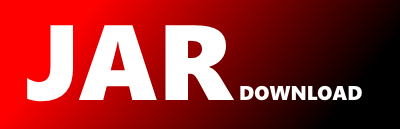
org.ikasan.flow.visitorPattern.FlowElementImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ikasan-flow-visitorPattern Show documentation
Show all versions of ikasan-flow-visitorPattern Show documentation
Ikasan EIP Flow implementation based on the Visitor design pattern
The newest version!
/*
* $Id$
* $URL$
*
* ====================================================================
* Ikasan Enterprise Integration Platform
*
* Distributed under the Modified BSD License.
* Copyright notice: The copyright for this software and a full listing
* of individual contributors are as shown in the packaged copyright.txt
* file.
*
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* - Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* - Neither the name of the ORGANIZATION nor the names of its contributors may
* be used to endorse or promote products derived from this software without
* specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE
* USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
* ====================================================================
*/
package org.ikasan.flow.visitorPattern;
import org.ikasan.spec.flow.FlowElement;
import org.ikasan.spec.flow.FlowElementInvoker;
import java.util.LinkedHashMap;
import java.util.Map;
/**
* Simple implementation of FlowElement
*
* @author Ikasan Development Team
*/
public class FlowElementImpl implements FlowElement
{
/** FlowComponent
being wrapped and given flow context */
private COMPONENT flowComponent;
/** Flow context specific name for the wrapped component */
private String componentName;
/** Map
of all flowComponent results to downstream FlowElement
s */
private Map transitions;
/** invoker for this flow element */
private FlowElementInvoker flowElementInvoker;
/**
* Human readable description of this FlowElement
*/
private String description;
/**
* The configured resource id.
*/
private String configuredResourceId;
/**
* Constructor for when there are more than one subsequent FlowElement
s
*
* @param componentName The name of the component
* @param flowComponent The FlowComponent
* @param transitions A map of transitions
*/
public FlowElementImpl(String componentName, COMPONENT flowComponent, FlowElementInvoker flowElementInvoker, Map transitions)
{
this.componentName = componentName;
this.flowComponent = flowComponent;
this.flowElementInvoker = flowElementInvoker;
this.transitions = transitions;
}
/**
* Overloaded constructor for when there is at most one subsequent FlowElement
*
* @param componentName The name of the component
* @param flowComponent The FlowComponent
* @param defaultTransition The default transition
*/
public FlowElementImpl(String componentName, COMPONENT flowComponent, FlowElementInvoker flowElementInvoker, FlowElement defaultTransition)
{
this(componentName, flowComponent, flowElementInvoker, createTransitionMap(defaultTransition));
}
/**
* Overloaded constructor for a FlowElement
with no downstream
*
* @param componentName The name of the component
* @param flowComponent The FlowComponent
*/
public FlowElementImpl(String componentName, COMPONENT flowComponent, FlowElementInvoker flowElementInvoker)
{
this(componentName, flowComponent, flowElementInvoker, (Map) null);
}
/**
* Creates the transition map when there is just the default transition
*
* @param defaultTransition The default transition
* @return Map mapping "default" to the specified FlowElement
*/
private static Map createTransitionMap(FlowElement defaultTransition)
{
Map defaultTransitions = new LinkedHashMap<>();
defaultTransitions.put(DEFAULT_TRANSITION_NAME, defaultTransition);
return defaultTransitions;
}
/*
* (non-Javadoc)
*
* @see flow.FlowElement#getFlowComponent()
*/
public COMPONENT getFlowComponent()
{
return flowComponent;
}
/*
* (non-Javadoc)
*
* @see flow.FlowElement#getComponentName()
*/
public String getComponentName()
{
return componentName;
}
/*
* (non-Javadoc)
*
* @see flow.FlowElement#getTransition(java.lang.String)
*/
public FlowElement getTransition(String transitionName)
{
FlowElement result = null;
if (transitions != null)
{
result = transitions.get(transitionName);
}
return result;
}
/* (non-Javadoc)
* @see org.ikasan.framework.flow.FlowElement#getDescription()
*/
public String getDescription()
{
return description;
}
/**
* Setter for description
*
* @param description
*/
public void setDescription(String description)
{
this.description = description;
}
@Override
public String toString()
{
StringBuilder sb = new StringBuilder(getClass().getName() + " [");
sb.append("name=");
sb.append(componentName);
sb.append(",");
sb.append("flowComponent=");
sb.append(flowComponent);
sb.append(",");
sb.append("transitions=");
sb.append(transitions);
sb.append("]");
return sb.toString();
}
/**
* Getter for the flow element invoker
* @return
*/
@Override
public FlowElementInvoker getFlowElementInvoker()
{
return this.flowElementInvoker;
}
/**
* Setter for the flow element invoker
* @param flowElementInvoker
*/
@Override
public void setFlowElementInvoker(FlowElementInvoker flowElementInvoker)
{
this.flowElementInvoker = flowElementInvoker;
}
public Map getTransitions()
{
Map result = new LinkedHashMap<>();
if (transitions != null)
{
result.putAll(transitions);
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy