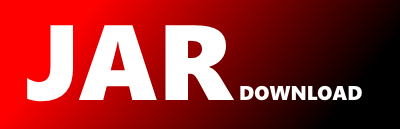
org.infinispan.client.hotrod.impl.operations.GetAllOperation Maven / Gradle / Ivy
The newest version!
package org.infinispan.client.hotrod.impl.operations;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import org.infinispan.client.hotrod.impl.ClientStatistics;
import org.infinispan.client.hotrod.impl.InternalRemoteCache;
import org.infinispan.client.hotrod.impl.protocol.Codec;
import org.infinispan.client.hotrod.impl.transport.netty.ByteBufUtil;
import org.infinispan.client.hotrod.impl.transport.netty.HeaderDecoder;
import io.netty.buffer.ByteBuf;
import io.netty.channel.Channel;
/**
* Implements "getAll" as defined by Hot Rod protocol specification.
*
* @author William Burns
* @since 7.2
*/
public class GetAllOperation extends AbstractCacheOperation
© 2015 - 2025 Weber Informatics LLC | Privacy Policy