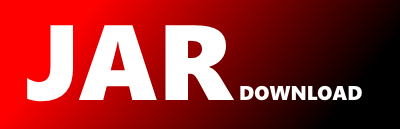
org.infinispan.stream.StreamMarshalling Maven / Gradle / Ivy
package org.infinispan.stream;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.function.Function;
import java.util.function.Predicate;
import org.infinispan.AdvancedCache;
import org.infinispan.Cache;
import org.infinispan.commons.marshall.AdvancedExternalizer;
import org.infinispan.commons.util.Util;
import org.infinispan.container.entries.CacheEntry;
import org.infinispan.factories.annotations.Inject;
import org.infinispan.factories.scopes.Scope;
import org.infinispan.factories.scopes.Scopes;
import org.infinispan.marshall.core.Ids;
/**
* Static factory class containing methods that will provide marshallable instances for very common use cases.
* Every instance returned from the various static methods uses the Infinispan marshalling to reduce payload sizes
* considerably and should be used whenever possible.
*/
public class StreamMarshalling {
private StreamMarshalling() { }
/**
* Provides a predicate that returns true when the object is equal.
* @param object the instance to test equality on
* @return the predicate
*/
public static Predicate
© 2015 - 2025 Weber Informatics LLC | Privacy Policy