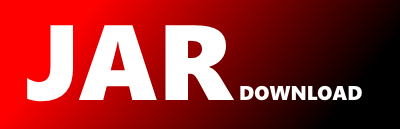
org.imixs.marty.profile.TextUsernameAdapter Maven / Gradle / Ivy
package org.imixs.marty.profile;
import java.util.List;
import java.util.Vector;
import java.util.logging.Logger;
import jakarta.ejb.EJB;
import jakarta.ejb.Stateless;
import jakarta.enterprise.event.Observes;
import org.imixs.workflow.ItemCollection;
import org.imixs.workflow.engine.TextEvent;
import org.imixs.workflow.engine.TextItemValueAdapter;
import org.imixs.workflow.engine.plugins.AbstractPlugin;
import org.imixs.workflow.util.XMLParser;
/**
* The TextUsernameAdapter replaces text fragments with the tag
* .. . The values of the item will be replaced with the
* display name from the corresponding user profile display name.
*
* Example:
*
* {@code
* Workitem updated by: namcurrenteditor .
* }
*
* This will replace the namcurrenteditor with the corrsponding profile full
* username. If the username item value is a multiValue object the single values
* can be spearated by a separator
*
* Example:
*
* {@code
* Team List: txtTeam
* }
*
* @author rsoika
*
*/
@Stateless
public class TextUsernameAdapter {
private static Logger logger = Logger.getLogger(AbstractPlugin.class.getName());
@EJB
ProfileService profileService;
/**
* This method reacts on CDI events of the type TextEvent and parses a string
* for xml tag . Those tags will be replaced with the corresponding
* display name of the user profile.
*
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public void onEvent(@Observes TextEvent event) {
String text = event.getText();
ItemCollection documentContext = event.getDocument();
String sSeparator = " ";
String sItem;
if (text == null)
return;
// lower case into
if (text.contains("")) {
logger.warning("Deprecated tag should be lowercase !");
text = text.replace("", " ");
}
List tagList = XMLParser.findTags(text, "username");
logger.finest(tagList.size() + " tags found");
// test if a tag exists...
for (String tag : tagList) {
// next we check if the start tag contains a 'separator' attribute
sSeparator = XMLParser.findAttribute(tag, "separator");
sItem = XMLParser.findAttribute(tag, "item");
if (sItem==null || sItem.isEmpty()) {
sItem="txtUserName";
}
// extract Item Value
String sItemValue = XMLParser.findTagValue(tag, "username");
List tempList = documentContext.getItemValue(sItemValue);
// clone List
List vUserIDs = new Vector(tempList);
// get usernames ....
for (int i = 0; i < vUserIDs.size(); i++) {
ItemCollection profile = profileService.findProfileById(vUserIDs.get(i));
if (profile != null) {
vUserIDs.set(i, profile.getItemValueString(sItem));
}
}
// format field value
TextItemValueAdapter textItemValueAdapter=new TextItemValueAdapter();
String sResult = textItemValueAdapter.formatItemValues(vUserIDs, sSeparator, "");
// now replace the tag with the result string
int iStartPos = text.indexOf(tag);
int iEndPos = text.indexOf(tag) + tag.length();
text = text.substring(0, iStartPos) + sResult + text.substring(iEndPos);
}
event.setText(text);
}
}