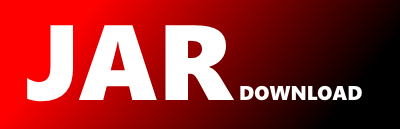
org.infinispan.container.DataContainer Maven / Gradle / Ivy
package org.infinispan.container;
import java.util.Iterator;
import java.util.Spliterator;
import java.util.Spliterators;
import org.infinispan.container.entries.InternalCacheEntry;
import org.infinispan.container.impl.InternalEntryFactory;
import org.infinispan.eviction.impl.PassivationManager;
import org.infinispan.factories.scopes.Scope;
import org.infinispan.factories.scopes.Scopes;
import org.infinispan.metadata.Metadata;
/**
* The main internal data structure which stores entries. Care should be taken when using this directly as entries
* could be stored in a different way than they were given to a {@link org.infinispan.Cache}. If you wish to convert
* entries to the stored format, you should use the provided {@link org.infinispan.encoding.DataConversion} such as
*
* cache.getAdvancedCache().getKeyDataConversion().toStorage(key);
*
* when dealing with keys or the following when dealing with values
*
* cache.getAdvancedCache().getValueDataConversion().toStorage(value);
*
* You can also convert from storage to the user provided type by using the
* {@link org.infinispan.encoding.DataConversion#fromStorage(Object)} method on any value returned from the DataContainer
* @author Manik Surtani ([email protected])
* @author Galder Zamarreño
* @author Vladimir Blagojevic
* @since 4.0
*/
@Scope(Scopes.NAMED_CACHE)
public interface DataContainer extends Iterable> {
/**
* Retrieves a cached entry
*
* @param k key under which entry is stored
* @return entry, if it exists and has not expired, or null if not
* @deprecated since 10.1 - Please use {@link #peek(Object)} instead.
*/
@Deprecated(forRemoval=true, since = "10.1")
InternalCacheEntry get(Object k);
/**
* Retrieves a cache entry in the same way as {@link #get(Object)}} except that it does not update or reorder any of
* the internal constructs. I.e., expiration does not happen, and in the case of the LRU container, the entry is not
* moved to the end of the chain.
*
* This method should be used instead of {@link #get(Object)}} when called while iterating through the data container
* using methods like {@link #iterator()} to avoid changing the underlying collection's order.
*
* @param k key under which entry is stored
* @return entry, if it exists, or null if not
*/
InternalCacheEntry peek(Object k);
/**
* Puts an entry in the cache along with metadata adding information such lifespan of entry, max idle time, version
* information...etc.
*
* @param k key under which to store entry
* @param v value to store
* @param metadata metadata of the entry
*/
void put(K k, V v, Metadata metadata);
/**
* Tests whether an entry exists in the container
*
* @param k key to test
* @return true if entry exists and has not expired; false otherwise
*/
boolean containsKey(Object k);
/**
* Removes an entry from the cache
*
* @param k key to remove
* @return entry removed, or null if it didn't exist or had expired
*/
InternalCacheEntry remove(Object k);
/**
* @return count of the number of entries in the container excluding expired entries
* @implSpec
* Default method invokes the {@link #iterator()} method and just counts entries.
*/
default int size() {
int size = 0;
// We have to loop through to make sure to remove expired entries
for (InternalCacheEntry ignore : this) {
if (++size == Integer.MAX_VALUE) return Integer.MAX_VALUE;
}
return size;
}
/**
*
* @return count of the number of entries in the container including expired entries
*/
int sizeIncludingExpired();
/**
* Removes all entries in the container
*/
void clear();
/**
* Atomically, it removes the key from {@code DataContainer} and passivates it to persistence.
*
* The passivation must be done by invoking the method {@link PassivationManager#passivateAsync(InternalCacheEntry)}.
*
* @param key The key to evict.
*/
void evict(K key);
/**
* Computes the new value for the key.
*
* See {@link org.infinispan.container.DataContainer.ComputeAction#compute(Object,
* org.infinispan.container.entries.InternalCacheEntry, InternalEntryFactory)}.
*
* Note the entry provided to {@link org.infinispan.container.DataContainer.ComputeAction} may be expired as these
* entries are not filtered as many other methods do.
* @param key The key.
* @param action The action that will compute the new value.
* @return The {@link org.infinispan.container.entries.InternalCacheEntry} associated to the key.
*/
InternalCacheEntry compute(K key, ComputeAction action);
/**
* {@inheritDoc}
* This iterator only returns entries that are not expired, however it will not remove them while doing so.
* @return iterator that doesn't produce expired entries
*/
@Override
Iterator> iterator();
/**
* {@inheritDoc}
* This spliterator only returns entries that are not expired, however it will not remove them while doing so.
* @return spliterator that doesn't produce expired entries
*/
@Override
default Spliterator> spliterator() {
return Spliterators.spliterator(iterator(), sizeIncludingExpired(),
Spliterator.CONCURRENT | Spliterator.NONNULL | Spliterator.DISTINCT);
}
/**
* Same as {@link DataContainer#iterator()} except that is also returns expired entries.
* @return iterator that returns all entries including expired ones
*/
Iterator> iteratorIncludingExpired();
/**
* Same as {@link DataContainer#spliterator()} except that is also returns expired entries.
* @return spliterator that returns all entries including expired ones
*/
default Spliterator> spliteratorIncludingExpired() {
return Spliterators.spliterator(iteratorIncludingExpired(), sizeIncludingExpired(),
Spliterator.CONCURRENT | Spliterator.NONNULL | Spliterator.DISTINCT);
}
interface ComputeAction {
/**
* Computes the new value for the key.
*
* @return The new {@code InternalCacheEntry} for the key, {@code null} if the entry is to be removed or {@code
* oldEntry} is the entry is not to be changed (i.e. not entries are added, removed or touched).
*/
InternalCacheEntry compute(K key, InternalCacheEntry oldEntry, InternalEntryFactory factory);
}
/**
* Resizes the capacity of the underlying container. This is only supported if the container is bounded.
* An {@link UnsupportedOperationException} is thrown otherwise.
*
* @param newSize the new size
*/
default void resize(long newSize) {
throw new UnsupportedOperationException();
}
/**
* Returns the capacity of the underlying container. This is only supported if the container is bounded. An {@link UnsupportedOperationException} is thrown
* otherwise.
*
* @return
*/
default long capacity() {
throw new UnsupportedOperationException();
}
/**
* Returns how large the eviction size is currently. This is only supported if the container is bounded. An
* {@link UnsupportedOperationException} is thrown otherwise. This value will always be lower than the value returned
* from {@link DataContainer#capacity()}
* @return how large the counted eviction is
*/
default long evictionSize() {
throw new UnsupportedOperationException();
}
}