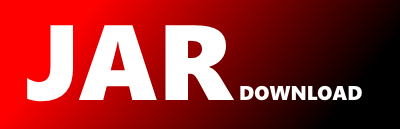
org.immutables.fixture.modifiable.ModifiableImmutableWithModifiable Maven / Gradle / Ivy
Show all versions of value-fixture Show documentation
package org.immutables.fixture.modifiable;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* A modifiable implementation of the {@link AbstractImmutableWithModifiable AbstractImmutableWithModifiable} type.
* Use the {@link #create()} static factory methods to create new instances.
* Use the {@link #toImmutable()} method to convert to canonical immutable instances.
*
ModifiableImmutableWithModifiable is not thread-safe
* @see ImmutableWithModifiable
*/
@Generated(from = "AbstractImmutableWithModifiable", generator = "Modifiables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated({"Modifiables.generator", "AbstractImmutableWithModifiable"})
@NotThreadSafe
public final class ModifiableImmutableWithModifiable
implements AbstractImmutableWithModifiable {
private int id;
private String description;
private final ArrayList names = new ArrayList();
private ModifiableImmutableWithModifiable() {}
/**
* Construct a modifiable instance of {@code AbstractImmutableWithModifiable}.
* @return A new modifiable instance
*/
public static ModifiableImmutableWithModifiable create() {
return new ModifiableImmutableWithModifiable();
}
/**
* @return value of {@code id} attribute
*/
@Override
public final int getId() {
return id;
}
/**
* @return value of {@code description} attribute, may be {@code null}
*/
@Override
public final String getDescription() {
return description;
}
/**
* @return modifiable list {@code names}
*/
@Override
public final List getNames() {
return names;
}
/**
* Clears the object by setting all attributes to their initial values.
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableImmutableWithModifiable clear() {
id = 0;
description = null;
names.clear();
return this;
}
/**
* Fill this modifiable instance with attribute values from the provided {@link AbstractImmutableWithModifiable} instance.
* Regular attribute values will be overridden, i.e. replaced with ones of an instance.
* Any of the instance's absent optional values will not be copied (will not override current values).
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} for use in a chained invocation
*/
public ModifiableImmutableWithModifiable from(AbstractImmutableWithModifiable instance) {
Objects.requireNonNull(instance, "instance");
if (instance instanceof ModifiableImmutableWithModifiable) {
from((ModifiableImmutableWithModifiable) instance);
return this;
}
setId(instance.getId());
String descriptionValue = instance.getDescription();
if (descriptionValue != null) {
setDescription(descriptionValue);
}
addAllNames(instance.getNames());
return this;
}
/**
* Fill this modifiable instance with attribute values from the provided {@link AbstractImmutableWithModifiable} instance.
* Regular attribute values will be overridden, i.e. replaced with ones of an instance.
* Any of the instance's absent optional values will not be copied (will not override current values).
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} for use in a chained invocation
*/
public ModifiableImmutableWithModifiable from(ModifiableImmutableWithModifiable instance) {
Objects.requireNonNull(instance, "instance");
setId(instance.getId());
String descriptionValue = instance.getDescription();
if (descriptionValue != null) {
setDescription(descriptionValue);
}
addAllNames(instance.getNames());
return this;
}
/**
* Assigns a value to the {@link AbstractImmutableWithModifiable#getId() id} attribute.
* @param id The value for id
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableImmutableWithModifiable setId(int id) {
this.id = id;
return this;
}
/**
* Assigns a value to the {@link AbstractImmutableWithModifiable#getDescription() description} attribute.
* @param description The value for description, can be {@code null}
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableImmutableWithModifiable setDescription(String description) {
this.description = description;
return this;
}
/**
* Adds one element to {@link AbstractImmutableWithModifiable#getNames() names} list.
* @param element The names element
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableImmutableWithModifiable addNames(String element) {
Objects.requireNonNull(element, "names element");
this.names.add(element);
return this;
}
/**
* Adds elements to {@link AbstractImmutableWithModifiable#getNames() names} list.
* @param elements An array of names elements
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public final ModifiableImmutableWithModifiable addNames(String... elements) {
for (String e : elements) {
addNames(e);
}
return this;
}
/**
* Sets or replaces all elements for {@link AbstractImmutableWithModifiable#getNames() names} list.
* @param elements An iterable of names elements
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableImmutableWithModifiable setNames(Iterable elements) {
this.names.clear();
addAllNames(elements);
return this;
}
/**
* Adds elements to {@link AbstractImmutableWithModifiable#getNames() names} list.
* @param elements An iterable of names elements
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableImmutableWithModifiable addAllNames(Iterable elements) {
for (String e : elements) {
addNames(e);
}
return this;
}
/**
* Returns {@code true} if all required attributes are set, indicating that the object is initialized.
* @return {@code true} if set
*/
public final boolean isInitialized() {
return true;
}
/**
* Converts to {@link ImmutableWithModifiable ImmutableWithModifiable}.
* @return An immutable instance of ImmutableWithModifiable
*/
public final ImmutableWithModifiable toImmutable() {
return ImmutableWithModifiable.copyOf(this);
}
/**
* This instance is equal to all instances of {@code ModifiableImmutableWithModifiable} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
if (!(another instanceof ModifiableImmutableWithModifiable)) return false;
ModifiableImmutableWithModifiable other = (ModifiableImmutableWithModifiable) another;
return equalTo(other);
}
private boolean equalTo(ModifiableImmutableWithModifiable another) {
return id == another.id
&& Objects.equals(description, another.description)
&& names.equals(another.names);
}
/**
* Computes a hash code from attributes: {@code id}, {@code description}, {@code names}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + id;
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + names.hashCode();
return h;
}
/**
* Generates a string representation of this {@code AbstractImmutableWithModifiable}.
* If uninitialized, some attribute values may appear as question marks.
* @return A string representation
*/
@Override
public String toString() {
return "ModifiableImmutableWithModifiable{"
+ "id=" + getId()
+ ", description=" + getDescription()
+ ", names=" + getNames()
+ "}";
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}