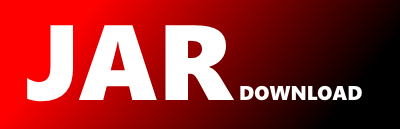
org.immutables.fixture.serial.ImmutableSomeSer Maven / Gradle / Ivy
package org.immutables.fixture.serial;
import com.google.common.base.MoreObjects;
import com.google.common.base.Preconditions;
import com.google.common.collect.Interner;
import com.google.common.collect.Interners;
import java.io.ObjectStreamException;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link SomeSer}.
*
* Use the builder to create immutable instances:
* {@code ImmutableSomeSer.builder()}.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "SomeSer"})
@Immutable
public final class ImmutableSomeSer extends SomeSer {
private final int regular;
private ImmutableSomeSer(ImmutableSomeSer.Builder builder) {
this.regular = builder.regularIsSet()
? builder.regular
: super.regular();
}
private ImmutableSomeSer(int regular) {
this.regular = regular;
}
/**
* @return The value of the {@code regular} attribute
*/
@Override
int regular() {
return regular;
}
/**
* Copy the current immutable object by setting a value for the {@link SomeSer#regular() regular} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param regular A new value for regular
* @return A modified copy of the {@code this} object
*/
public final ImmutableSomeSer withRegular(int regular) {
if (this.regular == regular) return this;
return validate(new ImmutableSomeSer(regular));
}
/**
* This instance is equal to all instances of {@code ImmutableSomeSer} that have equal attribute values.
* As instances of the {@code ImmutableSomeSer} class are interned, the {@code equals} method is implemented
* as an efficient reference equality check.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
return this == another;
}
private boolean equalTo(ImmutableSomeSer another) {
return regular == another.regular;
}
/**
* Computes a hash code from attributes: {@code regular}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + regular;
return h;
}
/**
* Prints the immutable value {@code SomeSer} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("SomeSer")
.omitNullValues()
.add("regular", regular)
.toString();
}
private transient volatile long lazyInitBitmap;
private static final long VERSION_LAZY_INIT_BIT = 0x1L;
private transient int version;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link SomeSer#version() version} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* @return A lazily initialized value of the {@code l.name} attribute
*/
@Override
public int version() {
if ((lazyInitBitmap & VERSION_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & VERSION_LAZY_INIT_BIT) == 0) {
this.version = super.version();
lazyInitBitmap |= VERSION_LAZY_INIT_BIT;
}
}
}
return version;
}
private static class InternProxy {
final ImmutableSomeSer instance;
InternProxy(ImmutableSomeSer instance) {
this.instance = instance;
}
@Override
public boolean equals(@Nullable Object another) {
return instance.equalTo(((InternProxy) another).instance);
}
@Override
public int hashCode() {
return instance.hashCode();
}
}
private static final Interner INTERNER = Interners.newStrongInterner();
private static ImmutableSomeSer validate(ImmutableSomeSer instance) {
return INTERNER.intern(new InternProxy(instance)).instance;
}
/**
* Creates an immutable copy of a {@link SomeSer} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SomeSer instance
*/
public static ImmutableSomeSer copyOf(SomeSer instance) {
if (instance instanceof ImmutableSomeSer) {
return (ImmutableSomeSer) instance;
}
return ImmutableSomeSer.builder()
.from(instance)
.build();
}
private static final long serialVersionUID = 1L;
private Object readResolve() throws ObjectStreamException {
return validate(this);
}
/**
* Creates a builder for {@link ImmutableSomeSer ImmutableSomeSer}.
* @return A new ImmutableSomeSer builder
*/
public static ImmutableSomeSer.Builder builder() {
return new ImmutableSomeSer.Builder();
}
/**
* Builds instances of type {@link ImmutableSomeSer ImmutableSomeSer}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
public static final class Builder {
private static final long OPT_BIT_REGULAR = 0x1L;
private long optBits;
private int regular;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code SomeSer} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(SomeSer instance) {
Preconditions.checkNotNull(instance, "instance");
regular(instance.regular());
return this;
}
/**
* Initializes the value for the {@link SomeSer#regular() regular} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link SomeSer#regular() regular}.
* @param regular The value for regular
* @return {@code this} builder for use in a chained invocation
*/
public final Builder regular(int regular) {
this.regular = regular;
optBits |= OPT_BIT_REGULAR;
return this;
}
/**
* Builds a new {@link ImmutableSomeSer ImmutableSomeSer}.
* @return An immutable instance of SomeSer
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableSomeSer build() {
return ImmutableSomeSer.validate(new ImmutableSomeSer(this));
}
private boolean regularIsSet() {
return (optBits & OPT_BIT_REGULAR) != 0;
}
}
}