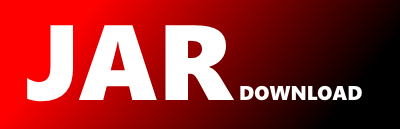
org.infinispan.commons.configuration.attributes.AttributeDefinition Maven / Gradle / Ivy
package org.infinispan.commons.configuration.attributes;
import org.infinispan.commons.CacheConfigurationException;
/**
*
* AttributeDefinition. Defines the characteristics of a configuration attribute. It is used to
* construct an actual {@link Attribute} holder.
*
* An attribute definition has the following characteristics:
*
* - A name
* - A default value or a value initializer
* - A type, which needs to be specified if it cannot be inferred from the default value, i.e.
* when it is null
* - Whether an attribute is immutable or not, i.e. whether its value is constant after
* initialization or it can be changed
* - A validator which intercepts invalid values
*
*
* @author Tristan Tarrant
* @since 7.2
*/
public final class AttributeDefinition {
private final String name;
private final T defaultValue;
private boolean immutable;
private final AttributeCopier copier;
private final AttributeInitializer extends T> initializer;
private final AttributeValidator super T> validator;
private final Class> type;
AttributeDefinition(String name, T initialValue, Class type, boolean immutable, AttributeCopier copier, AttributeValidator super T> validator, AttributeInitializer extends T> initializer) {
this.name = name;
this.defaultValue = initialValue;
this.immutable = immutable;
this.copier = copier;
this.initializer = initializer;
this.validator = validator;
this.type = type;
}
public String name() {
return name;
}
@SuppressWarnings("unchecked")
public Class getType() {
return (Class) type;
}
public T getDefaultValue() {
return initializer != null ? initializer().initialize() : defaultValue;
}
public boolean isImmutable() {
return immutable;
}
public AttributeCopier copier() {
return copier;
}
public AttributeInitializer extends T> initializer() {
return initializer;
}
AttributeValidator super T> validator() {
return validator;
}
public Attribute toAttribute() {
return new Attribute(this);
}
public void validate(T value) {
if (validator != null) {
validator.validate(value);
}
}
public static Builder builder(String name, T defaultValue) {
if (defaultValue != null) {
return new Builder(name, defaultValue, defaultValue.getClass());
} else {
throw new CacheConfigurationException("Must specify type when passing null for AttributeDefinition " + name);
}
}
public static Builder builder(String name, T defaultValue, Class klass) {
return new Builder<>(name, defaultValue, klass);
}
public static final class Builder {
private final String name;
private final T defaultValue;
private final Class> type;
private boolean immutable = false;
private AttributeCopier copier = null;
private AttributeInitializer extends T> initializer;
private AttributeValidator super T> validator;
private Builder(String name, T defaultValue, Class type) {
this.name = name;
this.defaultValue = defaultValue;
this.type = type;
}
public Builder immutable() {
this.immutable = true;
return this;
}
public Builder copier(AttributeCopier copier) {
this.copier = copier;
return this;
}
public Builder initializer(AttributeInitializer extends T> initializer) {
this.initializer = initializer;
return this;
}
public Builder validator(AttributeValidator super T> validator) {
this.validator = validator;
return this;
}
public AttributeDefinition build() {
return new AttributeDefinition(name, defaultValue, type, immutable, copier, validator, initializer);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy