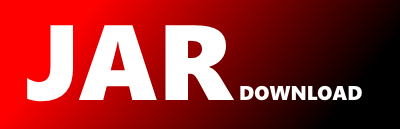
org.infinispan.util.WriteableCacheCollectionMapper Maven / Gradle / Ivy
package org.infinispan.util;
import java.util.Collection;
import java.util.function.Function;
import java.util.function.Predicate;
import org.infinispan.CacheCollection;
import org.infinispan.CacheStream;
import org.infinispan.commons.util.CloseableIterator;
import org.infinispan.commons.util.CloseableSpliterator;
import org.infinispan.commons.util.InjectiveFunction;
import org.infinispan.commons.util.IteratorMapper;
import org.infinispan.commons.util.SpliteratorMapper;
/**
* A writeable cache collection mapper that also has constant time operations for things such as
* {@link Collection#contains(Object)} if the underlying Collection does.
*
* This collection should be used for cases when a simple transformation of a element to another is all that is
* needed by the underlying collection.
*
* Note this class allows for a different function specifically for values returned from an iterator. This
* can be useful to intercept calls such as {@link java.util.Map.Entry#setValue(Object)} and update appropriately.
* @author wburns
* @since 9.2
* @param the original collection type - referred to as old in some methods
* @param the resulting collection type - referred to as new in some methods
*/
public class WriteableCacheCollectionMapper extends CollectionMapper implements CacheCollection {
protected final CacheCollection realCacheCollection;
protected final Function super E, ? extends R> toNewTypeIteratorFunction;
protected final Function super R, ? extends E> fromNewTypeFunction;
protected final InjectiveFunction