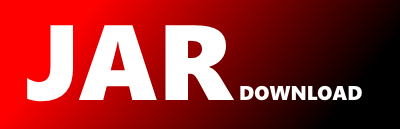
org.imixs.marty.profile.UserInputController Maven / Gradle / Ivy
/*******************************************************************************
* Imixs Workflow Technology
* Copyright (C) 2003, 2008 Imixs Software Solutions GmbH,
* http://www.imixs.com
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* as published by the Free Software Foundation; either version 2
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* General Public License for more details.
*
* You can receive a copy of the GNU General Public
* License at http://www.gnu.org/licenses/gpl.html
*
* Contributors:
* Imixs Software Solutions GmbH - initial API and implementation
* Ralph Soika
*
*******************************************************************************/
package org.imixs.marty.profile;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Vector;
import java.util.logging.Logger;
import jakarta.ejb.EJB;
import jakarta.enterprise.context.RequestScoped;
import jakarta.faces.context.FacesContext;
import jakarta.inject.Inject;
import jakarta.inject.Named;
import org.imixs.workflow.ItemCollection;
import org.imixs.workflow.ItemCollectionComparator;
import org.imixs.workflow.engine.WorkflowService;
import org.imixs.workflow.engine.index.SchemaService;
/**
* The UserInputController provides suggest-box behavior based on the JSF 2.0
* Ajax capability to add user names into a ItemValue of a WorkItem.
*
* Usage:
*
*
*
*
*
* @author rsoika
* @version 1.0
*/
@Named("userInputController")
@RequestScoped
public class UserInputController implements Serializable {
@Inject
protected UserController userController;
@EJB
protected WorkflowService workflowService;
@EJB
protected SchemaService schemaService;
@EJB
protected ProfileService profileService;
private List searchResult = null;
private static int MAX_SEARCH_RESULT = 100;
private static final long serialVersionUID = 1L;
private static Logger logger = Logger.getLogger(UserInputController.class.getName());
public UserInputController() {
super();
searchResult = new ArrayList();
}
/**
* This method returns a list of profile ItemCollections matching the search
* phrase. The search statement includes the items 'txtName', 'txtEmail' and
* 'txtUserName'. The result list is sorted by txtUserName
*
* @param phrase - search phrase
* @return - list of matching profiles
*/
public List searchProfile(String phrase) {
List searchResult = new ArrayList();
if (phrase == null || phrase.isEmpty())
return searchResult;
// start lucene search
Collection col = null;
try {
phrase = phrase.trim().toLowerCase();
phrase = schemaService.escapeSearchTerm(phrase);
// issue #170
phrase = schemaService.normalizeSearchTerm(phrase);
// String sQuery = "(type:profile) AND ($processid:[210 TO 249]) AND ((txtname:" + phrase
// + "*) OR (txtusername:" + phrase + "*) OR (txtemail:" + phrase + "*))";
// Issue #384
String sQuery = "(type:profile) AND ($processid:[210 TO 249]) AND " + phrase + "*";
logger.finest("searchprofile: " + sQuery);
logger.fine("searchWorkitems: " + sQuery);
col = workflowService.getDocumentService().find(sQuery, MAX_SEARCH_RESULT, 0);
} catch (Exception e) {
logger.warning("Lucene error error: " + e.getMessage());
}
if (col != null) {
for (ItemCollection profile : col) {
searchResult.add(profileService.cloneWorkitem(profile));
}
// sort by username..
Collections.sort(searchResult, new ItemCollectionComparator("txtusername", true));
}
return searchResult;
}
public List getSearchResult() {
return searchResult;
}
/**
* This method tests if a given string is a defined Access Role.
*
* @param aName
* @return true if the name is a access role
* @see DocumentService.getAccessRoles()
*/
public boolean isRole(String aName) {
String accessRoles = workflowService.getDocumentService().getAccessRoles();
String roleList = "org.imixs.ACCESSLEVEL.READERACCESS,org.imixs.ACCESSLEVEL.AUTHORACCESS,org.imixs.ACCESSLEVEL.EDITORACCESS,org.imixs.ACCESSLEVEL.MANAGERACCESS,"
+ accessRoles;
List roles = Arrays.asList(roleList.split("\\s*,\\s*"));
if (roles.stream().anyMatch(aName::equalsIgnoreCase)) {
return true;
} else {
return false;
}
}
/**
* This method returns a sorted list of profiles for a given userId list
*
* @param aNameList - string list with user ids
* @return - list of profiles
*/
public List getSortedProfilelist(List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy