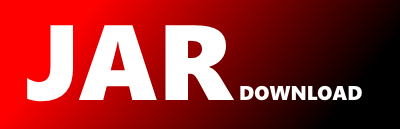
org.ikasan.scheduled.job.model.SolrSchedulerJobImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ikasan-solr-client Show documentation
Show all versions of ikasan-solr-client Show documentation
Ikasan EIP Solr Client Abstraction
The newest version!
package org.ikasan.scheduled.job.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
import org.ikasan.spec.scheduled.job.model.SchedulerJob;
import java.util.*;
public class SolrSchedulerJobImpl implements SchedulerJob {
protected String jobIdentifier;
protected String agentName;
protected String jobName;
protected String displayName;
protected String jobDescription;
protected String contextName;
protected List childContextNames;
protected String startupControlType = "AUTOMATIC";
@JsonIgnore
protected boolean skip = false;
protected Map skippedContexts = new HashMap<>();
protected Map heldContexts = new HashMap<>();
protected int ordinal = -1;
protected Boolean templateJob;
protected Boolean templateBased;
protected String templateName;
@Override
public String getContextName() {
return this.contextName;
}
@Override
public void setContextName(String contextName) {
this.contextName = contextName;
}
public List getChildContextNames() {
if(childContextNames == null) {
childContextNames = new ArrayList<>();
}
return childContextNames;
}
public void setChildContextNames(List childContextNames) {
this.childContextNames = childContextNames;
}
@Override
public String getIdentifier() {
return this.jobIdentifier;
}
@Override
public void setIdentifier(String jobIdentifier) {
this.jobIdentifier = jobIdentifier;
}
@Override
public String getAgentName() {
return this.agentName;
}
@Override
public void setAgentName(String agentName) {
this.agentName = agentName;
}
@Override
public String getJobName() {
return this.jobName;
}
@Override
public void setJobName(String jobName) {
this.jobName = jobName;
}
@Override
public String getDisplayName() {
return displayName;
}
@Override
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
@Override
public String getJobDescription() {
return this.jobDescription;
}
@Override
public void setJobDescription(String jobDescription) {
this.jobDescription = jobDescription;
}
@Override
public String getStartupControlType() {
return startupControlType;
}
@Override
public void setStartupControlType(String startupControlType) {
this.startupControlType = startupControlType;
}
@JsonIgnore
public boolean isSkip() {
return skip;
}
@JsonIgnore
public void setSkip(boolean skip) {
this.skip = skip;
}
@Override
public Map getSkippedContexts() {
return skippedContexts;
}
@Override
public void setSkippedContexts(Map skippedContexts) {
this.skippedContexts = skippedContexts;
}
@Override
public Map getHeldContexts() {
return heldContexts;
}
@Override
public void setHeldContexts(Map heldContexts) {
this.heldContexts = heldContexts;
}
@Override
public int getOrdinal() {
return ordinal;
}
@Override
public void setOrdinal(int ordinal) {
this.ordinal = ordinal;
}
@Override
public Boolean isTemplateJob() {
return templateJob;
}
@Override
public void setTemplateJob(Boolean templateJob) {
this.templateJob = templateJob;
}
@Override
public Boolean isTemplateBased() {
return templateBased;
}
@Override
public void setTemplateBased(Boolean templateBased) {
this.templateBased = templateBased;
}
@Override
public String getTemplateName() {
return templateName;
}
@Override
public void setTemplateName(String templateName) {
this.templateName = templateName;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.SHORT_PREFIX_STYLE);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SchedulerJob that = (SchedulerJob) o;
return Objects.equals(getIdentifier(), that.getIdentifier());
}
@Override
public int hashCode() {
return Objects.hash(getIdentifier());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy