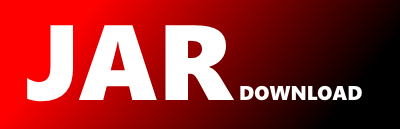
org.infinispan.statetransfer.OutboundTransferTask Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source
* Copyright 2012 Red Hat Inc. and/or its affiliates and other
* contributors as indicated by the @author tags. All rights reserved.
* See the copyright.txt in the distribution for a full listing of
* individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.infinispan.statetransfer;
import org.infinispan.commands.CommandsFactory;
import org.infinispan.container.DataContainer;
import org.infinispan.container.entries.InternalCacheEntry;
import org.infinispan.distribution.ch.ConsistentHash;
import org.infinispan.loaders.CacheLoaderException;
import org.infinispan.loaders.CacheLoaderManager;
import org.infinispan.loaders.CacheStore;
import org.infinispan.remoting.rpc.ResponseMode;
import org.infinispan.remoting.rpc.RpcManager;
import org.infinispan.remoting.transport.Address;
import org.infinispan.remoting.transport.jgroups.SuspectException;
import org.infinispan.util.InfinispanCollections;
import org.infinispan.util.ReadOnlyDataContainerBackedKeySet;
import org.infinispan.util.concurrent.ConcurrentMapFactory;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
import java.util.*;
import java.util.concurrent.CopyOnWriteArraySet;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.FutureTask;
/**
* Outbound state transfer task. Pushes data segments to another cluster member on request. Instances of
* OutboundTransferTask are created and managed by StateTransferManagerImpl. There should be at most
* one such task per destination at any time.
*
* @author [email protected]
* @since 5.2
*/
public class OutboundTransferTask implements Runnable {
private static final Log log = LogFactory.getLog(OutboundTransferTask.class);
private final boolean trace = log.isTraceEnabled();
private final StateProviderImpl stateProvider;
private final int topologyId;
private final Address destination;
private final Set segments = new CopyOnWriteArraySet();
private final int stateTransferChunkSize;
private final ConsistentHash readCh;
private final DataContainer dataContainer;
private final CacheLoaderManager cacheLoaderManager;
private final RpcManager rpcManager;
private final CommandsFactory commandsFactory;
private final long timeout;
private final String cacheName;
private final Map> entriesBySegment = ConcurrentMapFactory.makeConcurrentMap();
/**
* The total number of entries from all segments accumulated in entriesBySegment.
*/
private int accumulatedEntries;
/**
* The Future obtained from submitting this task to an executor service. This is used for cancellation.
*/
private FutureTask runnableFuture;
public OutboundTransferTask(Address destination, Set segments, int stateTransferChunkSize,
int topologyId, ConsistentHash readCh, StateProviderImpl stateProvider, DataContainer dataContainer,
CacheLoaderManager cacheLoaderManager, RpcManager rpcManager,
CommandsFactory commandsFactory, long timeout, String cacheName) {
if (segments == null || segments.isEmpty()) {
throw new IllegalArgumentException("Segments must not be null or empty");
}
if (destination == null) {
throw new IllegalArgumentException("Destination address cannot be null");
}
if (stateTransferChunkSize <= 0) {
throw new IllegalArgumentException("stateTransferChunkSize must be greater than 0");
}
this.stateProvider = stateProvider;
this.destination = destination;
this.segments.addAll(segments);
this.stateTransferChunkSize = stateTransferChunkSize;
this.topologyId = topologyId;
this.readCh = readCh;
this.dataContainer = dataContainer;
this.cacheLoaderManager = cacheLoaderManager;
this.rpcManager = rpcManager;
this.commandsFactory = commandsFactory;
this.timeout = timeout;
this.cacheName = cacheName;
}
public void execute(ExecutorService executorService) {
if (runnableFuture != null) {
throw new IllegalStateException("This task was already submitted");
}
runnableFuture = new FutureTask(this, null) {
@Override
protected void done() {
stateProvider.onTaskCompletion(OutboundTransferTask.this);
}
};
executorService.submit(runnableFuture);
}
public Address getDestination() {
return destination;
}
public Set getSegments() {
return segments;
}
//todo [anistor] check thread interrupt status in loops to implement faster cancellation
public void run() {
try {
// send data container entries
for (InternalCacheEntry ice : dataContainer) {
Object key = ice.getKey(); //todo [anistor] should we check for expired entries?
int segmentId = readCh.getSegment(key);
if (segments.contains(segmentId)) {
sendEntry(ice, segmentId);
}
}
// send cache store entries if needed
CacheStore cacheStore = getCacheStore();
if (cacheStore != null) {
try {
//todo [anistor] need to extend CacheStore interface to be able to specify a filter when loading keys (ie. keys should belong to desired segments)
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy