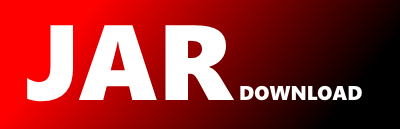
org.immutables.fixture.modifiable.ModifiableSmall Maven / Gradle / Ivy
Show all versions of value-fixture Show documentation
package org.immutables.fixture.modifiable;
import com.google.common.base.MoreObjects;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* A modifiable implementation of the {@link Companion.Small Small} type.
* Use the constructor to create new modifiable instances. You may even extend this class to
* add some convenience methods, however most of the methods in this class are final
* to preserve safety and predictable invariants.
*
ModifiableSmall is not thread-safe
*/
@Generated(from = "Companion.Small", generator = "Modifiables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated({"Modifiables.generator", "Companion.Small"})
@NotThreadSafe
public class ModifiableSmall implements Companion.Small {
private static final long INIT_BIT_FIRST = 0x1L;
private static final long INIT_BIT_SECOND = 0x2L;
private long initBits = 0x3L;
private int first;
private String second;
/**
* Construct a modifiable instance of {@code Small}.
* @param first The value for the {@code first} attribute
* @param second The value for the {@code second} attribute
*/
public ModifiableSmall(int first, String second) {
setFirst(first);
setSecond(second);
}
/**
* Construct a modifiable instance of {@code Small}.
*/
public ModifiableSmall() {}
/**
* @return value of {@code first} attribute
*/
@Override
public final int first() {
if (!firstIsSet()) {
checkRequiredAttributes();
}
return first;
}
/**
* @return value of {@code second} attribute
*/
@Override
public final String second() {
if (!secondIsSet()) {
checkRequiredAttributes();
}
return second;
}
/**
* Clears the object by setting all attributes to their initial values.
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableSmall clear() {
initBits = 0x3L;
first = 0;
second = null;
return this;
}
/**
* Fill this modifiable instance with attribute values from the provided {@link Companion.Small} instance.
* Regular attribute values will be overridden, i.e. replaced with ones of an instance.
* Any of the instance's absent optional values will not be copied (will not override current values).
* @param instance The instance from which to copy values
* @return {@code this} for use in a chained invocation
*/
public ModifiableSmall from(Companion.Small instance) {
Objects.requireNonNull(instance, "instance");
if (instance instanceof ModifiableSmall) {
from((ModifiableSmall) instance);
return this;
}
setFirst(instance.first());
setSecond(instance.second());
return this;
}
/**
* Fill this modifiable instance with attribute values from the provided {@link Companion.Small} instance.
* Regular attribute values will be overridden, i.e. replaced with ones of an instance.
* Any of the instance's absent optional values will not be copied (will not override current values).
* @param instance The instance from which to copy values
* @return {@code this} for use in a chained invocation
*/
public ModifiableSmall from(ModifiableSmall instance) {
Objects.requireNonNull(instance, "instance");
if (instance.firstIsSet()) {
setFirst(instance.first());
}
if (instance.secondIsSet()) {
setSecond(instance.second());
}
return this;
}
/**
* Assigns a value to the {@code first} attribute.
* @param first The value for first
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableSmall setFirst(int first) {
this.first = first;
initBits &= ~INIT_BIT_FIRST;
return this;
}
/**
* Assigns a value to the {@code second} attribute.
* @param second The value for second
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public ModifiableSmall setSecond(String second) {
this.second = Objects.requireNonNull(second, "second");
initBits &= ~INIT_BIT_SECOND;
return this;
}
/**
* Returns {@code true} if the required attribute {@code first} is set.
* @return {@code true} if set
*/
public final boolean firstIsSet() {
return (initBits & INIT_BIT_FIRST) == 0;
}
/**
* Returns {@code true} if the required attribute {@code second} is set.
* @return {@code true} if set
*/
public final boolean secondIsSet() {
return (initBits & INIT_BIT_SECOND) == 0;
}
/**
* Reset an attribute to its initial value.
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public final ModifiableSmall unsetFirst() {
initBits |= INIT_BIT_FIRST;
first = 0;
return this;
}
/**
* Reset an attribute to its initial value.
* @return {@code this} for use in a chained invocation
*/
@CanIgnoreReturnValue
public final ModifiableSmall unsetSecond() {
initBits |= INIT_BIT_SECOND;
second = null;
return this;
}
/**
* Returns {@code true} if all required attributes are set, indicating that the object is initialized.
* @return {@code true} if set
*/
public final boolean isInitialized() {
return initBits == 0;
}
private void checkRequiredAttributes() {
if (!isInitialized()) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if (!firstIsSet()) attributes.add("first");
if (!secondIsSet()) attributes.add("second");
return "Small is not initialized, some of the required attributes are not set " + attributes;
}
/**
* This instance is equal to all instances of {@code ModifiableSmall} that have equal attribute values.
* An uninitialized instance is equal only to itself.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
if (!(another instanceof ModifiableSmall)) return false;
ModifiableSmall other = (ModifiableSmall) another;
if (!isInitialized() || !other.isInitialized()) {
return false;
}
return equalTo(other);
}
private boolean equalTo(ModifiableSmall another) {
return first == another.first
&& second.equals(another.second);
}
/**
* Computes a hash code from attributes: {@code first}, {@code second}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + first;
h += (h << 5) + second.hashCode();
return h;
}
/**
* Generates a string representation of this {@code Small}.
* If uninitialized, some attribute values may appear as question marks.
* @return A string representation
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ModifiableSmall")
.add("first", firstIsSet() ? first() : "?")
.add("second", secondIsSet() ? second() : "?")
.toString();
}
}