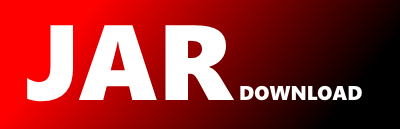
org.infinispan.util.ReadOnlyDataContainerBackedKeySet Maven / Gradle / Ivy
package org.infinispan.util;
import java.util.Collection;
import java.util.Iterator;
import java.util.Set;
import org.infinispan.container.DataContainer;
/**
* A Set view of keys in a data container, which is read-only and has efficient contains(), unlike some data container
* ley sets.
*
* @author Manik Surtani
* @since 4.1
* @deprecated DataContainer keySet method will be removed in the future. See {@link DataContainer#keySet()}
*/
@Deprecated
public class ReadOnlyDataContainerBackedKeySet implements Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy