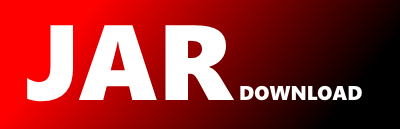
org.immutables.fixture.ImmutableSillyMapTup Maven / Gradle / Ivy
Show all versions of value-fixture Show documentation
package org.immutables.fixture;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Maps;
import com.google.errorprone.annotations.Var;
import java.lang.annotation.RetentionPolicy;
import java.util.Map;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link SillyMapTup}.
*
* Use the static factory method to create immutable instances:
* {@code ImmutableSillyMapTup.of()}.
*/
@Generated(from = "SillyMapTup", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableSillyMapTup extends SillyMapTup {
private final ImmutableMap holder1;
private final int value;
private ImmutableSillyMapTup(Map holder1, int value) {
this.holder1 = Maps.immutableEnumMap(holder1);
this.value = value;
}
private ImmutableSillyMapTup(
ImmutableSillyMapTup original,
ImmutableMap holder1,
int value) {
this.holder1 = holder1;
this.value = value;
}
/**
* @return The value of the {@code holder1} attribute
*/
@Override
public ImmutableMap holder1() {
return holder1;
}
/**
* @return The value of the {@code value} attribute
*/
@Override
public int value() {
return value;
}
/**
* Copy the current immutable object by replacing the {@link SillyMapTup#holder1() holder1} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the holder1 map
* @return A modified copy of {@code this} object
*/
public final ImmutableSillyMapTup withHolder1(Map entries) {
if (this.holder1 == entries) return this;
ImmutableMap newValue = Maps.immutableEnumMap(entries);
return new ImmutableSillyMapTup(this, newValue, this.value);
}
/**
* Copy the current immutable object by setting a value for the {@link SillyMapTup#value() value} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for value
* @return A modified copy of the {@code this} object
*/
public final ImmutableSillyMapTup withValue(int value) {
if (this.value == value) return this;
return new ImmutableSillyMapTup(this, this.holder1, value);
}
/**
* This instance is equal to all instances of {@code ImmutableSillyMapTup} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableSillyMapTup
&& equalTo(0, (ImmutableSillyMapTup) another);
}
private boolean equalTo(int synthetic, ImmutableSillyMapTup another) {
return holder1.equals(another.holder1)
&& value == another.value;
}
/**
* Computes a hash code from attributes: {@code holder1}, {@code value}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + holder1.hashCode();
h += (h << 5) + value;
return h;
}
/**
* Prints the immutable value {@code SillyMapTup} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("SillyMapTup")
.omitNullValues()
.add("holder1", holder1)
.add("value", value)
.toString();
}
/**
* Construct a new immutable {@code SillyMapTup} instance.
* @param holder1 The value for the {@code holder1} attribute
* @param value The value for the {@code value} attribute
* @return An immutable SillyMapTup instance
*/
public static ImmutableSillyMapTup of(Map holder1, int value) {
return new ImmutableSillyMapTup(holder1, value);
}
/**
* Creates an immutable copy of a {@link SillyMapTup} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SillyMapTup instance
*/
public static ImmutableSillyMapTup copyOf(SillyMapTup instance) {
if (instance instanceof ImmutableSillyMapTup) {
return (ImmutableSillyMapTup) instance;
}
return ImmutableSillyMapTup.of(instance.holder1(), instance.value());
}
}