {
this.addClusterNode(readHostAndPortFromString(hostAndPort));
}
}
private HostPortPair readHostAndPortFromString(String hostAndPort) {
String[] args = split(hostAndPort, ":");
assertNotNull(args, "HostAndPort need to be seperated by ':'.");
assertIsTrue(args.length == 2, () -> "Host and Port String needs to specified as host:port");
return new HostPortPair(args[0], Integer.valueOf(args[1]).intValue());
}
@Override
public Set getAddresses(boolean ssl) {
return HostPortPair.convertToStringPairs(getClusterNodes(), true, ssl);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy