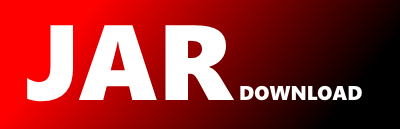
org.incendo.cloud.brigadier.node.ImmutableArgumentMapping Maven / Gradle / Ivy
Show all versions of cloud-brigadier Show documentation
//
// MIT License
//
// Copyright (c) 2024 Incendo
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
package org.incendo.cloud.brigadier.node;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.apiguardian.api.API;
import org.checkerframework.checker.nullness.qual.NonNull;
import org.checkerframework.checker.nullness.qual.Nullable;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ArgumentMapping}.
*
* Use the builder to create immutable instances:
* {@code ImmutableArgumentMapping.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableArgumentMapping.of()}.
*/
@Generated(from = "ArgumentMapping", generator = "Immutables")
@SuppressWarnings({"all"})
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@API(status = API.Status.STABLE, consumers = "org.incendo.cloud.*")
final class ImmutableArgumentMapping implements ArgumentMapping {
private final com.mojang.brigadier.arguments.@NonNull ArgumentType> argumentType;
private final org.incendo.cloud.brigadier.suggestion.@NonNull SuggestionsType suggestionsType;
private final com.mojang.brigadier.suggestion.@Nullable SuggestionProvider suggestionProvider;
private ImmutableArgumentMapping(
com.mojang.brigadier.arguments.@NonNull ArgumentType> argumentType,
org.incendo.cloud.brigadier.suggestion.@NonNull SuggestionsType suggestionsType,
com.mojang.brigadier.suggestion.@Nullable SuggestionProvider suggestionProvider) {
this.argumentType = Objects.requireNonNull(argumentType, "argumentType");
this.suggestionsType = Objects.requireNonNull(suggestionsType, "suggestionsType");
this.suggestionProvider = suggestionProvider;
}
private ImmutableArgumentMapping(ImmutableArgumentMapping.Builder builder) {
this.argumentType = builder.argumentType;
this.suggestionProvider = builder.suggestionProvider;
this.suggestionsType = builder.suggestionsType != null
? builder.suggestionsType
: Objects.requireNonNull(ArgumentMapping.super.suggestionsType(), "suggestionsType");
}
private ImmutableArgumentMapping(
ImmutableArgumentMapping original,
com.mojang.brigadier.arguments.@NonNull ArgumentType> argumentType,
org.incendo.cloud.brigadier.suggestion.@NonNull SuggestionsType suggestionsType,
com.mojang.brigadier.suggestion.@Nullable SuggestionProvider suggestionProvider) {
this.argumentType = argumentType;
this.suggestionsType = suggestionsType;
this.suggestionProvider = suggestionProvider;
}
/**
* @return The value of the {@code argumentType} attribute
*/
@Override
public com.mojang.brigadier.arguments.@NonNull ArgumentType> argumentType() {
return argumentType;
}
/**
* @return The value of the {@code suggestionsType} attribute
*/
@Override
public org.incendo.cloud.brigadier.suggestion.@NonNull SuggestionsType suggestionsType() {
return suggestionsType;
}
/**
* @return The value of the {@code suggestionProvider} attribute
*/
@Override
public com.mojang.brigadier.suggestion.@Nullable SuggestionProvider suggestionProvider() {
return suggestionProvider;
}
/**
* Copy the current immutable object by setting a value for the {@link ArgumentMapping#argumentType() argumentType} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for argumentType
* @return A modified copy of the {@code this} object
*/
public final ImmutableArgumentMapping withArgumentType(com.mojang.brigadier.arguments.@NonNull ArgumentType> value) {
if (this.argumentType == value) return this;
com.mojang.brigadier.arguments.@NonNull ArgumentType> newValue = Objects.requireNonNull(value, "argumentType");
return new ImmutableArgumentMapping<>(this, newValue, this.suggestionsType, this.suggestionProvider);
}
/**
* Copy the current immutable object by setting a value for the {@link ArgumentMapping#suggestionsType() suggestionsType} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for suggestionsType
* @return A modified copy of the {@code this} object
*/
public final ImmutableArgumentMapping withSuggestionsType(org.incendo.cloud.brigadier.suggestion.@NonNull SuggestionsType value) {
org.incendo.cloud.brigadier.suggestion.@NonNull SuggestionsType newValue = Objects.requireNonNull(value, "suggestionsType");
if (this.suggestionsType == newValue) return this;
return new ImmutableArgumentMapping<>(this, this.argumentType, newValue, this.suggestionProvider);
}
/**
* Copy the current immutable object by setting a value for the {@link ArgumentMapping#suggestionProvider() suggestionProvider} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for suggestionProvider (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableArgumentMapping withSuggestionProvider(com.mojang.brigadier.suggestion.@Nullable SuggestionProvider value) {
if (this.suggestionProvider == value) return this;
return new ImmutableArgumentMapping<>(this, this.argumentType, this.suggestionsType, value);
}
/**
* This instance is equal to all instances of {@code ImmutableArgumentMapping} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableArgumentMapping>
&& equalTo(0, (ImmutableArgumentMapping>) another);
}
private boolean equalTo(int synthetic, ImmutableArgumentMapping> another) {
return argumentType.equals(another.argumentType)
&& suggestionsType.equals(another.suggestionsType)
&& Objects.equals(suggestionProvider, another.suggestionProvider);
}
/**
* Computes a hash code from attributes: {@code argumentType}, {@code suggestionsType}, {@code suggestionProvider}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + argumentType.hashCode();
h += (h << 5) + suggestionsType.hashCode();
h += (h << 5) + Objects.hashCode(suggestionProvider);
return h;
}
/**
* Prints the immutable value {@code ArgumentMapping} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ArgumentMapping{"
+ "argumentType=" + argumentType
+ ", suggestionsType=" + suggestionsType
+ ", suggestionProvider=" + suggestionProvider
+ "}";
}
/**
* Construct a new immutable {@code ArgumentMapping} instance.
* @param generic parameter S
* @param argumentType The value for the {@code argumentType} attribute
* @param suggestionsType The value for the {@code suggestionsType} attribute
* @param suggestionProvider The value for the {@code suggestionProvider} attribute
* @return An immutable ArgumentMapping instance
*/
public static ImmutableArgumentMapping of(com.mojang.brigadier.arguments.@NonNull ArgumentType> argumentType, org.incendo.cloud.brigadier.suggestion.@NonNull SuggestionsType suggestionsType, com.mojang.brigadier.suggestion.@Nullable SuggestionProvider suggestionProvider) {
return new ImmutableArgumentMapping<>(argumentType, suggestionsType, suggestionProvider);
}
/**
* Creates an immutable copy of a {@link ArgumentMapping} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param generic parameter S
* @param instance The instance to copy
* @return A copied immutable ArgumentMapping instance
*/
public static ImmutableArgumentMapping copyOf(ArgumentMapping instance) {
if (instance instanceof ImmutableArgumentMapping>) {
return (ImmutableArgumentMapping) instance;
}
return ImmutableArgumentMapping.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableArgumentMapping ImmutableArgumentMapping}.
*
* ImmutableArgumentMapping.<S>builder()
* .argumentType(com.mojang.brigadier.arguments.@org.checkerframework.checker.nullness.qual.NonNull ArgumentType<?>) // required {@link ArgumentMapping#argumentType() argumentType}
* .suggestionsType(org.incendo.cloud.brigadier.suggestion.@org.checkerframework.checker.nullness.qual.NonNull SuggestionsType) // optional {@link ArgumentMapping#suggestionsType() suggestionsType}
* .suggestionProvider(com.mojang.brigadier.suggestion.@org.checkerframework.checker.nullness.qual.Nullable SuggestionProvider<S> | null) // nullable {@link ArgumentMapping#suggestionProvider() suggestionProvider}
* .build();
*
* @param generic parameter S
* @return A new ImmutableArgumentMapping builder
*/
public static ImmutableArgumentMapping.Builder builder() {
return new ImmutableArgumentMapping.Builder<>();
}
/**
* Builds instances of type {@link ImmutableArgumentMapping ImmutableArgumentMapping}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ArgumentMapping", generator = "Immutables")
static final class Builder {
private static final long INIT_BIT_ARGUMENT_TYPE = 0x1L;
private long initBits = 0x1L;
private com.mojang.brigadier.arguments.@NonNull ArgumentType> argumentType;
private org.incendo.cloud.brigadier.suggestion.@NonNull SuggestionsType suggestionsType;
private com.mojang.brigadier.suggestion.@Nullable SuggestionProvider suggestionProvider;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ArgumentMapping} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ArgumentMapping instance) {
Objects.requireNonNull(instance, "instance");
argumentType(instance.argumentType());
suggestionsType(instance.suggestionsType());
com.mojang.brigadier.suggestion.@Nullable SuggestionProvider suggestionProviderValue = instance.suggestionProvider();
if (suggestionProviderValue != null) {
suggestionProvider(suggestionProviderValue);
}
return this;
}
/**
* Initializes the value for the {@link ArgumentMapping#argumentType() argumentType} attribute.
* @param argumentType The value for argumentType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder argumentType(com.mojang.brigadier.arguments.@NonNull ArgumentType> argumentType) {
this.argumentType = Objects.requireNonNull(argumentType, "argumentType");
initBits &= ~INIT_BIT_ARGUMENT_TYPE;
return this;
}
/**
* Initializes the value for the {@link ArgumentMapping#suggestionsType() suggestionsType} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link ArgumentMapping#suggestionsType() suggestionsType}.
* @param suggestionsType The value for suggestionsType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder suggestionsType(org.incendo.cloud.brigadier.suggestion.@NonNull SuggestionsType suggestionsType) {
this.suggestionsType = Objects.requireNonNull(suggestionsType, "suggestionsType");
return this;
}
/**
* Initializes the value for the {@link ArgumentMapping#suggestionProvider() suggestionProvider} attribute.
* @param suggestionProvider The value for suggestionProvider (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
public final Builder suggestionProvider(com.mojang.brigadier.suggestion.@Nullable SuggestionProvider suggestionProvider) {
this.suggestionProvider = suggestionProvider;
return this;
}
/**
* Builds a new {@link ImmutableArgumentMapping ImmutableArgumentMapping}.
* @return An immutable instance of ArgumentMapping
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableArgumentMapping build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableArgumentMapping(this);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ARGUMENT_TYPE) != 0) attributes.add("argumentType");
return "Cannot build ArgumentMapping, some of required attributes are not set " + attributes;
}
}
}