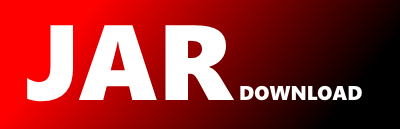
org.infinispan.atomic.AtomicMapLookup Maven / Gradle / Ivy
package org.infinispan.atomic;
import static org.infinispan.commons.util.Immutables.immutableMapWrap;
import java.util.Collections;
import java.util.Map;
import org.infinispan.Cache;
import org.infinispan.atomic.impl.AtomicMapProxyImpl;
import org.infinispan.atomic.impl.FineGrainedAtomicMapProxyImpl;
/**
* A helper that locates or safely constructs and registers atomic maps with a given cache. This should be the
* only way AtomicMaps are created/retrieved, to prevent concurrent creation, registration and possibly
* overwriting of such a map within the cache.
*
* @author Manik Surtani
* @see AtomicMap
* @since 4.0
*/
public class AtomicMapLookup {
/**
* Retrieves an atomic map from a given cache, stored under a given key. If an atomic map did not exist, one is
* created and registered in an atomic fashion.
*
* @param cache underlying cache
* @param key key under which the atomic map exists
* @param key param of the cache
* @param key param of the AtomicMap
* @param value param of the AtomicMap
* @return an AtomicMap
*/
public static AtomicMap getAtomicMap(Cache cache, MK key) {
return getAtomicMap(cache, key, true);
}
/**
* Retrieves a fine grained atomic map from a given cache, stored under a given key. If a fine grained atomic map did
* not exist, one is created and registered in an atomic fashion.
*
* @param cache underlying cache
* @param key key under which the atomic map exists
* @param key param of the cache
* @param key param of the AtomicMap
* @param value param of the AtomicMap
* @return an AtomicMap
*/
public static FineGrainedAtomicMap getFineGrainedAtomicMap(Cache cache, MK key) {
return getFineGrainedAtomicMap(cache, key, true);
}
/**
* Retrieves an atomic map from a given cache, stored under a given key.
*
* @param cache underlying cache
* @param key key under which the atomic map exists
* @param createIfAbsent if true, a new atomic map is created if one doesn't exist; otherwise null is returned if the
* map didn't exist.
* @param key param of the cache
* @param key param of the AtomicMap
* @param value param of the AtomicMap
* @return an AtomicMap, or null if one did not exist.
*/
public static AtomicMap getAtomicMap(Cache cache, MK key, boolean createIfAbsent) {
return (AtomicMap) getMap((Cache) cache, key, createIfAbsent, false);
}
/**
* Retrieves an atomic map from a given cache, stored under a given key.
*
* @param cache underlying cache
* @param key key under which the atomic map exists
* @param createIfAbsent if true, a new atomic map is created if one doesn't exist; otherwise null is returned if the
* map didn't exist.
* @param key param of the cache
* @param key param of the AtomicMap
* @param value param of the AtomicMap
* @return an AtomicMap, or null if one did not exist.
*/
public static FineGrainedAtomicMap getFineGrainedAtomicMap(Cache cache, MK key, boolean createIfAbsent) {
return (FineGrainedAtomicMap) getMap((Cache) cache, key, createIfAbsent, true);
}
/**
* Retrieves an atomic map from a given cache, stored under a given key.
*
*
* @param cache underlying cache
* @param key key under which the atomic map exists
* @param createIfAbsent if true, a new atomic map is created if one doesn't exist; otherwise null is returned if the
* map didn't exist.
* @param fineGrained if true, and createIfAbsent is true then created atomic map will be fine grained.
* @return an AtomicMap, or null if one did not exist.
*/
@SuppressWarnings("unchecked")
private static Map getMap(Cache cache, MK key, boolean createIfAbsent, boolean fineGrained) {
if (fineGrained) {
// With fine grained maps the cache will store both the keyset under master key and the entries under group keys
return FineGrainedAtomicMapProxyImpl.newInstance((Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy