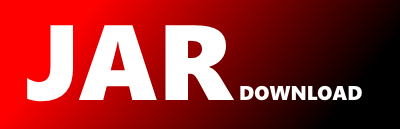
org.infinispan.commons.util.CloseableIteratorCollection Maven / Gradle / Ivy
package org.infinispan.commons.util;
import java.util.Collection;
import java.util.stream.Stream;
/**
* A collection that defines an iterator method that returns a {@link CloseableIterator}
* instead of a non closeable one. This is needed so that iterators can be properly cleaned up. All other
* methods will internally clean up any iterators created and don't have other side effects.
*
* @author wburns
* @since 7.0
*/
public interface CloseableIteratorCollection extends Collection {
/**
* {@inheritDoc}
*
* This iterator should be explicitly closed when iteration upon it is completed. Failure to do so could cause
* resources to not be freed properly
*/
@Override
CloseableIterator iterator();
/**
* {@inheritDoc}
*
* This spliterator should be explicitly closed after it has been used. Failure to do so could cause
* resources to not be freed properly
*/
@Override
CloseableSpliterator spliterator();
/**
* {@inheritDoc}
*
* This stream should be explicitly closed after it has been used. Failure to do so could cause
* resources to not be freed properly
*/
@Override
default Stream stream() {
return Collection.super.stream();
}
/**
* {@inheritDoc}
*
* This stream should be explicitly closed after it has been used. Failure to do so could cause
* resources to not be freed properly
*/
@Override
default Stream parallelStream() {
return Collection.super.parallelStream();
}
}