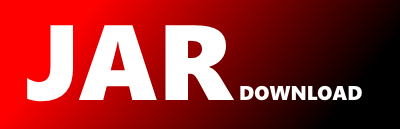
org.immutables.fixture.strict.ImmutableBar Maven / Gradle / Ivy
package org.immutables.fixture.strict;
import com.google.common.base.MoreObjects;
import com.google.common.base.Optional;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.primitives.Ints;
import java.util.Map;
import javax.annotation.Generated;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
/**
* Immutable implementation of {@link Bar}.
*
* Use the builder to create immutable instances:
* {@code ImmutableBar.builder()}.
*/
@SuppressWarnings("all")
@ParametersAreNonnullByDefault
@Generated({"Immutables.generator", "Bar"})
@Immutable
final class ImmutableBar implements Bar {
private final ImmutableList nums;
private final ImmutableMap mps;
private final Optional opt;
private ImmutableBar(
ImmutableList nums,
ImmutableMap mps,
Optional opt) {
this.nums = nums;
this.mps = mps;
this.opt = opt;
}
/**
* @return The value of the {@code nums} attribute
*/
@Override
public ImmutableList nums() {
return nums;
}
/**
* @return The value of the {@code mps} attribute
*/
@Override
public ImmutableMap mps() {
return mps;
}
/**
* @return The value of the {@code opt} attribute
*/
@Override
public Optional opt() {
return opt;
}
/**
* Copy the current immutable object with elements that replace the content of {@link Bar#nums() nums}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableBar withNums(int... elements) {
ImmutableList newValue = ImmutableList.copyOf(Ints.asList(elements));
return new ImmutableBar(newValue, this.mps, this.opt);
}
/**
* Copy the current immutable object with elements that replace the content of {@link Bar#nums() nums}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of nums elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableBar withNums(Iterable elements) {
if (this.nums == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableBar(newValue, this.mps, this.opt);
}
/**
* Copy the current immutable object by replacing the {@link Bar#mps() mps} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param mps The entries to be added to the mps map
* @return A modified copy of {@code this} object
*/
public final ImmutableBar withMps(Map mps) {
if (this.mps == mps) return this;
ImmutableMap newValue = ImmutableMap.copyOf(mps);
return new ImmutableBar(this.nums, newValue, this.opt);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link Bar#opt() opt} attribute.
* @param value The value for opt
* @return A modified copy of {@code this} object
*/
public final ImmutableBar withOpt(int value) {
Optional newValue = Optional.of(value);
if (this.opt.equals(newValue)) return this;
return new ImmutableBar(this.nums, this.mps, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link Bar#opt() opt} attribute.
* An equality check is used to prevent copying of the same value by returning {@code this}.
* @param optional A value for opt
* @return A modified copy of {@code this} object
*/
public final ImmutableBar withOpt(Optional optional) {
Optional value = Preconditions.checkNotNull(optional, "opt");
if (this.opt.equals(value)) return this;
return new ImmutableBar(this.nums, this.mps, value);
}
/**
* This instance is equal to all instances of {@code ImmutableBar} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableBar
&& equalTo((ImmutableBar) another);
}
private boolean equalTo(ImmutableBar another) {
return nums.equals(another.nums)
&& mps.equals(another.mps)
&& opt.equals(another.opt);
}
/**
* Computes a hash code from attributes: {@code nums}, {@code mps}, {@code opt}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 31;
h = h * 17 + nums.hashCode();
h = h * 17 + mps.hashCode();
h = h * 17 + opt.hashCode();
return h;
}
/**
* Prints the immutable value {@code Bar} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Bar")
.omitNullValues()
.add("nums", nums)
.add("mps", mps)
.add("opt", opt.orNull())
.toString();
}
/**
* Creates an immutable copy of a {@link Bar} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Bar instance
*/
public static ImmutableBar copyOf(Bar instance) {
if (instance instanceof ImmutableBar) {
return (ImmutableBar) instance;
}
return ImmutableBar.builder()
.addAllNums(instance.nums())
.putAllMps(instance.mps())
.opt(instance.opt())
.build();
}
/**
* Creates a builder for {@link ImmutableBar ImmutableBar}.
* @return A new ImmutableBar builder
*/
public static ImmutableBar.Builder builder() {
return new ImmutableBar.Builder();
}
/**
* Builds instances of type {@link ImmutableBar ImmutableBar}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@NotThreadSafe
static final class Builder {
private static final long OPT_BIT_OPT = 0x1L;
private long optBits;
private ImmutableList.Builder nums = ImmutableList.builder();
private ImmutableMap.Builder mps = ImmutableMap.builder();
private Optional opt = Optional.absent();
private Builder() {
}
/**
* Adds one element to {@link Bar#nums() nums} list.
* @param element A nums element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addNums(int element) {
this.nums.add(element);
return this;
}
/**
* Adds elements to {@link Bar#nums() nums} list.
* @param elements An array of nums elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addNums(int... elements) {
this.nums.addAll(Ints.asList(elements));
return this;
}
/**
* Adds elements to {@link Bar#nums() nums} list.
* @param elements An iterable of nums elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllNums(Iterable elements) {
this.nums.addAll(elements);
return this;
}
/**
* Put one entry to the {@link Bar#mps() mps} map.
* @param key The key in the mps map
* @param value The associated value in the mps map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putMps(String key, int value) {
this.mps.put(key, value);
return this;
}
/**
* Put one entry to the {@link Bar#mps() mps} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putMps(Map.Entry entry) {
this.mps.put(entry);
return this;
}
/**
* Put all mappings from the specified map as entries to {@link Bar#mps() mps} map. Nulls are not permitted
* @param mps The entries that will be added to the mps map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putAllMps(Map mps) {
this.mps.putAll(mps);
return this;
}
/**
* Initializes the optional value {@link Bar#opt() opt} to opt.
* @param opt The value for opt
* @return {@code this} builder for chained invocation
*/
public final Builder opt(int opt) {
checkNotIsSet(optIsSet(), "opt");
this.opt = Optional.of(opt);
optBits |= OPT_BIT_OPT;
return this;
}
/**
* Initializes the optional value {@link Bar#opt() opt} to opt.
* @param opt The value for opt
* @return {@code this} builder for use in a chained invocation
*/
public final Builder opt(Optional opt) {
checkNotIsSet(optIsSet(), "opt");
this.opt = Preconditions.checkNotNull(opt, "opt");
optBits |= OPT_BIT_OPT;
return this;
}
/**
* Builds a new {@link ImmutableBar ImmutableBar}.
* @return An immutable instance of Bar
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableBar build() {
return new ImmutableBar(nums.build(), mps.build(), opt);
}
private boolean optIsSet() {
return (optBits & OPT_BIT_OPT) != 0;
}
private void checkNotIsSet(boolean isSet, String name) {
if (isSet) throw new IllegalStateException("Builder of Bar is strict, attribute is already set: ".concat(name));
}
}
}