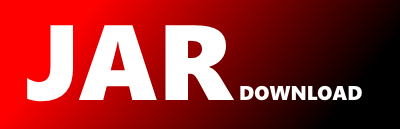
org.infinispan.api.async.AsyncCache Maven / Gradle / Ivy
package org.infinispan.api.async;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Flow;
import org.infinispan.api.common.CacheEntry;
import org.infinispan.api.common.CacheEntryVersion;
import org.infinispan.api.common.CacheOptions;
import org.infinispan.api.common.CacheWriteOptions;
import org.infinispan.api.common.events.cache.CacheEntryEvent;
import org.infinispan.api.common.events.cache.CacheEntryEventType;
import org.infinispan.api.common.events.cache.CacheListenerOptions;
import org.infinispan.api.common.process.CacheEntryProcessorResult;
import org.infinispan.api.common.process.CacheProcessor;
import org.infinispan.api.common.process.CacheProcessorOptions;
import org.infinispan.api.configuration.CacheConfiguration;
/**
* @since 14.0
**/
public interface AsyncCache {
/**
* The name of the cache.
*
* @return
*/
String name();
/**
* The configuration for this cache.
*
* @return
*/
CompletionStage configuration();
/**
* Return the container of this cache
*
* @return
*/
AsyncContainer container();
/**
* Get the value of the Key if such exists
*
* @param key
* @return the value
*/
default CompletionStage get(K key) {
return get(key, CacheOptions.DEFAULT);
}
/**
* Get the value of the Key if such exists
*
* @param key
* @param options
* @return the value
*/
default CompletionStage get(K key, CacheOptions options) {
return getEntry(key, options)
.thenApply(ce -> ce != null ? ce.value() : null);
}
/**
* Get the entry of the Key if such exists
*
* @param key
* @return the entry
*/
default CompletionStage> getEntry(K key) {
return getEntry(key, CacheOptions.DEFAULT);
}
/**
* Get the entry of the Key if such exists
*
* @param key
* @param options
* @return the entry
*/
CompletionStage> getEntry(K key, CacheOptions options);
/**
* Insert the key/value if such key does not exist
*
* @param key
* @param value
* @return the previous value if present
*/
default CompletionStage> putIfAbsent(K key, V value) {
return putIfAbsent(key, value, CacheWriteOptions.DEFAULT);
}
/**
* Insert the key/value if such key does not exist
*
* @param key
* @param value
* @param options
* @return the previous value if present
*/
CompletionStage> putIfAbsent(K key, V value, CacheWriteOptions options);
/**
* Insert the key/value if such key does not exist
*
* @param key
* @param value
* @return
*/
default CompletionStage setIfAbsent(K key, V value) {
return setIfAbsent(key, value, CacheWriteOptions.DEFAULT);
}
/**
* Insert the key/value if such key does not exist
*
* @param key
* @param value
* @param options
* @return Void
*/
default CompletionStage setIfAbsent(K key, V value, CacheWriteOptions options) {
return putIfAbsent(key, value, options)
.thenApply(Objects::isNull);
}
/**
* @param key
* @param value
* @return Void
*/
default CompletionStage> put(K key, V value) {
return put(key, value, CacheWriteOptions.DEFAULT);
}
/**
* @param key
* @param value
* @param options
* @return Void
*/
CompletionStage> put(K key, V value, CacheWriteOptions options);
/**
* @param key
* @param value
* @return
*/
default CompletionStage set(K key, V value) {
return set(key, value, CacheWriteOptions.DEFAULT);
}
/**
* @param key
* @param value
* @param options
* @return
*/
default CompletionStage set(K key, V value, CacheWriteOptions options) {
return put(key, value, options)
.thenApply(__ -> null);
}
/**
* @param key
* @param value
* @return
*/
default CompletionStage replace(K key, V value, CacheEntryVersion version) {
return replace(key, value, version, CacheWriteOptions.DEFAULT);
}
/**
* @param key
* @param value
* @param options
* @return
*/
default CompletionStage replace(K key, V value, CacheEntryVersion version, CacheWriteOptions options) {
return getOrReplaceEntry(key, value, version, options)
.thenApply(ce -> ce != null && version.equals(ce.metadata().version()));
}
/**
* @param key
* @param value
* @param version
* @return
*/
default CompletionStage> getOrReplaceEntry(K key, V value, CacheEntryVersion version) {
return getOrReplaceEntry(key, value, version, CacheWriteOptions.DEFAULT);
}
/**
* @param key
* @param value
* @param options
* @param version
* @return
*/
CompletionStage> getOrReplaceEntry(K key, V value, CacheEntryVersion version, CacheWriteOptions options);
/**
* Delete the key
*
* @param key
* @return whether the entry was removed.
*/
default CompletionStage remove(K key) {
return remove(key, CacheOptions.DEFAULT);
}
/**
* Delete the key
*
* @param key
* @param options
* @return whether the entry was removed.
*/
CompletionStage remove(K key, CacheOptions options);
/**
* Delete the key only if the version matches
*
* @param key
* @param version
* @return whether the entry was removed.
*/
default CompletionStage remove(K key, CacheEntryVersion version) {
return remove(key, version, CacheOptions.DEFAULT);
}
/**
* Delete the key only if the version matches
*
* @param key
* @param version
* @param options
* @return whether the entry was removed.
*/
CompletionStage remove(K key, CacheEntryVersion version, CacheOptions options);
/**
* Removes the key and returns its value if present.
*
* @param key
* @return the value of the key before removal. Returns null if the key didn't exist.
*/
default CompletionStage> getAndRemove(K key) {
return getAndRemove(key, CacheOptions.DEFAULT);
}
/**
* Removes the key and returns its value if present.
*
* @param key
* @param options
* @return the value of the key before removal. Returns null if the key didn't exist.
*/
CompletionStage> getAndRemove(K key, CacheOptions options);
/**
* Retrieve all keys
*
* @return
*/
default Flow.Publisher keys() {
return keys(CacheOptions.DEFAULT);
}
/**
* Retrieve all keys
*
* @param options
* @return
*/
Flow.Publisher keys(CacheOptions options);
/**
* Retrieve all entries
*
* @return
*/
default Flow.Publisher> entries() {
return entries(CacheOptions.DEFAULT);
}
/**
* Retrieve all entries
*
* @param options
* @return
*/
Flow.Publisher> entries(CacheOptions options);
/**
* @param entries
* @return Void
*/
default CompletionStage putAll(Map entries) {
return putAll(entries, CacheWriteOptions.DEFAULT);
}
/**
* @param entries
* @param options
* @return
*/
CompletionStage putAll(Map entries, CacheWriteOptions options);
/**
* @param entries
* @return Void
*/
default CompletionStage putAll(Flow.Publisher> entries) {
return putAll(entries, CacheWriteOptions.DEFAULT);
}
/**
* @param entries
* @param options
* @return
*/
CompletionStage putAll(Flow.Publisher> entries, CacheWriteOptions options);
/**
* Retrieves the entries for the specified keys.
*
* @param keys
* @return
*/
default Flow.Publisher> getAll(Set keys) {
return getAll(keys, CacheOptions.DEFAULT);
}
/**
* Retrieves the entries for the specified keys.
*
* @param keys
* @param options
* @return
*/
Flow.Publisher> getAll(Set keys, CacheOptions options);
/**
* Retrieves the entries for the specified keys.
*
* @param keys
* @return
*/
default Flow.Publisher> getAll(K... keys) {
return getAll(CacheOptions.DEFAULT, keys);
}
/**
* Retrieves the entries for the specified keys.
*
* @param keys
* @param options
* @return
*/
Flow.Publisher> getAll(CacheOptions options, K... keys);
/**
* Removes a set of keys. Returns the keys that were removed.
*
* @param keys
* @return
*/
default Flow.Publisher removeAll(Set keys) {
return removeAll(keys, CacheWriteOptions.DEFAULT);
}
/**
* Removes a set of keys. Returns the keys that were removed.
*
* @param keys
* @param options
* @return
*/
Flow.Publisher removeAll(Set keys, CacheWriteOptions options);
/**
* @param keys
* @return Void
*/
default Flow.Publisher removeAll(Flow.Publisher keys) {
return removeAll(keys, CacheWriteOptions.DEFAULT);
}
/**
* @param keys
* @param options
* @return
*/
Flow.Publisher removeAll(Flow.Publisher keys, CacheWriteOptions options);
/**
* Removes a set of keys. Returns the keys that were removed.
*
* @param keys
* @return
*/
default Flow.Publisher> getAndRemoveAll(Set keys) {
return getAndRemoveAll(keys, CacheWriteOptions.DEFAULT);
}
/**
* Removes a set of keys. Returns the keys that were removed.
*
* @param keys
* @param options
* @return
*/
Flow.Publisher> getAndRemoveAll(Set keys, CacheWriteOptions options);
/**
* Removes a set of keys. Returns the keys that were removed.
*
* @param keys
* @return
*/
default Flow.Publisher> getAndRemoveAll(Flow.Publisher keys) {
return getAndRemoveAll(keys, CacheWriteOptions.DEFAULT);
}
/**
* Removes a set of keys. Returns the keys that were removed.
*
* @param keys
* @param options
* @return
*/
Flow.Publisher> getAndRemoveAll(Flow.Publisher keys, CacheWriteOptions options);
/**
* Estimate the size of the store
*
* @return Long, estimated size
*/
default CompletionStage estimateSize() {
return estimateSize(CacheOptions.DEFAULT);
}
/**
* Estimate the size of the store
*
* @param options
* @return Long, estimated size
*/
CompletionStage estimateSize(CacheOptions options);
/**
* Clear the cache. If a concurrent operation puts data in the cache the clear might work properly
*
* @return Void
*/
default CompletionStage clear() {
return clear(CacheOptions.DEFAULT);
}
/**
* Clear the cache. If a concurrent operation puts data in the cache the clear might not properly work
*
* @param options
* @return Void
*/
CompletionStage clear(CacheOptions options);
/**
* @param
* @param query query String
* @return
*/
default AsyncQuery query(String query) {
return query(query, CacheOptions.DEFAULT);
}
/**
* Executes the query and returns an iterable with the entries
*
* @param
* @param query query String
* @param options
* @return
*/
AsyncQuery query(String query, CacheOptions options);
/**
* Register a cache listener with default {@link CacheListenerOptions}
*
* @param types
* @return
*/
default Flow.Publisher> listen(CacheEntryEventType... types) {
return listen(new CacheListenerOptions(), types);
}
/**
* Register a cache listener with the supplied {@link CacheListenerOptions}
*
* @param options
* @param types one or more {@link CacheEntryEventType}
* @return
*/
Flow.Publisher> listen(CacheListenerOptions options, CacheEntryEventType... types);
/**
* Process entries using the supplied task
*
* @param keys
* @param processor
* @return
*/
default Flow.Publisher> process(Set keys, AsyncCacheEntryProcessor processor) {
return process(keys, processor, CacheOptions.DEFAULT);
}
/**
* Process entries using the supplied task
*
* @param keys
* @param task
* @param options
* @return
*/
Flow.Publisher> process(Set keys, AsyncCacheEntryProcessor task, CacheOptions options);
/**
* Execute a {@link CacheProcessor} on a cache
*
* @param
* @param processor
* @return
*/
default Flow.Publisher> processAll(AsyncCacheEntryProcessor processor) {
return processAll(processor, CacheProcessorOptions.DEFAULT);
}
/**
* Execute a {@link CacheProcessor} on a cache
*
* @param
* @param processor
* @param options
* @return
*/
Flow.Publisher> processAll(AsyncCacheEntryProcessor processor, CacheProcessorOptions options);
/**
* @return
*/
AsyncStreamingCache streaming();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy