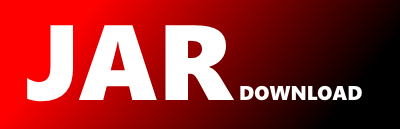
org.infinispan.statetransfer.TransactionInfo Maven / Gradle / Ivy
package org.infinispan.statetransfer;
import static org.infinispan.commons.marshall.MarshallUtil.marshallCollection;
import static org.infinispan.commons.marshall.MarshallUtil.unmarshallCollection;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.infinispan.commands.write.WriteCommand;
import org.infinispan.commons.marshall.AbstractExternalizer;
import org.infinispan.marshall.core.Ids;
import org.infinispan.transaction.xa.GlobalTransaction;
/**
* A representation of a transaction that is suitable for transferring between a StateProvider and a StateConsumer
* running on different members of the same cache.
*
* @author [email protected]
* @since 5.2
*/
public class TransactionInfo {
private final GlobalTransaction globalTransaction;
private final List modifications;
private final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy