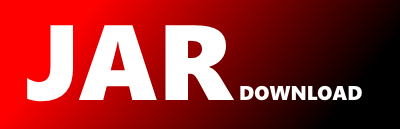
org.infinispan.marshall.AbstractDelegatingMarshaller Maven / Gradle / Ivy
/*
* Copyright 2012 Red Hat, Inc. and/or its affiliates.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301 USA
*/
package org.infinispan.marshall;
import org.infinispan.io.ByteBuffer;
import java.io.IOException;
import java.io.InputStream;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.io.OutputStream;
/**
* With the introduction of global and cache marshallers, there's a need to
* separate marshallers but still rely on the same marshalling backend as
* previously. So, this class acts as a delegator for the new marshallers.
*
* @author Galder Zamarreño
* @since 5.0
*/
public abstract class AbstractDelegatingMarshaller implements StreamingMarshaller {
protected StreamingMarshaller marshaller;
@Override
public void stop() {
marshaller.stop();
}
@Override
public ObjectOutput startObjectOutput(OutputStream os, boolean isReentrant, final int estimatedSize) throws IOException {
return marshaller.startObjectOutput(os, isReentrant, estimatedSize);
}
@Override @Deprecated
public ObjectOutput startObjectOutput(OutputStream os, boolean isReentrant) throws IOException {
return marshaller.startObjectOutput(os, isReentrant);
}
@Override
public void finishObjectOutput(ObjectOutput oo) {
marshaller.finishObjectOutput(oo);
}
@Override
public void objectToObjectStream(Object obj, ObjectOutput out) throws IOException {
marshaller.objectToObjectStream(obj, out);
}
@Override
public ObjectInput startObjectInput(InputStream is, boolean isReentrant) throws IOException {
return marshaller.startObjectInput(is, isReentrant);
}
@Override
public void finishObjectInput(ObjectInput oi) {
marshaller.finishObjectInput(oi);
}
@Override
public Object objectFromObjectStream(ObjectInput in) throws IOException, ClassNotFoundException, InterruptedException {
return marshaller.objectFromObjectStream(in);
}
@Override
public Object objectFromInputStream(InputStream is) throws IOException, ClassNotFoundException {
return marshaller.objectFromInputStream(is);
}
@Override
public byte[] objectToByteBuffer(Object obj, int estimatedSize) throws IOException, InterruptedException {
return marshaller.objectToByteBuffer(obj, estimatedSize);
}
@Override
public byte[] objectToByteBuffer(Object obj) throws IOException, InterruptedException {
return marshaller.objectToByteBuffer(obj);
}
@Override
public Object objectFromByteBuffer(byte[] buf) throws IOException, ClassNotFoundException {
return marshaller.objectFromByteBuffer(buf);
}
@Override
public Object objectFromByteBuffer(byte[] buf, int offset, int length) throws IOException, ClassNotFoundException {
return marshaller.objectFromByteBuffer(buf, offset, length);
}
@Override
public ByteBuffer objectToBuffer(Object o) throws IOException, InterruptedException {
return marshaller.objectToBuffer(o);
}
@Override
public boolean isMarshallable(Object o) throws Exception {
return marshaller.isMarshallable(o);
}
@Override
public BufferSizePredictor getBufferSizePredictor(Object o) {
return marshaller.getBufferSizePredictor(o);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy