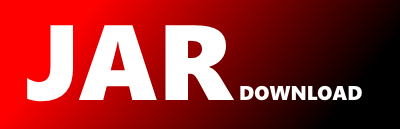
org.infinispan.topology.TopologyManagementHelper Maven / Gradle / Ivy
package org.infinispan.topology;
import static java.util.concurrent.TimeUnit.MILLISECONDS;
import static org.infinispan.util.logging.Log.CLUSTER;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.TimeUnit;
import org.infinispan.commands.GlobalRpcCommand;
import org.infinispan.commands.ReplicableCommand;
import org.infinispan.commands.topology.AbstractCacheControlCommand;
import org.infinispan.commons.util.Util;
import org.infinispan.factories.GlobalComponentRegistry;
import org.infinispan.factories.impl.BasicComponentRegistry;
import org.infinispan.remoting.inboundhandler.DeliverOrder;
import org.infinispan.remoting.responses.ExceptionResponse;
import org.infinispan.remoting.responses.Response;
import org.infinispan.remoting.responses.SuccessfulResponse;
import org.infinispan.remoting.transport.Address;
import org.infinispan.remoting.transport.ResponseCollector;
import org.infinispan.remoting.transport.Transport;
import org.infinispan.remoting.transport.impl.SingleResponseCollector;
import org.infinispan.commons.util.concurrent.CompletableFutures;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
public class TopologyManagementHelper {
private static final Log log = LogFactory.getLog(TopologyManagementHelper.class);
private final GlobalComponentRegistry gcr;
private final BasicComponentRegistry bcr;
public TopologyManagementHelper(GlobalComponentRegistry gcr) {
this.gcr = gcr;
this.bcr = gcr.getComponent(BasicComponentRegistry.class);
}
public CompletionStage executeOnClusterSync(Transport transport, ReplicableCommand command,
int timeout, ResponseCollector responseCollector) {
// First invoke the command remotely, but make sure we don't call finish() on the collector
ResponseCollector delegatingCollector = new DelegatingResponseCollector<>(responseCollector);
CompletionStage remoteFuture =
transport.invokeCommandOnAll(command, delegatingCollector, DeliverOrder.NONE, timeout, MILLISECONDS);
// Then invoke the command on the local node
CompletionStage> localFuture;
try {
if (log.isTraceEnabled())
log.tracef("Attempting to execute command on self: %s", command);
bcr.wireDependencies(command, true);
localFuture = invokeAsync(command);
} catch (Throwable throwable) {
localFuture = CompletableFuture.failedFuture(throwable);
}
return addLocalResult(responseCollector, remoteFuture, localFuture, transport.getAddress());
}
public void executeOnClusterAsync(Transport transport, ReplicableCommand command) {
// invoke remotely
try {
DeliverOrder deliverOrder = DeliverOrder.NONE;
transport.sendToAll(command, deliverOrder);
} catch (Exception e) {
throw Util.rewrapAsCacheException(e);
}
// invoke the command on the local node
try {
if (log.isTraceEnabled())
log.tracef("Attempting to execute command on self: %s", command);
bcr.wireDependencies(command, true);
invokeAsync(command);
} catch (Throwable throwable) {
// The command already logs any exception in invoke()
}
}
public CompletionStage
© 2015 - 2025 Weber Informatics LLC | Privacy Policy