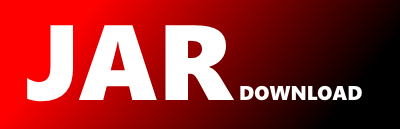
org.ikasan.dashboard.ui.scheduler.component.AgentWidget Maven / Gradle / Ivy
package org.ikasan.dashboard.ui.scheduler.component;
import com.vaadin.flow.component.ComponentEventListener;
import com.vaadin.flow.component.UI;
import com.vaadin.flow.component.grid.ItemDoubleClickEvent;
import com.vaadin.flow.component.html.Div;
import com.vaadin.flow.component.html.H4;
import com.vaadin.flow.component.icon.Icon;
import com.vaadin.flow.component.icon.VaadinIcon;
import com.vaadin.flow.component.orderedlayout.FlexComponent;
import com.vaadin.flow.component.orderedlayout.HorizontalLayout;
import com.vaadin.flow.component.textfield.TextField;
import com.vaadin.flow.data.renderer.TemplateRenderer;
import org.ikasan.dashboard.ui.util.SystemEventLogger;
import org.ikasan.dashboard.ui.visualisation.component.filter.ModuleSearchFilter;
import org.ikasan.scheduled.event.service.ScheduledProcessManagementService;
import org.ikasan.spec.metadata.ModuleMetaData;
import org.ikasan.spec.metadata.ModuleMetaDataService;
import org.ikasan.spec.module.client.ConfigurationService;
import org.ikasan.spec.module.client.DownloadLogFileService;
import org.ikasan.spec.module.client.MetaDataService;
import org.ikasan.spec.module.client.ModuleControlService;
import org.ikasan.spec.scheduled.context.service.ScheduledContextService;
import org.ikasan.spec.scheduled.general.SchedulerService;
import org.ikasan.spec.scheduled.job.service.SchedulerJobService;
import org.ikasan.spec.scheduled.provision.JobProvisionService;
import java.util.Map;
public class AgentWidget extends Div {
private ScheduledAgentsFilteringGrid scheduledAgentsFilteringGrid;
private ModuleMetaDataService moduleMetadataService;
private ScheduledProcessManagementService scheduledProcessManagementService;
private TextField filterTextField;
private ConfigurationService configurationRestService;
private ModuleControlService moduleControlRestService;
private MetaDataService metaDataRestService;
private SystemEventLogger systemEventLogger;
private SchedulerService schedulerService;
private SchedulerJobService schedulerJobService;
private Map schedulerJobExecutionEnvironmentLabel;
private DownloadLogFileService downloadLogFileService;
private ScheduledContextService scheduledContextService;
private JobProvisionService jobProvisionService;
public AgentWidget(ModuleMetaDataService moduleMetadataService, ScheduledProcessManagementService scheduledProcessManagementService,
ConfigurationService configurationRestService, ModuleControlService moduleControlRestService, MetaDataService metaDataRestService,
SystemEventLogger systemEventLogger, SchedulerService schedulerService, JobProvisionService jobProvisionService, SchedulerJobService schedulerJobService,
DownloadLogFileService downloadLogFileService, ScheduledContextService scheduledContextService) {
this.moduleMetadataService = moduleMetadataService;
this.scheduledProcessManagementService = scheduledProcessManagementService;
this.configurationRestService = configurationRestService;
this.moduleControlRestService = moduleControlRestService;
this.metaDataRestService = metaDataRestService;
this.systemEventLogger = systemEventLogger;
this.schedulerService = schedulerService;
this.schedulerJobService = schedulerJobService;
this.jobProvisionService = jobProvisionService;
this.downloadLogFileService = downloadLogFileService;
this.scheduledContextService = scheduledContextService;
this.filterTextField = new TextField();
this.createGrid();
Div div = new Div();
div.addClassNames("card-counter");
div.setHeight("500px");
Icon icon = VaadinIcon.SEARCH.create();
icon.setSize("12pt");
filterTextField.setPrefixComponent(icon);
filterTextField.setId("filterTextField");
HorizontalLayout layout = new HorizontalLayout();
H4 modules = new H4(getTranslation("header.scheduler-agents", UI.getCurrent().getLocale()));
layout.add(modules, filterTextField);
layout.setVerticalComponentAlignment(FlexComponent.Alignment.START, modules);
layout.setVerticalComponentAlignment(FlexComponent.Alignment.END, filterTextField);
filterTextField.getElement().getStyle().set("margin-left", "auto");
div.add(layout);
div.add(this.scheduledAgentsFilteringGrid);
this.scheduledAgentsFilteringGrid.init();
this.add(div);
}
private void createGrid() {
// Create a modulesGrid bound to the list
ModuleSearchFilter moduleSearchFilter = new ModuleSearchFilter();
this.scheduledAgentsFilteringGrid = new ScheduledAgentsFilteringGrid(this.moduleMetadataService, moduleSearchFilter);
this.scheduledAgentsFilteringGrid.setId("scheduledAgentsFilteringGrid");
this.scheduledAgentsFilteringGrid.removeAllColumns();
this.scheduledAgentsFilteringGrid.setVisible(true);
this.scheduledAgentsFilteringGrid.setWidthFull();
this.scheduledAgentsFilteringGrid.setHeight("80%");
scheduledAgentsFilteringGrid.addColumn(ModuleMetaData::getName)
.setHeader(getTranslation("table-header.module-name", UI.getCurrent().getLocale())).setKey("name")
.setFlexGrow(16);
scheduledAgentsFilteringGrid.addColumn(TemplateRenderer.of("[[item.description]]")
.withProperty("description", ModuleMetaData::getDescription))
.setHeader(getTranslation("table-header.module-description", UI.getCurrent().getLocale()))
.setKey("description")
.setFlexGrow(32);;
this.scheduledAgentsFilteringGrid.addGridFiltering(filterTextField, moduleSearchFilter::setModuleNameFilter);
this.scheduledAgentsFilteringGrid.addItemDoubleClickListener((ComponentEventListener>) moduleMetaDataItemDoubleClickEvent -> {
SchedulerAgentManagementDialog schedulerAgentManagementDialog
= new SchedulerAgentManagementDialog(moduleMetaDataItemDoubleClickEvent.getItem(), downloadLogFileService
, this.scheduledContextService, this.jobProvisionService, this.schedulerJobService);
schedulerAgentManagementDialog.open();
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy