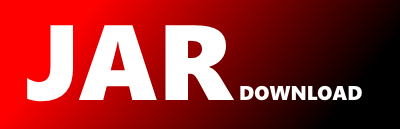
org.iherus.shiro.cache.redis.serializer.FstSerializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shiro-redis Show documentation
Show all versions of shiro-redis Show documentation
Shiro cache Integration Adapter.
/**
* Copyright (c) 2016-2019, Bosco.Liao ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.iherus.shiro.cache.redis.serializer;
import static org.iherus.shiro.util.Utils.isEmpty;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.Serializable;
import org.apache.shiro.io.SerializationException;
import org.nustaq.serialization.FSTConfiguration;
import org.nustaq.serialization.FSTObjectInput;
import org.nustaq.serialization.FSTObjectOutput;
/**
* FST序相比Java原生序列化,具有更好的性能和更小的体积,建议使用
*
* 注意:当引入热部署组件:spring-boot-devtools,FST序列化会抛异常:java.lang.ClassCastException。
*
* @author Bosco.Liao
* @since 2.0.0
*/
public class FstSerializer extends ValueSerializer {
private final FSTConfiguration conf;
public FstSerializer() {
super();
this.conf = FSTConfiguration.createDefaultConfiguration();
}
@Override
public byte[] serialize(Object object) throws SerializationException {
if (object == null) {
return EMPTY_ARRAY;
}
if (!(object instanceof Serializable)) {
throw new IllegalArgumentException(getClass().getSimpleName() + " requires a Serializable payload "
+ "but received an object of type [" + object.getClass().getName() + "]");
}
ByteArrayOutputStream out = new ByteArrayOutputStream(DEFAULT_BUFFER_SIZE);
FSTObjectOutput objectOutput = conf.getObjectOutput(out);
try {
objectOutput.writeObject(object);
return objectOutput.getCopyOfWrittenBuffer();
} catch (Exception e) {
throw new SerializationException("Failed to serialize object of type: " + object.getClass().getName(), e);
} finally {
try {
objectOutput.flush();
out.close();
} catch (Exception ex) {
// ignored
}
}
}
@Override
public Object deserialize(byte[] serialized) throws SerializationException {
if (isEmpty(serialized)) {
return null;
}
ByteArrayInputStream inputStream = new ByteArrayInputStream(serialized);
FSTObjectInput objectInput = conf.getObjectInput(inputStream);
try {
return objectInput.readObject();
} catch (Exception e) {
throw new SerializationException(
"Failed to deserialize payload. " + "Is the byte array a result of corresponding serialization for "
+ this.getClass().getSimpleName() + "?", e);
} finally {
try {
inputStream.close();
} catch (IOException e) {
// ignored
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy