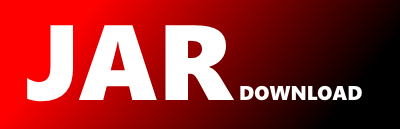
org.infinispan.statetransfer.StateChunk Maven / Gradle / Ivy
package org.infinispan.statetransfer;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.Collection;
import java.util.Collections;
import java.util.Set;
import org.infinispan.commons.marshall.AbstractExternalizer;
import org.infinispan.container.entries.InternalCacheEntry;
import org.infinispan.marshall.core.Ids;
/**
* Encapsulates a chunk of cache entries that belong to the same segment. This representation is suitable for sending it
* to another cache during state transfer.
*
* @author [email protected]
* @since 5.2
*/
public class StateChunk {
/**
* The id of the segment for which we push cache entries.
*/
private final int segmentId;
/**
* The cache entries. They are all guaranteed to be long to the same segment: segmentId.
*/
private final Collection> cacheEntries;
/**
* Indicates to receiver if there are more chunks to come for this segment.
*/
private final boolean isLastChunk;
public StateChunk(int segmentId, Collection> cacheEntries, boolean isLastChunk) {
this.segmentId = segmentId;
this.cacheEntries = cacheEntries;
this.isLastChunk = isLastChunk;
}
public int getSegmentId() {
return segmentId;
}
public Collection> getCacheEntries() {
return cacheEntries;
}
public boolean isLastChunk() {
return isLastChunk;
}
@Override
public String toString() {
return "StateChunk{" +
"segmentId=" + segmentId +
", cacheEntries=" + cacheEntries.size() +
", isLastChunk=" + isLastChunk +
'}';
}
public static class Externalizer extends AbstractExternalizer {
@Override
public Integer getId() {
return Ids.STATE_CHUNK;
}
@Override
public Set> getTypeClasses() {
return Collections.singleton(StateChunk.class);
}
@Override
public void writeObject(ObjectOutput output, StateChunk object) throws IOException {
output.writeInt(object.segmentId);
output.writeObject(object.cacheEntries);
output.writeBoolean(object.isLastChunk);
}
@Override
@SuppressWarnings("unchecked")
public StateChunk readObject(ObjectInput input) throws IOException, ClassNotFoundException {
int segmentId = input.readInt();
Collection> cacheEntries = (Collection>) input.readObject();
boolean isLastChunk = input.readBoolean();
return new StateChunk(segmentId, cacheEntries, isLastChunk);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy