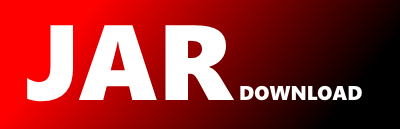
org.immutables.criteria.geode.OqlLiterals Maven / Gradle / Ivy
/*
* Copyright 2020 Immutables Authors and Contributors
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.immutables.criteria.geode;
import com.google.common.collect.ImmutableSet;
import java.text.SimpleDateFormat;
import java.time.Instant;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.ZoneOffset;
import java.time.format.DateTimeFormatter;
import java.util.Date;
import java.util.Objects;
import java.util.Set;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
/**
* Helper class to convert java objects to
* Geode OQL literals like dates, iterables, numbers etc.
* Using bind variables is preferred however in some circumstances like
* Continuous Querying
* it is not possible.
*
* @see Implementing Continuous Querying
*/
final class OqlLiterals {
private OqlLiterals() {}
/**
* Convert java object to Geode OQL literal
*/
static String fromObject(Object value) {
if (value == null) {
return Objects.toString(null);
} else if (value instanceof CharSequence) {
return charSequence((CharSequence) value);
} else if (value instanceof Pattern) {
return pattern((Pattern) value);
} else if (value instanceof Iterable) {
return iterable((Iterable>) value);
} else if (value.getClass().isEnum()) {
return fromEnum((Enum>) value);
} else if (value instanceof LocalDate) {
return localDate((LocalDate) value);
} else if (value instanceof LocalDateTime) {
return localDateTime((LocalDateTime) value);
} else if (value instanceof Instant) {
return instant((Instant) value);
} else if (value instanceof Date) {
return date((Date) value);
} else if (value instanceof Long) {
return longValue((Long) value);
}
// probably string is best representation in OQL (without bind variables)
return Objects.toString(value);
}
private static String iterable(Iterable> value) {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy