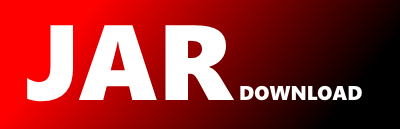
org.infinispan.commons.util.InfinispanCollections Maven / Gradle / Ivy
package org.infinispan.commons.util;
import org.infinispan.commons.marshall.AbstractExternalizer;
import org.infinispan.commons.marshall.Ids;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.*;
import static java.util.Collections.singletonMap;
import static java.util.Collections.unmodifiableMap;
/**
* Static helpers for Infinispan-specific collections
*
* @author Manik Surtani
* @since 4.0
*/
public class InfinispanCollections {
private static final Set EMPTY_SET = new EmptySet();
private static final Map EMPTY_MAP = new EmptyMap();
private static final List EMPTY_LIST = new EmptyList();
public static final class EmptySet extends AbstractSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy