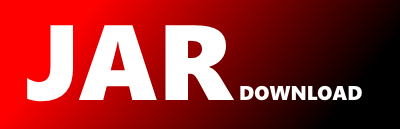
org.infinispan.util.ModuleProperties Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source
* Copyright 2009 Red Hat Inc. and/or its affiliates and other
* contributors as indicated by the @author tags. All rights reserved.
* See the copyright.txt in the distribution for a full listing of
* individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.infinispan.util;
import org.infinispan.commands.ReplicableCommand;
import org.infinispan.commands.module.ExtendedModuleCommandFactory;
import org.infinispan.commands.module.ModuleCommandExtensions;
import org.infinispan.commands.module.ModuleCommandFactory;
import org.infinispan.commands.module.ModuleCommandInitializer;
import org.infinispan.commands.remote.CacheRpcCommand;
import org.infinispan.factories.components.ModuleMetadataFileFinder;
import org.infinispan.lifecycle.ModuleLifecycle;
import org.infinispan.util.logging.Log;
import org.infinispan.util.logging.LogFactory;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.ServiceLoader;
/**
* The ModuleProperties
class represents Infinispan's module service extensions
*
* @author Vladimir Blagojevic
* @author Sanne Grinovero
* @author Galder Zamarreño
* @since 4.0
*/
public class ModuleProperties extends Properties {
private static final long serialVersionUID = 2558131508076199744L;
private static final Log log = LogFactory.getLog(ModuleProperties.class);
private Map commandFactories;
private Map commandInitializers;
private Collection> moduleCommands;
public static List resolveModuleLifecycles(ClassLoader cl) {
ServiceLoader moduleLifecycleLoader =
ServiceLoader.load(ModuleLifecycle.class, cl);
if (moduleLifecycleLoader.iterator().hasNext()) {
List lifecycles = new LinkedList();
for (ModuleLifecycle lifecycle : moduleLifecycleLoader) {
log.debugf("Loading lifecycle SPI class: %s", lifecycle);
lifecycles.add(lifecycle);
}
return lifecycles;
} else {
log.debugf("No module lifecycle SPI classes available");
return InfinispanCollections.emptyList();
}
}
/**
* Retrieves an Iterable containing metadata file finders declared by each module.
* @param cl class loader to use
* @return an Iterable of ModuleMetadataFileFinders
*/
public static Iterable getModuleMetadataFiles(ClassLoader cl) {
return ServiceLoader.load(ModuleMetadataFileFinder.class, cl);
}
@SuppressWarnings("unchecked")
public void loadModuleCommandHandlers(ClassLoader cl) {
ServiceLoader moduleCmdExtLoader =
ServiceLoader.load(ModuleCommandExtensions.class, cl);
if (moduleCmdExtLoader.iterator().hasNext()) {
commandFactories = new HashMap(1);
commandInitializers = new HashMap(1);
moduleCommands = new HashSet>(1);
for (ModuleCommandExtensions extension : moduleCmdExtLoader) {
log.debugf("Loading module command extension SPI class: %s", extension);
ExtendedModuleCommandFactory cmdFactory = extension.getModuleCommandFactory();
ModuleCommandInitializer cmdInitializer = extension.getModuleCommandInitializer();
for (Map.Entry> command :
cmdFactory.getModuleCommands().entrySet()) {
byte id = command.getKey();
if (commandFactories.containsKey(id))
throw new IllegalArgumentException(String.format(
"Cannot use id %d for commands, as it is already in use by %s",
id, commandFactories.get(id).getClass().getName()));
commandFactories.put(id, cmdFactory);
moduleCommands.add(command.getValue());
commandInitializers.put(id, cmdInitializer);
}
}
} else {
log.debugf("No module command extensions to load");
commandInitializers = InfinispanCollections.emptyMap();
commandFactories = InfinispanCollections.emptyMap();
}
}
public Collection> moduleCommands() {
return moduleCommands;
}
public Map moduleCommandFactories() {
return commandFactories;
}
public Map moduleCommandInitializers() {
return commandInitializers;
}
@SuppressWarnings("unchecked")
public Collection> moduleCacheRpcCommands() {
Collection> cmds = moduleCommands();
if (cmds == null || cmds.isEmpty())
return InfinispanCollections.emptySet();
Collection> cacheRpcCmds = new HashSet>(2);
for (Class extends ReplicableCommand> moduleCmdClass : cmds) {
if (CacheRpcCommand.class.isAssignableFrom(moduleCmdClass))
cacheRpcCmds.add((Class extends CacheRpcCommand>) moduleCmdClass);
}
return cacheRpcCmds;
}
public Collection> moduleOnlyReplicableCommands() {
Collection> cmds = moduleCommands();
if (cmds == null || cmds.isEmpty())
return InfinispanCollections.emptySet();
Collection> replicableOnlyCmds =
new HashSet>(2);
for (Class extends ReplicableCommand> moduleCmdClass : cmds) {
if (!CacheRpcCommand.class.isAssignableFrom(moduleCmdClass)) {
replicableOnlyCmds.add(moduleCmdClass);
}
}
return replicableOnlyCmds;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy