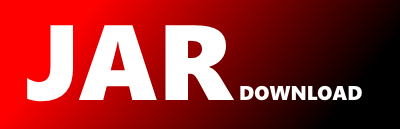
org.infinispan.client.hotrod.impl.InternalRemoteCache Maven / Gradle / Ivy
package org.infinispan.client.hotrod.impl;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Flow;
import javax.management.ObjectName;
import org.infinispan.api.async.AsyncCacheEntryProcessor;
import org.infinispan.api.common.CacheEntry;
import org.infinispan.api.common.CacheEntryVersion;
import org.infinispan.api.common.CacheOptions;
import org.infinispan.api.common.CacheWriteOptions;
import org.infinispan.api.common.events.cache.CacheEntryEvent;
import org.infinispan.api.common.events.cache.CacheEntryEventType;
import org.infinispan.api.common.events.cache.CacheListenerOptions;
import org.infinispan.api.common.process.CacheEntryProcessorResult;
import org.infinispan.api.common.process.CacheProcessorOptions;
import org.infinispan.api.configuration.CacheConfiguration;
import org.infinispan.client.hotrod.DataFormat;
import org.infinispan.client.hotrod.Flag;
import org.infinispan.client.hotrod.RemoteCache;
import org.infinispan.client.hotrod.VersionedValue;
import org.infinispan.client.hotrod.configuration.Configuration;
import org.infinispan.client.hotrod.event.impl.ClientListenerNotifier;
import org.infinispan.client.hotrod.impl.operations.CacheOperationsFactory;
import org.infinispan.client.hotrod.impl.operations.GetWithMetadataOperation;
import org.infinispan.client.hotrod.impl.operations.PingResponse;
import org.infinispan.client.hotrod.impl.transport.netty.OperationDispatcher;
import org.infinispan.commons.dataconversion.MediaType;
import org.infinispan.commons.util.CloseableIterator;
import org.infinispan.commons.util.IntSet;
import io.netty.channel.Channel;
public interface InternalRemoteCache extends RemoteCache {
byte[] getNameBytes();
CloseableIterator keyIterator(IntSet segments);
CloseableIterator> entryIterator(IntSet segments);
default boolean removeEntry(Map.Entry entry) {
return removeEntry(entry.getKey(), entry.getValue());
}
default boolean removeEntry(K key, V value) {
VersionedValue versionedValue = getWithMetadata(key);
return versionedValue != null && value.equals(versionedValue.getValue()) &&
removeWithVersion(key, versionedValue.getVersion());
}
CompletionStage> getWithMetadataAsync(K key, Channel channel);
@Override
InternalRemoteCache withFlags(Flag... flags);
@Override
InternalRemoteCache noFlags();
/**
* Similar to {@link #flags()} except it returns the flags as an int instead of a set of enums
* @return flags set as an int
*/
int flagInt();
@Override
InternalRemoteCache withDataFormat(DataFormat dataFormat);
boolean hasForceReturnFlag();
void resolveStorage();
default void resolveStorage(MediaType key, MediaType value) {
resolveStorage();
}
@Override
ClientStatistics clientStatistics();
void init(Configuration configuration, OperationDispatcher dispatcher);
void init(Configuration configuration, OperationDispatcher dispatcher, ObjectName jmxParent);
OperationDispatcher getDispatcher();
byte[] keyToBytes(Object o);
CompletionStage ping();
/**
* Add a client listener to handle near cache with bloom filter optimization
* The listener object must be annotated with @{@link org.infinispan.client.hotrod.annotation.ClientListener} annotation.
*/
Channel addNearCacheListener(Object listener, int bloomBits);
/**
* Sends the current bloom filter to the listener node where a near cache listener is installed. If this
* cache does not have near caching this will return an already completed stage.
* @return stage that when complete the filter was sent to the listener node
*/
CompletionStage updateBloomFilter();
CacheOperationsFactory getOperationsFactory();
ClientListenerNotifier getListenerNotifier();
CompletionStage configuration();
CompletionStage get(K key, CacheOptions options);
CompletionStage> getEntry(K key, CacheOptions options);
CompletionStage> putIfAbsent(K key, V value, CacheWriteOptions options);
CompletionStage setIfAbsent(K key, V value, CacheWriteOptions options);
CompletionStage> put(K key, V value, CacheWriteOptions options);
CompletionStage set(K key, V value, CacheWriteOptions options);
CompletionStage replace(K key, V value, CacheEntryVersion version, CacheWriteOptions options);
CompletionStage> getOrReplaceEntry(K key, V value, CacheEntryVersion version, CacheWriteOptions options);
CompletionStage remove(K key, CacheOptions options);
CompletionStage remove(K key, CacheEntryVersion version, CacheOptions options);
CompletionStage> getAndRemove(K key, CacheOptions options);
Flow.Publisher keys(CacheOptions options);
Flow.Publisher> entries(CacheOptions options);
CompletionStage putAll(Map entries, CacheWriteOptions options);
CompletionStage putAll(Flow.Publisher> entries, CacheWriteOptions options);
Flow.Publisher> getAll(Set keys, CacheOptions options);
Flow.Publisher> getAll(CacheOptions options, K[] keys);
Flow.Publisher removeAll(Set keys, CacheWriteOptions options);
Flow.Publisher removeAll(Flow.Publisher keys, CacheWriteOptions options);
Flow.Publisher> getAndRemoveAll(Set keys, CacheWriteOptions options);
Flow.Publisher> getAndRemoveAll(Flow.Publisher keys, CacheWriteOptions options);
CompletionStage estimateSize(CacheOptions options);
CompletionStage clear(CacheOptions options);
Flow.Publisher> listen(CacheListenerOptions options, CacheEntryEventType[] types);
Flow.Publisher> process(Set keys, AsyncCacheEntryProcessor task, CacheOptions options);
Flow.Publisher> processAll(AsyncCacheEntryProcessor processor, CacheProcessorOptions options);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy