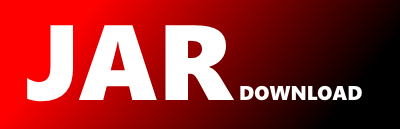
org.infinispan.lock.impl.functions.UnlockFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinispan-clustered-lock Show documentation
Show all versions of infinispan-clustered-lock Show documentation
Infinispan Clustered Lock module
package org.infinispan.lock.impl.functions;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.Collections;
import java.util.Set;
import java.util.function.Function;
import org.infinispan.commons.logging.LogFactory;
import org.infinispan.commons.marshall.AdvancedExternalizer;
import org.infinispan.commons.marshall.MarshallUtil;
import org.infinispan.functional.EntryView;
import org.infinispan.lock.impl.entries.ClusteredLockKey;
import org.infinispan.lock.impl.entries.ClusteredLockState;
import org.infinispan.lock.impl.entries.ClusteredLockValue;
import org.infinispan.lock.impl.externalizers.ExternalizerIds;
import org.infinispan.lock.logging.Log;
/**
* Function that allows to unlock the lock, if it's not already released.
*
*
*
* - If the requestor is not the owner, the lock won't be released.
* - If the requestId is null, this value does not affect the unlock
* - If the requestId is not null, the lock will be released only if the requestId and the owner match
* - If lock is already released, nothing happens
*
*
*
* @author Katia Aresti, [email protected]
* @since 9.2
*/
public class UnlockFunction implements Function, Boolean> {
private static final Log log = LogFactory.getLog(UnlockFunction.class, Log.class);
public static final AdvancedExternalizer EXTERNALIZER = new Externalizer();
private final String requestId;
private final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy