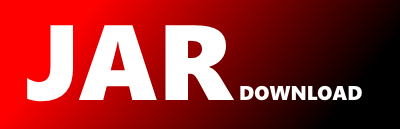
org.immutables.fixture.generics.ModifiableFirstie Maven / Gradle / Ivy
package org.immutables.fixture.generics;
import com.google.common.base.MoreObjects;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.NotThreadSafe;
/**
* A modifiable implementation of the {@link Firstie Firstie} type.
* Use the {@link #create()} static factory methods to create new instances.
* Use the {@link #toImmutable()} method to convert to canonical immutable instances.
*
ModifiableFirstie is not thread-safe
* @param generic parameter T
* @param generic parameter V
* @see ImmutableFirstie
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Modifiables.generator", "Firstie"})
@NotThreadSafe
public final class ModifiableFirstie
implements Firstie {
private static final long INIT_BIT_REF = 0x1L;
private long initBits = 0x1L;
private T ref;
private final ArrayList commands = new ArrayList();
private ModifiableFirstie() {}
/**
* Construct a modifiable instance of {@code Firstie}.
* @param generic parameter T
* @param generic parameter V
* @return A new modifiable instance
*/
public static ModifiableFirstie create() {
return new ModifiableFirstie<>();
}
/**
* @return value of {@code ref} attribute
*/
@Override
public final T ref() {
if (!refIsSet()) {
checkRequiredAttributes();
}
return ref;
}
/**
* @return modifiable list {@code commands}
*/
@Override
public final List commands() {
return commands;
}
/**
* Clears the object by setting all attributes to their initial values.
* @return {@code this} for use in a chained invocation
*/
public ModifiableFirstie clear() {
initBits = 0x1L;
ref = null;
commands.clear();
return this;
}
/**
* Fill this modifiable instance with attribute values from the provided {@link Firstie} instance.
* Regular attribute values will be overridden, i.e. replaced with ones of an instance.
* Any of the instance's absent optional values will not be copied (will not override current values).
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} for use in a chained invocation
*/
public ModifiableFirstie from(Firstie instance) {
Preconditions.checkNotNull(instance, "instance");
setRef(instance.ref());
addAllCommands(instance.commands());
return this;
}
/**
* Assigns a value to the {@link Firstie#ref() ref} attribute.
* @param ref The value for ref
* @return {@code this} for use in a chained invocation
*/
public ModifiableFirstie setRef(T ref) {
this.ref = Preconditions.checkNotNull(ref, "ref");
initBits &= ~INIT_BIT_REF;
return this;
}
/**
* Adds one element to {@link Firstie#commands() commands} list.
* @param element The commands element
* @return {@code this} for use in a chained invocation
*/
public ModifiableFirstie addCommands(V element) {
commands.add(Preconditions.checkNotNull(element, "commands element"));
return this;
}
/**
* Adds elements to {@link Firstie#commands() commands} list.
* @param elements An array of commands elements
* @return {@code this} for use in a chained invocation
*/
@SafeVarargs
public final ModifiableFirstie addCommands(V... elements) {
for (V element : elements) {
addCommands(Preconditions.checkNotNull(element, "commands element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link Firstie#commands() commands} list.
* @param elements An iterable of commands elements
* @return {@code this} for use in a chained invocation
*/
public ModifiableFirstie setCommands(Iterable extends V> elements) {
commands.clear();
return addAllCommands(elements);
}
/**
* Adds elements to {@link Firstie#commands() commands} list.
* @param elements An iterable of commands elements
* @return {@code this} for use in a chained invocation
*/
public ModifiableFirstie addAllCommands(Iterable extends V> elements) {
for (V element : elements) {
commands.add(Preconditions.checkNotNull(element, "commands element"));
}
return this;
}
/**
* Returns {@code true} if the required attribute {@link Firstie#ref() ref} is set.
* @return {@code true} if set
*/
public final boolean refIsSet() {
return (initBits & INIT_BIT_REF) == 0;
}
/**
* Returns {@code true} if all required attributes are set, indicating that the object is initialized.
* @return {@code true} if set
*/
public final boolean isInitialized() {
return initBits == 0;
}
private void checkRequiredAttributes() {
if (!isInitialized()) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = Lists.newArrayList();
if (!refIsSet()) attributes.add("ref");
return "Firstie in not initialized, some of the required attributes are not set " + attributes;
}
/**
* Converts to {@link ImmutableFirstie ImmutableFirstie}.
* @return An immutable instance of Firstie
*/
public final ImmutableFirstie toImmutable() {
checkRequiredAttributes();
return ImmutableFirstie.copyOf(this);
}
/**
* This instance is equal to all instances of {@code ModifiableFirstie} that have equal attribute values.
* An uninitialized instance is equal only to itself.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
if (!(another instanceof ModifiableFirstie)) return false;
ModifiableFirstie other = (ModifiableFirstie) another;
if (!isInitialized() || !other.isInitialized()) {
return false;
}
return equalTo(other);
}
private boolean equalTo(ModifiableFirstie another) {
return ref.equals(another.ref)
&& commands.equals(another.commands);
}
/**
* Computes a hash code from attributes: {@code ref}, {@code commands}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + ref.hashCode();
h = h * 17 + commands.hashCode();
return h;
}
/**
* Generates a string representation of this {@code Firstie}.
* If uninitialized, some attribute values may appear as question marks.
* @return A string representation
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ModifiableFirstie")
.add("ref", refIsSet() ? ref() : "?")
.add("commands", commands())
.toString();
}
}