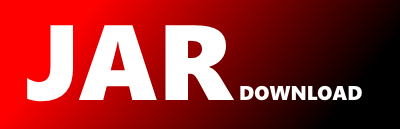
org.infinispan.reactive.RxJavaInterop Maven / Gradle / Ivy
package org.infinispan.reactive;
import java.lang.invoke.MethodHandles;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.function.BiFunction;
import org.infinispan.commons.util.concurrent.CompletionStages;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
import io.reactivex.rxjava3.core.Flowable;
import io.reactivex.rxjava3.processors.AsyncProcessor;
import io.reactivex.rxjava3.processors.UnicastProcessor;
/**
* Static factory class that provides methods to obtain commonly used instances for interoperation between RxJava
* and standard JRE.
* @author wburns
* @since 10.0
*/
public class RxJavaInterop extends org.infinispan.commons.reactive.RxJavaInterop {
private RxJavaInterop() { }
protected final static Log log = LogFactory.getLog(MethodHandles.lookup().lookupClass());
private static final BiFunction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy